there is a problem with this code I want to delete node 10 and display the linked list after that it cannot be displayed and I don't know why please don't change the type of node from class to struct the code : #include using namespace std; class node { public: int data; node* next; }; void display(node * head) // printing the data of the nodes { node* temp = head; while (temp != NULL) { cout << temp->data << endl; temp = temp->next; } } // add void push(node** head, int newdata) // { 5, 10 , 20 , 30 , 40 } { node* newnode = new node(); newnode->data = newdata; newnode->next = *head; *head = newnode; } void append(node** head, int newdata) // { 10 , 20 , 30 , 40 } { node* newnode = new node(); newnode->data = newdata; newnode->next = NULL; node* last = *head; if (*head == NULL) { *head = newnode; return; } while (last->next != NULL) { last = last->next; } last->next = newnode; } void insertafter(node* previousnode, int newdata) // { 10 , 15 , 20 , 30 , 40 } { if (previousnode == NULL) { cout << "The previous node cannot be NULL"; } node* newnode = new node(); newnode->data = newdata; newnode->next = previousnode->next; previousnode->next = newnode; } //delete void remove(node* head, int target) // { 10 , 20 , 30 , 40 } { node* node2del; node* temp; temp = head; if (temp != NULL) { if (temp->data == target) { free(temp); head = head->next; //delete(temp); return; } while (temp->next != NULL) { if (temp->next->data == target) { node2del = temp->next; temp->next = temp->next->next; free(node2del); return; } temp = temp->next; } if (temp->data != target) { cout << "the item is not in the list %d" << target; } } return; } int main() { node* head = new node(); node* second = new node(); node* third = new node(); node* fourth = new node(); head->data = 10; head->next = second; second->data = 20; second->next = third; third->data = 30; third->next = fourth; fourth->data = 40; fourth->next = NULL; cout << "\nDisplay the linked list items \n"; display(head); /*cout << "\nPUSH \n"; push(&head, 5); display(head);*/ /*cout << "\nappend \n"; append(&head, 100); display(head);*/ //cout << "\nInsert after \n"; // { 10 , 20 , 30 , 15 , 40 } //insertafter(head->next->next, 15); //display(head); remove(head, 10); cout << "\nafter Delete \n"; display(head);
there is a problem with this code
I want to delete node 10 and display the linked list after that
it cannot be displayed and I don't know why
please don't change the type of node from class to struct
the code :
#include <iostream>
using namespace std;
class node
{
public:
int data;
node* next;
};
void display(node * head) // printing the data of the nodes
{
node* temp = head;
while (temp != NULL) {
cout << temp->data << endl;
temp = temp->next;
}
}
// add
void push(node** head, int newdata) // { 5, 10 , 20 , 30 , 40 }
{
node* newnode = new node();
newnode->data = newdata;
newnode->next = *head;
*head = newnode;
}
void append(node** head, int newdata) // { 10 , 20 , 30 , 40 }
{
node* newnode = new node();
newnode->data = newdata;
newnode->next = NULL;
node* last = *head;
if (*head == NULL) {
*head = newnode;
return;
}
while (last->next != NULL)
{
last = last->next;
}
last->next = newnode;
}
void insertafter(node* previousnode, int newdata) // { 10 , 15 , 20 , 30 , 40 }
{
if (previousnode == NULL) {
cout << "The previous node cannot be NULL";
}
node* newnode = new node();
newnode->data = newdata;
newnode->next = previousnode->next;
previousnode->next = newnode;
}
//delete
void remove(node* head, int target) // { 10 , 20 , 30 , 40 }
{
node* node2del;
node* temp;
temp = head;
if (temp != NULL) {
if (temp->data == target) {
free(temp);
head = head->next;
//delete(temp);
return;
}
while (temp->next != NULL) {
if (temp->next->data == target) {
node2del = temp->next;
temp->next = temp->next->next;
free(node2del);
return;
}
temp = temp->next;
}
if (temp->data != target) {
cout << "the item is not in the list %d" << target;
}
}
return;
}
int main()
{
node* head = new node();
node* second = new node();
node* third = new node();
node* fourth = new node();
head->data = 10;
head->next = second;
second->data = 20;
second->next = third;
third->data = 30;
third->next = fourth;
fourth->data = 40;
fourth->next = NULL;
cout << "\nDisplay the linked list items \n";
display(head);
/*cout << "\nPUSH \n";
push(&head, 5);
display(head);*/
/*cout << "\nappend \n";
append(&head, 100);
display(head);*/
//cout << "\nInsert after \n"; // { 10 , 20 , 30 , 15 , 40 }
//insertafter(head->next->next, 15);
//display(head);
remove(head, 10);
cout << "\nafter Delete \n";
display(head);
}

Step by step
Solved in 2 steps with 1 images

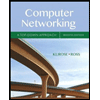
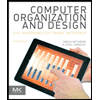
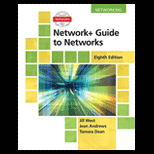
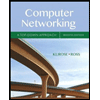
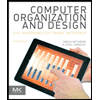
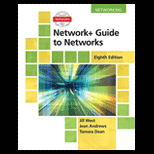
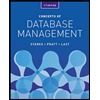
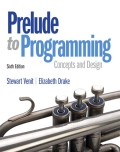
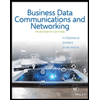