Given the user inputs, complete a program that does the following tasks: • Define a list, my_list, containing the user inputs: my_flower1, my_flower2, and my_flower3 in the same order. • Define a list, your_list, containing the user inputs, your_flower1 and your_flower2, in the same order. • Define a list, our_list, by concatenating my_list and your_list. Append the user input, their_flower, to the end of our_list. • Replace my_flower2 in our_list with their_flower. • Remove the first occurrence of their_flower from our_list without using index(). • Remove the second element of our_list. Observe the output of each print statement carefully to understand what was done by each task of the program. Ex: If the input is: rose peony lily rose daisy aster the output is: ['rose', 'peony', 'lily', 'rose', 'daisy'] ['rose', 'peony', 'lily', 'rose', 'daisy', 'aster'] ['rose', 'aster', 'lily', 'rose', 'daisy', 'aster'] ['rose', 'lily', 'rose', 'daisy', 'aster'] ['rose', 'rose', 'daisy', 'aster']
Given the user inputs, complete a program that does the following tasks: • Define a list, my_list, containing the user inputs: my_flower1, my_flower2, and my_flower3 in the same order. • Define a list, your_list, containing the user inputs, your_flower1 and your_flower2, in the same order. • Define a list, our_list, by concatenating my_list and your_list. Append the user input, their_flower, to the end of our_list. • Replace my_flower2 in our_list with their_flower. • Remove the first occurrence of their_flower from our_list without using index(). • Remove the second element of our_list. Observe the output of each print statement carefully to understand what was done by each task of the program. Ex: If the input is: rose peony lily rose daisy aster the output is: ['rose', 'peony', 'lily', 'rose', 'daisy'] ['rose', 'peony', 'lily', 'rose', 'daisy', 'aster'] ['rose', 'aster', 'lily', 'rose', 'daisy', 'aster'] ['rose', 'lily', 'rose', 'daisy', 'aster'] ['rose', 'rose', 'daisy', 'aster']
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hi I need help with this problem. The program I'm using is Python.
![3.18 LAB: List basics
Given the user inputs, complete a program that does the following tasks:
• Define a list, my_list, containing the user inputs: my_flower1, my_flower2, and my_flower3 in the same order.
Define a list, your_list, containing the user inputs, your_flower1 and your_flower2, in the same order.
Define a list, our_list, by concatenating my_list and your_list.
Append the user input, their_flower, to the end of our_list.
Replace my_flower2 in our_list with their_flower.
Remove the first occurrence of their_flower from our_list without using index().
• Remove the second element of our_list.
●
●
●
Observe the output of each print statement carefully to understand what was done by each task of the program.
Ex: If the input is:
rose
peony
lily
rose
daisy
aster
the output is:
['rose', 'peony', 'lily', 'rose', 'daisy']
['rose', 'peony', 'lily', 'rose', 'daisy', 'aster']
['rose', 'aster', 'lily', 'rose', 'daisy', 'aster']
['rose', 'lily', 'rose', 'daisy', 'aster']
['rose', 'rose', 'daisy', 'aster']](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F38ffcadc-da65-4b22-b38b-e3b0eee6db32%2F227d789a-3b05-4eb1-85f2-75ac66f2d927%2Fzvmbdks_processed.png&w=3840&q=75)
Transcribed Image Text:3.18 LAB: List basics
Given the user inputs, complete a program that does the following tasks:
• Define a list, my_list, containing the user inputs: my_flower1, my_flower2, and my_flower3 in the same order.
Define a list, your_list, containing the user inputs, your_flower1 and your_flower2, in the same order.
Define a list, our_list, by concatenating my_list and your_list.
Append the user input, their_flower, to the end of our_list.
Replace my_flower2 in our_list with their_flower.
Remove the first occurrence of their_flower from our_list without using index().
• Remove the second element of our_list.
●
●
●
Observe the output of each print statement carefully to understand what was done by each task of the program.
