USE C++ OR JAVA --- POST SOURCE CODE WILL ONLY UPVOTE IF CORRECT NO TIME CONSTRAINT --------------------------------------------------------------------------------------------------- Create a simple program that plays poker 1000 times with the following rules: Each time the program will: Shuffle the 52-card deck and deal yourself 5 cards. Using the remaining 47 cards, deal other 5 players their 5 cards. Determine if your hand would win or lose compared to the others. (Poker hands ranking listed below for reference) Your program will produce 2 output files (1000 entries): A session log output as a CSV (comma-separated value) file, with each hand (you and 5 other hands) on a separate line. For each hand: Output the cards in the hand; what the hand was evaluated as; and its winning percentage.
USE C++ OR JAVA --- POST SOURCE CODE
WILL ONLY UPVOTE IF CORRECT
NO TIME CONSTRAINT
---------------------------------------------------------------------------------------------------
Create a simple program that plays poker 1000 times with the following rules:
Each time the program will:
- Shuffle the 52-card deck and deal yourself 5 cards.
- Using the remaining 47 cards, deal other 5 players their 5 cards.
- Determine if your hand would win or lose compared to the others. (Poker hands ranking listed below for reference)
- Your program will produce 2 output files (1000 entries):
- A session log output as a CSV (comma-separated value) file, with each hand (you and 5 other hands) on a separate line. For each hand: Output the cards in the hand; what the hand was evaluated as; and its winning percentage.
- A summary showing the percentage of hands falling into each rank, and the overall win percentage for each rank, as a ‘normal’ text file.
---------------------------------------------------------------------------------------------------
The system random-number generator (random() and related functions) for the most common development environments will be more than adequate for this project.
Picture reference for card rankings:



public class Card
{
public final static int SPADES = 0; // Codes for the 4 suits, plus Joker.
public final static int HEARTS = 1;
public final static int DIAMONDS = 2;
public final static int CLUBS = 3;
public final static int JOKER = 4;
public final static int ACE = 1; // Codes for the non-numeric cards.
public final static int JACK = 11; // Cards 2 through 10 have their
public final static int QUEEN = 12; // numerical values for their codes.
public final static int KING = 13;
private final int suit;
private final int value;
public Card()
{
suit = JOKER;
value = 1;
}
public Card(int theValue, int theSuit)
{
if (theSuit != SPADES && theSuit != HEARTS && theSuit != DIAMONDS &&
theSuit != CLUBS && theSuit != JOKER)
throw new IllegalArgumentException("Illegal playing card suit");
if (theSuit != JOKER && (theValue < 1 || theValue > 13))
throw new IllegalArgumentException("Illegal playing card value");
value = theValue;
suit = theSuit;
}
public int getSuit()
{
return suit;
}
public int getValue()
{
return value;
}
public String getSuitAsString()
{
switch ( suit )
{
case SPADES: return "Spades";
case HEARTS: return "Hearts";
case DIAMONDS: return "Diamonds";
case CLUBS: return "Clubs";
default: return "Joker";
}
}
Step by step
Solved in 4 steps

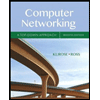
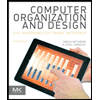
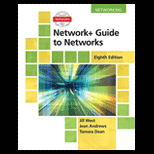
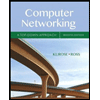
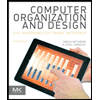
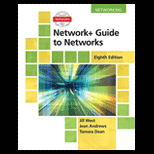
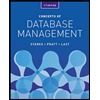
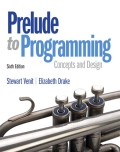
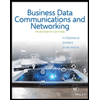