This is the question I am struggling on - Create a TeeShirt class for Toby’s Tee Shirt Company. Fields include: orderNumber - of type int size - of type String color - of type String price - of type double Create set methods for the order number, size, and color and get methods for all four fields. The price is determined by the size: $22.99 for XXL or XXXL, and $19.99 for all other sizes. Create a subclass named CustomTee that descends from TeeShirt and includes a field named slogan (of type String) to hold the slogan requested for the shirt, and include get and set methods for this field. This is the code I have done but I am not getting the right output- public class CustomTee extends TeeShirt { private String slogan; public void setSlogan(String slgn) { // write your code here slogan = slgn; } public String getSlogan() { // write your code here return slogan; } } ----------------------------------------- import java.util.*; public class DemoTees { public static void main(String[] args) { TeeShirt tee1 = new TeeShirt(); TeeShirt tee2 = new TeeShirt(); CustomTee tee3 = new CustomTee();; CustomTee tee4 = new CustomTee(); tee1.setOrderNumber(100); tee1.setSize("XXL"); tee1.setColor("blue"); tee2.setOrderNumber(101); tee2.setSize("S"); tee2.setColor("gray"); tee3.setOrderNumber(102); tee3.setSize("L"); tee3.setColor("red"); tee3.setSlogan("Born to have fun"); tee4.setOrderNumber(104); tee4.setSize("XXXL"); tee4.setColor("black"); tee4.setSlogan("Wilson for Mayor"); display(tee1); display(tee2); displayCustomData(tee3); displayCustomData(tee4); } public static void display(TeeShirt tee) { System.out.println("Order #" + tee.getOrderNumber()); System.out.println(" Description: " + tee.getSize() + " " + tee.getColor()); System.out.println(" Price: $" + tee.getPrice()); } public static void displayCustomData(CustomTee tee) { display(tee); System.out.println(" Slogan: " + tee.getSlogan()); } } ------------------------------------- public class TeeShirt { private int orderNumber; private String size; private String color; private double price; public void setOrderNumber(int num) { // write your code here orderNumber = num; } public void setSize(String sz) { // write your code here size = sz; } public void setColor(String color) { // write your code here color = color; } public int getOrderNumber() { // write your code here return orderNumber; } public String getSize() { // write your code here return size; } public String getColor() { // write your code here return color; } public double getPrice() { // write your code here return price; } }
This is the question I am struggling on - Create a TeeShirt class for Toby’s Tee Shirt Company. Fields include: orderNumber - of type int size - of type String color - of type String price - of type double Create set methods for the order number, size, and color and get methods for all four fields. The price is determined by the size: $22.99 for XXL or XXXL, and $19.99 for all other sizes. Create a subclass named CustomTee that descends from TeeShirt and includes a field named slogan (of type String) to hold the slogan requested for the shirt, and include get and set methods for this field. This is the code I have done but I am not getting the right output- public class CustomTee extends TeeShirt { private String slogan; public void setSlogan(String slgn) { // write your code here slogan = slgn; } public String getSlogan() { // write your code here return slogan; } } ----------------------------------------- import java.util.*; public class DemoTees { public static void main(String[] args) { TeeShirt tee1 = new TeeShirt(); TeeShirt tee2 = new TeeShirt(); CustomTee tee3 = new CustomTee();; CustomTee tee4 = new CustomTee(); tee1.setOrderNumber(100); tee1.setSize("XXL"); tee1.setColor("blue"); tee2.setOrderNumber(101); tee2.setSize("S"); tee2.setColor("gray"); tee3.setOrderNumber(102); tee3.setSize("L"); tee3.setColor("red"); tee3.setSlogan("Born to have fun"); tee4.setOrderNumber(104); tee4.setSize("XXXL"); tee4.setColor("black"); tee4.setSlogan("Wilson for Mayor"); display(tee1); display(tee2); displayCustomData(tee3); displayCustomData(tee4); } public static void display(TeeShirt tee) { System.out.println("Order #" + tee.getOrderNumber()); System.out.println(" Description: " + tee.getSize() + " " + tee.getColor()); System.out.println(" Price: $" + tee.getPrice()); } public static void displayCustomData(CustomTee tee) { display(tee); System.out.println(" Slogan: " + tee.getSlogan()); } } ------------------------------------- public class TeeShirt { private int orderNumber; private String size; private String color; private double price; public void setOrderNumber(int num) { // write your code here orderNumber = num; } public void setSize(String sz) { // write your code here size = sz; } public void setColor(String color) { // write your code here color = color; } public int getOrderNumber() { // write your code here return orderNumber; } public String getSize() { // write your code here return size; } public String getColor() { // write your code here return color; } public double getPrice() { // write your code here return price; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
This is the question I am struggling on -
Create a TeeShirt class for Toby’s Tee Shirt Company. Fields include:
- orderNumber - of type int
- size - of type String
- color - of type String
- price - of type double
Create set methods for the order number, size, and color and get methods for all four fields. The price is determined by the size: $22.99 for XXL or XXXL, and $19.99 for all other sizes.
