Third phase •Read in a vector of swim times •Build a loop, until quit is true: •Display the vector •Ask the user for an action (add, modify, quit) •Use a switch to select the action •Execute the action •Write out the changed vector •Hints: use a while (more) loop, use a menu / switch statement •Final phase
Can you help me with the second two phases because I have the first two done. My code is provided below:
First Phase
CODE
#include <iostream>
#include <vector>
using namespace std;
void print(vector<double> times){
int size = times.size();
for(int i=0;i<size;i++){
cout<<times[i]<<" ";
}
cout<<endl;
return;
}
int main(){
vector<double> times;
double input;
while(cin>>input){
times.push_back(input);
}
print(times);
return 0;
}
Second Phase
CODE
#include <iostream>
#include <string>
#include <vector>
#include <fstream>
#include <sstream>
using namespace std;
void display(vector<int> times) {
cout << "Your swim times are: ";
for (int i = 0; i < times.size(); i++) {
if (i < times.size() - 1) {
cout << times[i] << ", ";
}
else {
cout << times[i];
}
}
cout << endl;
}
int main() {
vector<int> times;
int time;
ifstream ifs("swimtimes.txt");
if (!ifs) {
cerr << "Failed to open file" << endl;
return 1;
}
string line;
while (getline(ifs, line)) {
stringstream ss(line);
ss >> time;
times.push_back(time);
}
display(times);
cout << "Please add a swim time." << endl;
cin >> time;
times.push_back(time);
cout << "Please select an index to modify." << endl;
int index;
cin >> index;
cout << "Please enter a new time." << endl;
cin >> time;
times[index] = time;
ofstream ofs("swimtimes.txt");
for (int i = 0; i < times.size(); i++) {
if (i < times.size() - 1) {
ofs << times[i] << endl;
}
else {
ofs << times[i];
}
}
}
Here are the final two phases I need help with:
Third phase
- •Read in a vector of swim times
- •Build a loop, until quit is true:
- •Display the vector
- •Ask the user for an action (add, modify, quit)
- •Use a switch to select the action
- •Execute the action
- •Write out the changed vector
- •Hints: use a while (more) loop, use a menu / switch statement
- •Create three
vectors : swim, run, bike - •Read in all three vectors
- •Modify the display function to show all three data sets
- •Modify the actions to ask which data set to add or modify
- •Write out all three data sets
- •Hint: need to go through all 3 vectors to see which one is longest before display

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Thank you so much! Just as a curiosity how would I add them all into one file because they all have their own start to the code and their own int mains
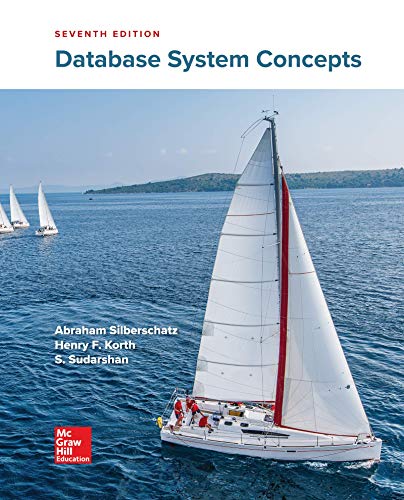
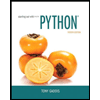
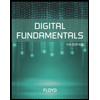
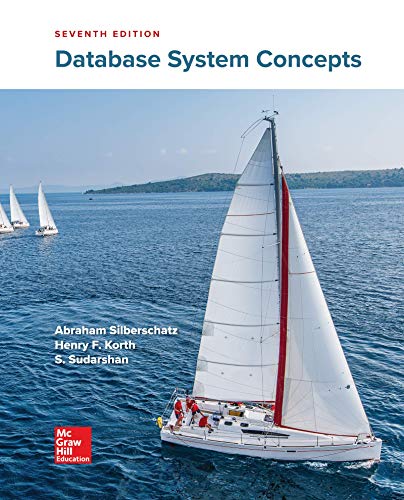
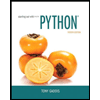
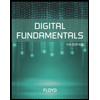
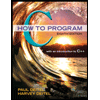
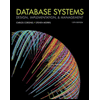
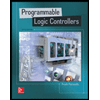