Ex: If the input is:
rose
peony
lily
rose
daisy
aster
the output is:
['rose', 'peony', 'lily', 'rose', 'daisy']
['rose', 'peony', 'lily', 'rose', 'daisy', 'aster']
['rose', 'aster', 'lily', 'rose', 'daisy', 'aster']
['rose', 'lily', 'rose', 'daisy', 'aster']
['rose', 'rose', 'daisy', 'aster']
![454192.2153518.qx3zqy7
LAB
ACTIVITY
3.18.1: LAB: List basics
1 my_flower1 = input()
2 my_flower2 = input()
3 my_flower3 = input()
4
5 your_flower1=input()
6 your_flower2 = input()
7
8 their_flower = input()
9
10 my_list=['my_flower1', 'my_flower2', 'my_flower3']
11
12 your list your_flower1, your_flower2
13
14 our_list = [my_list, your_list]
15
16
print (our_list)
17
18 their_flower.append (our_list)
Develop mode Submit mode
Enter program input (optional)
rose
peony
Run program
main.py
Input (from above)
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
second box.
main.py
0/10
Load default template...
Output (shown below)](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F38ffcadc-da65-4b22-b38b-e3b0eee6db32%2F227d789a-3b05-4eb1-85f2-75ac66f2d927%2F0p5qiy_processed.png&w=3840&q=75)
Transcribed Image Text:454192.2153518.qx3zqy7
LAB
ACTIVITY
3.18.1: LAB: List basics
1 my_flower1 = input()
2 my_flower2 = input()
3 my_flower3 = input()
4
5 your_flower1=input()
6 your_flower2 = input()
7
8 their_flower = input()
9
10 my_list=['my_flower1', 'my_flower2', 'my_flower3']
11
12 your list your_flower1, your_flower2
13
14 our_list = [my_list, your_list]
15
16
print (our_list)
17
18 their_flower.append (our_list)
Develop mode Submit mode
Enter program input (optional)
rose
peony
Run program
main.py
Input (from above)
Run your program as often as you'd like, before submitting for grading. Below, type any needed
input values in the first box, then click Run program and observe the program's output in the
second box.
main.py
0/10
Load default template...
Output (shown below)
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
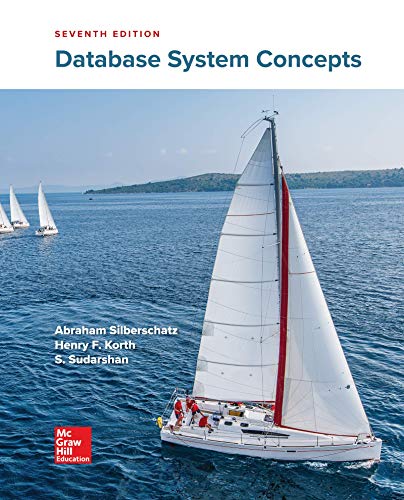
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
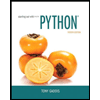
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
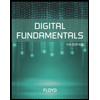
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
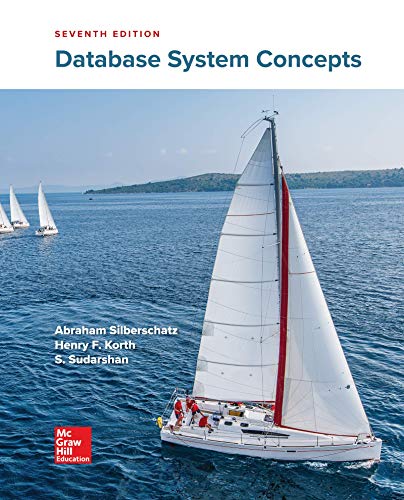
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
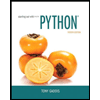
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
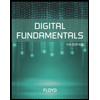
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
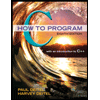
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
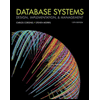
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
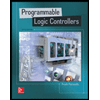
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education