This is the question I am stuck on - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons. Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return saleAmount; } public int getInvoiceNumber() { return invoiceNumber; } public void display() { System.out.println("Invoice #" + invoiceNumber + " Amount of sale: $" + saleAmount + " Tax: $" + tax); } } -------------------------------------------------------------------------- import java.util.Scanner; public class SortPurchasesArray { public static void main(String[] args) { // Write code here } public static void sortBySaleAmount(Purchase[] array) { // Write code here } public static void sortByInvoice(Purchase[] array) { // Write code here } public static void display(Purchase[] p, String msg) { // Write code here } }
This is the question I am stuck on - In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons. Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order. This is the code I have - public class Purchase { private int invoiceNumber; private double saleAmount; private double tax; private static final double RATE = 0.05; public void setInvoiceNumber(int num) { invoiceNumber = num; } public void setSaleAmount(double amt) { saleAmount = amt; tax = saleAmount * RATE; } public double getSaleAmount() { return saleAmount; } public int getInvoiceNumber() { return invoiceNumber; } public void display() { System.out.println("Invoice #" + invoiceNumber + " Amount of sale: $" + saleAmount + " Tax: $" + tax); } } -------------------------------------------------------------------------- import java.util.Scanner; public class SortPurchasesArray { public static void main(String[] args) { // Write code here } public static void sortBySaleAmount(Purchase[] array) { // Write code here } public static void sortByInvoice(Purchase[] array) { // Write code here } public static void display(Purchase[] p, String msg) { // Write code here } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
This is the question I am stuck on -
In the exercises in Chapter 6, you created a class named Purchase. Each Purchase contains an invoice number, amount of sale, amount of sales tax, and several methods. Add get methods for the invoice number and sale amount fields so their values can be used in comparisons.
Next, write a program that declares an array of five Purchase objects and prompt a user for their values. Then, in a loop that continues until a user inputs a sentinel value of Z, ask the user whether the Purchase objects should be sorted and displayed in invoice number order or sale amount order.
This is the code I have -
public class Purchase
{
private int invoiceNumber;
private double saleAmount;
private double tax;
private static final double RATE = 0.05;
public void setInvoiceNumber(int num)
{
invoiceNumber = num;
}
public void setSaleAmount(double amt)
{
saleAmount = amt;
tax = saleAmount * RATE;
}
public double getSaleAmount()
{
return saleAmount;
}
public int getInvoiceNumber()
{
return invoiceNumber;
}
public void display()
{
System.out.println("Invoice #" + invoiceNumber +
" Amount of sale: $" + saleAmount + " Tax: $" + tax);
}
}
--------------------------------------------------------------------------
import java.util.Scanner;
public class SortPurchasesArray {
public static void main(String[] args) {
// Write code here
}
public static void sortBySaleAmount(Purchase[] array) {
// Write code here
}
public static void sortByInvoice(Purchase[] array) {
// Write code here
}
public static void display(Purchase[] p, String msg) {
// Write code here
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
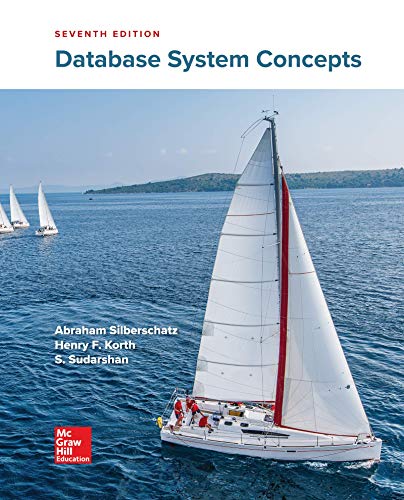
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
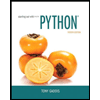
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
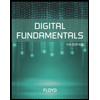
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
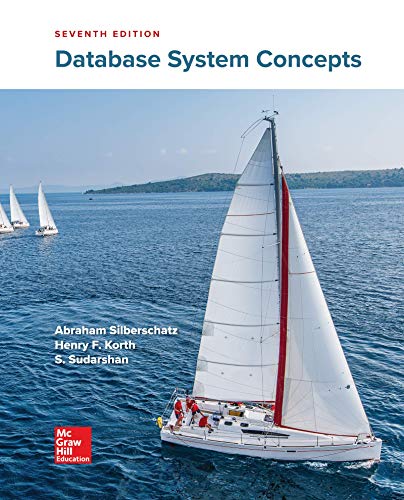
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
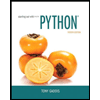
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
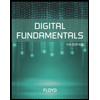
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
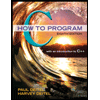
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
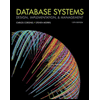
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
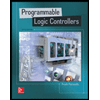
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education