When you compile and run packaged software from an IDE, the execution process can be as easy as clicking a run icon, as the IDE will maintain the classpath for you and will also let you know if anything is out of sorts. When you try to compile and interpret the code yourself from the command line, you will need to know exactly how to path your files. Let us start from c:\Code directory for this assignment. Consider a java file who's .class will result in the com.CITC1318.course package as follows: package com.CITC1318.course; public class GreetingsClass { public static void main(String[] args) { System.out.println("$ Greetings, CITC1318!"); } } This exercise will have you compiling and running the application with new classes created in a separate package: 1. Compile the program: c:\Code>javac -d . GreetingsClass.java 2. Run the program to ensure it is error-free: c:\Code>java -cp . com.CITC1318.course.GreetingsClass 3. Create three classes named Chapter1, Chapter2, and Chapter3 that are placed in the com.CITC1318.course.Chapters package. Create constructors that will print the names of the chapters to standard out. The details for the Chapter1 class are given here as an example of what you will need to do: package com.CITC1318.course.Chapters; public class Chapter1 { public Chapter1(){ System.out.println("Hello from Chapter1!"); } } 4. Instantiate each class from the main program, by adding the necessary code to the GreetingsClass class. Here is how to instantiate the object c1 from the class Chapter1: Chapter1 c1 = new Chapter1(); Make sure you do a c2 from the class Chapter2 and c3 from the class Chapter3. 5. Ensure that all of the class code are in the paths code\com\CITC1318\course and code\com\CITC1318\course\Chapters 6. Determine the command-line arguments needed to compile the complete program. Compile the complete program, and debug where necessary. Make sure to save all the commands and the details of your compilation in a text file that you need to upload to the Dropbox. 7. Determine the command-line arguments needed to execute the program. Make sure to update the text file in 6. Load all the files in the "code" directory as a zip file. Make sure your zip file not only contains the Code folder but also all the folders including code\com\CITC1318\course and code\com\CITC1318\course\Chapters and their files. Not uploading the zip file of "code" will cost you a 0. The standard output will read as follows: $ Greetings, CITC1318! Hello from Chapter1! Hello from Chapter2! Hello from Chapter3!
When you compile and run packaged software from an IDE, the execution process can be as easy as clicking a run icon, as the IDE will maintain the classpath for you and will also let you know if anything is out of sorts. When you try to compile and interpret the code yourself from the command line, you will need to know exactly how to path your files.
Let us start from c:\Code directory for this assignment. Consider a java file who's .class will result in the com.CITC1318.course package as follows:
package com.CITC1318.course;
public class GreetingsClass {
public static void main(String[] args) {
System.out.println("$ Greetings, CITC1318!");
} }
This exercise will have you compiling and running the application with new classes created in a separate package:
1. Compile the program: c:\Code>javac -d . GreetingsClass.java
2. Run the program to ensure it is error-free:
c:\Code>java -cp . com.CITC1318.course.GreetingsClass
3. Create three classes named Chapter1, Chapter2, and Chapter3 that are placed in the com.CITC1318.course.Chapters package. Create constructors that will print the names of the chapters to standard out. The details for the Chapter1 class are given here as an example of what you will need to do:
package com.CITC1318.course.Chapters;
public class Chapter1 {
public Chapter1(){
System.out.println("Hello from Chapter1!");
} }
4. Instantiate each class from the main program, by adding the necessary code to the GreetingsClass class. Here is how to instantiate the object c1 from the class Chapter1:
Chapter1 c1 = new Chapter1();
Make sure you do a c2 from the class Chapter2 and c3 from the class Chapter3.
5. Ensure that all of the class code are in the paths
code\com\CITC1318\course and code\com\CITC1318\course\Chapters
6. Determine the command-line arguments needed to compile the complete program. Compile the complete program, and debug where necessary. Make sure to save all the commands and the details of your compilation in a text file that you need to upload to the Dropbox.
7. Determine the command-line arguments needed to execute the program. Make sure to update the text file in 6. Load all the files in the "code" directory as a zip file. Make sure your zip file not only contains the Code folder but also all the folders including code\com\CITC1318\course and code\com\CITC1318\course\Chapters and their files. Not uploading the zip file of "code" will cost you a 0.
The standard output will read as follows:
$ Greetings, CITC1318!
Hello from Chapter1!
Hello from Chapter2!
Hello from Chapter3!

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

I have the code, I can not figure out how to compile it so that it will run. I keep getting errors
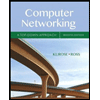
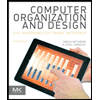
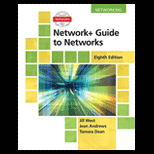
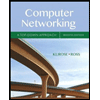
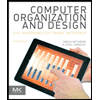
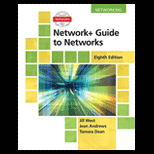
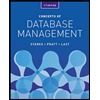
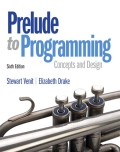
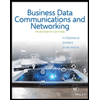