Create a subclass named CustomTee that descends from TeeShirt and includes a field named slogan (of type String) to hold the slogan requested for the shirt, and include get and set methods for this field.
This is the code I have done but I am not getting the right output-
public class CustomTee extends TeeShirt
{
private String slogan;
public void setSlogan(String slgn)
{
// write your code here
slogan = slgn;
}
public String getSlogan()
{
// write your code here
return slogan;
}
}
-----------------------------------------
import java.util.*;
public class DemoTees
{
public static void main(String[] args)
{
TeeShirt tee1 = new TeeShirt();
TeeShirt tee2 = new TeeShirt();
CustomTee tee3 = new CustomTee();;
CustomTee tee4 = new CustomTee();
tee1.setOrderNumber(100);
tee1.setSize("XXL");
tee1.setColor("blue");
tee2.setOrderNumber(101);
tee2.setSize("S");
tee2.setColor("gray");
tee3.setOrderNumber(102);
tee3.setSize("L");
tee3.setColor("red");
tee3.setSlogan("Born to have fun");
tee4.setOrderNumber(104);
tee4.setSize("XXXL");
tee4.setColor("black");
tee4.setSlogan("Wilson for Mayor");
display(tee1);
display(tee2);
displayCustomData(tee3);
displayCustomData(tee4);
}
public static void display(TeeShirt tee)
{
System.out.println("Order #" + tee.getOrderNumber());
System.out.println(" Description: " + tee.getSize() +
" " + tee.getColor());
System.out.println(" Price: $" + tee.getPrice());
}
public static void displayCustomData(CustomTee tee)
{
display(tee);
System.out.println(" Slogan: " + tee.getSlogan());
}
}
-------------------------------------public class TeeShirt
{
private int orderNumber;
private String size;
private String color;
private double price;
public void setOrderNumber(int num)
{
// write your code here
orderNumber = num;
}
public void setSize(String sz)
{
// write your code here
size = sz;
}
public void setColor(String color)
{
// write your code here
color = color;
}
public int getOrderNumber()
{
// write your code here
return orderNumber;
}
public String getSize()
{
// write your code here
return size;
}
public String getColor()
{
// write your code here
return color;
}
public double getPrice()
{
// write your code here
return price;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
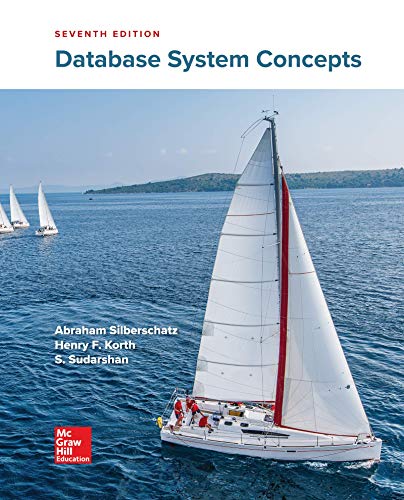
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
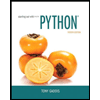
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
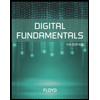
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
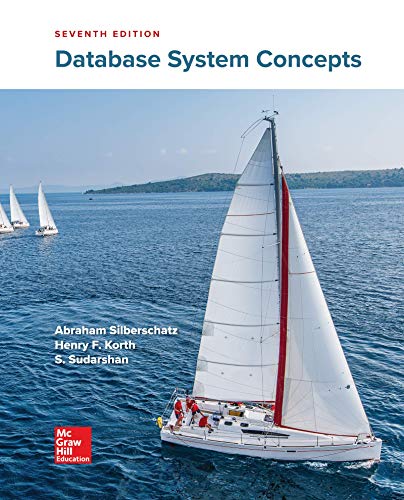
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
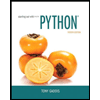
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
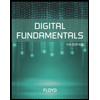
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
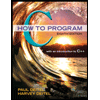
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
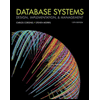
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
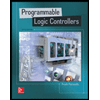
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education