this is COmputer machine architecture ! Help me fix the following code as theres an error. what were trying to accoplish; The assignment is to create a MIPS program that corrects bad data using Hamming codes. The program is to request the user to enter a 12-bit Hamming code and determine if it is correct or not. If correct, it is to display a message to that effect. If incorrect, it is to display a message saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. I will be testing only with single bit errors, so the program should be able to correct my tests just fine. You do not need to worry about multiple bit errors. # This program corrects bad data using Hamming codes # It requests the user to enter a 12-bit Hamming code and determines if it is correct or not # If correct, it displays a message to that effect. If incorrect, it displays a message # saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. # This program is designed to handle single bit errors only. .data prompt: .asciiz "Enter a 12-bit Hamming code: " correct_msg: .asciiz "The Hamming code is correct." incorrect_msg: .asciiz "The Hamming code is incorrect. The correct code is: " newline: .asciiz "\n" .text .globl main main: # Display prompt to enter Hamming code li $v0, 4 la $a0, prompt syscall # Read Hamming code from user li $v0, 5 syscall move $t0, $v0 # Calculate parity bits andi $t1, $t0, 0xF # Parity bit p1 andi $t2, $t0, 0x696 # Parity bit p2 srl $t2, $t2, 1 xor $t1, $t1, $t2 andi $t2, $t0, 0xF00 # Parity bit p4 srl $t2, $t2, 4 xor $t1, $t1, $t2 andi $t2, $t0, 0xF000 # Parity bit p8 srl $t2, $t2, 8 xor $t1, $t1, $t2 # Check if Hamming code is correct beq $t1, $zero, print_correct_msg # Hamming code is incorrect, correct it li $t2, 1 sll $t2, $t2, ($t1 - 1) xor $t0, $t0, $t2 print_incorrect_msg: # Display incorrect message li $v0, 4 la $a0, incorrect_msg syscall # Display correct Hamming code li $v0, 34 move $a0, $t0 syscall # Display newline li $v0, 4 la $a0, newline syscall # Exit program li $v0, 10 syscall print_correct_msg: # Display correct message li $v0, 4 la $a0, correct_msg syscall # Exit program li $v0, 10 syscall Heres the error: line 73 column 5: "sll": Too many or incorrectly formatted operands. Expected: sll $t1,$t2,10 Assemble: operation completed with errors.
this is COmputer machine architecture !
Help me fix the following code as theres an error.
what were trying to accoplish;
The assignment is to create a MIPS
The program is to request the user to enter a 12-bit Hamming code and determine if it is correct or not. If correct, it is to display a message to that effect. If incorrect, it is to display a message saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex. I will be testing only with single bit errors, so the program should be able to correct my tests just fine. You do not need to worry about multiple bit errors.
# This program corrects bad data using Hamming codes
# It requests the user to enter a 12-bit Hamming code and determines if it is correct or not
# If correct, it displays a message to that effect. If incorrect, it displays a message
# saying it was incorrect and what the correct data is (the 12-bit Hamming code) again in hex.
# This program is designed to handle single bit errors only.
.data
prompt: .asciiz "Enter a 12-bit Hamming code: "
correct_msg: .asciiz "The Hamming code is correct."
incorrect_msg: .asciiz "The Hamming code is incorrect. The correct code is: "
newline: .asciiz "\n"
.text
.globl main
main:
# Display prompt to enter Hamming code
li $v0, 4
la $a0, prompt
syscall
# Read Hamming code from user
li $v0, 5
syscall
move $t0, $v0
# Calculate parity bits
andi $t1, $t0, 0xF # Parity bit p1
andi $t2, $t0, 0x696 # Parity bit p2
srl $t2, $t2, 1
xor $t1, $t1, $t2
andi $t2, $t0, 0xF00 # Parity bit p4
srl $t2, $t2, 4
xor $t1, $t1, $t2
andi $t2, $t0, 0xF000 # Parity bit p8
srl $t2, $t2, 8
xor $t1, $t1, $t2
# Check if Hamming code is correct
beq $t1, $zero, print_correct_msg
# Hamming code is incorrect, correct it
li $t2, 1
sll $t2, $t2, ($t1 - 1)
xor $t0, $t0, $t2
print_incorrect_msg:
# Display incorrect message
li $v0, 4
la $a0, incorrect_msg
syscall
# Display correct Hamming code
li $v0, 34
move $a0, $t0
syscall
# Display newline
li $v0, 4
la $a0, newline
syscall
# Exit program
li $v0, 10
syscall
print_correct_msg:
# Display correct message
li $v0, 4
la $a0, correct_msg
syscall
# Exit program
li $v0, 10
syscall
Heres the error:
line 73 column 5: "sll": Too many or incorrectly formatted operands. Expected: sll $t1,$t2,10
Assemble: operation completed with errors.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps

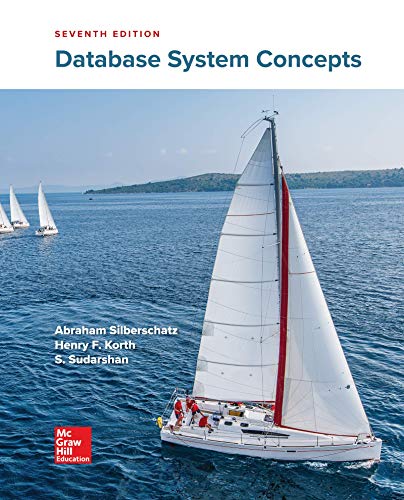
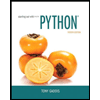
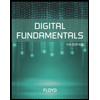
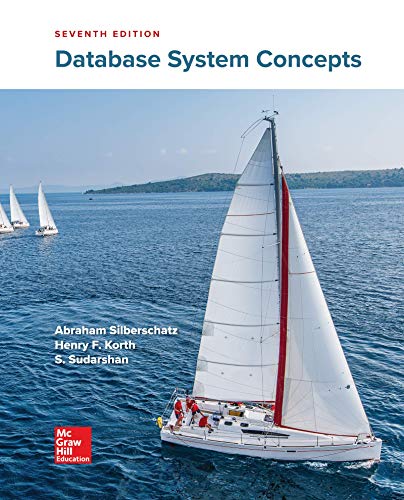
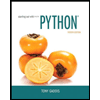
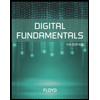
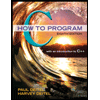
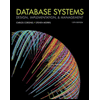
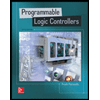