This is Python ordering system, I'm really having a hard time to fix my problems: - looping if order_quantity > food_list[order_food].get_stock() - wrong information int displaying the receipt Also, if you have a better idea on how to implement my code. Please tell me, I want to improve. Stackoverflow is kinda harsh for me. Thanks a lot
This is Python ordering system, I'm really having a hard time to fix my problems:
- looping if order_quantity > food_list[order_food].get_stock()
- wrong information int displaying the receipt
Also, if you have a better idea on how to implement my code. Please tell me, I want to improve. Stackoverflow is kinda harsh for me. Thanks a lot.
CODE:
import random
#
# CLASS
#
class Food:
def __init__(self, food_name, food_price, food_stock):
self.__food_name = food_name
self.__food_price = food_price
self.__food_stock = food_stock
# SETTER METHODS
def set_name(self, product_name):
self.__food_name = product_name
def set_price(self, product_price):
self.__food_price = product_price
def set_stock(self, food_stock):
self.__food_stock = food_stock
# GETTER METHODS
def get_name(self):
return self.__food_name
def get_price(self):
return self.__food_price
def get_stock(self):
return self.__food_stock
#
# LIST
#
# Store name and price in a list
food_list = \
[
Food("Fishball", 5, random.randint(0, 0)),
Food("Kwek-kwek", 10, random.randint(0, 300)),
Food("Tempura", 5, random.randint(0, 300)),
Food("Lumpia", 10, random.randint(0, 300)),
Food("Isaw", 5, random.randint(0, 300)),
Food("Hotdog", 20, random.randint(0, 300)),
Food("Chicken skin", 5, random.randint(0, 300)),
Food("Squid skin", 5, random.randint(0, 300)),
Food("Siomai", 15, random.randint(0, 300))
]
#
# FUNCTIONS
#
def order():
order_quantity = 0
print("How many orders will you make? ", end = "")
total = int(input())
print("")
for i in range(total):
# Get order from the user
order_food = int(input("What is your order? "))
while order_quantity < food_list[order_food].get_stock():
print("How many", food_list[order_food].get_name(), "do you want to order? ", end = "")
order_quantity = int(input())
if order_quantity > food_list[order_food].get_stock():
print("\n>> WE ONLY HAVE", food_list[order_food].get_stock(), "OF", food_list[order_food].get_name(), "\n")
print("\n>> ORDER", i + 1, "SUCCESSFULLY ADDED\n")
# Display ordered food
print("\n\n")
print("+ -- -- -- -- -- -- -- -- -- -- -- -- -- +")
print("| |")
print("| |")
print("| *** RECEIPT *** |")
print("| |")
print("| |")
for j in range(total):
order_total = food_list[order_food].get_price() * order_quantity
print("| \t", order_quantity, food_list[order_food].get_name(), ".......... ₱", order_total, " |")
print("| -------- |")
print("| |")
print("| THANK YOU FOR ORDERING!!! |")
print("| |")
print("+ -- -- -- -- -- -- -- -- -- -- -- -- -- +")
def food_menu():
# Display menu
print("Available today: ")
for i in range(len(food_list)):
print("\t[" + str(i + 1) + "] ---", food_list[i].get_name(), "(", food_list[i].get_stock(), "avail.)", ".......... ₱", str(food_list[i].get_price()))
print("\n==================")
# Call the order function to get the order/s of the user
order()
#
# MAIN
#
if __name__ == "__main__":
# Call the food_menu function to display the menu
food_menu()

Step by step
Solved in 2 steps with 3 images

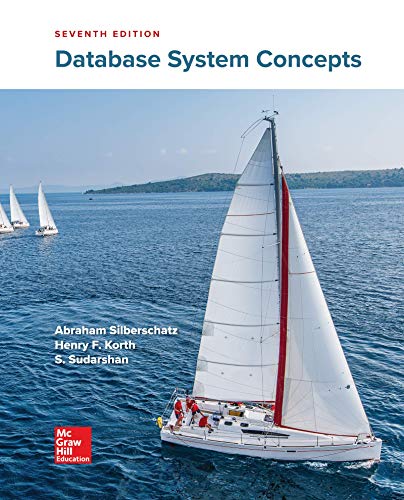
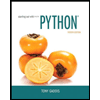
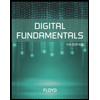
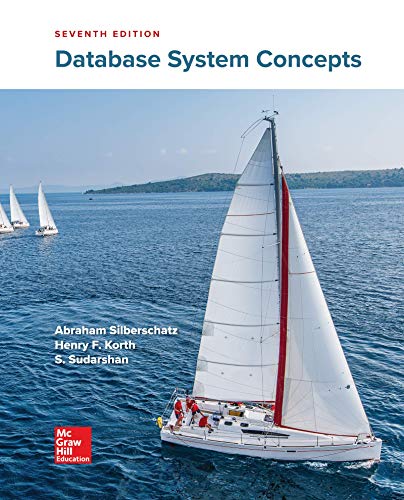
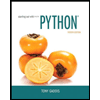
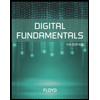
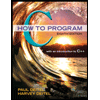
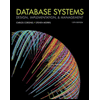
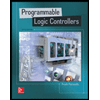