The Web Browser Web browsers are deceptively complex programs. The simple task of opening a web page carries a lot of steps: networking calls, protocol exchanges, rendering graphics, download and storage, JavaScript functionality, etc. It’s a lot to handle. The myriad number of tasks at hand provides a great example of different situations for collecting and managing information. Perfect for trying out the Java Collections Classes. Program requirements: • Create a new class, called “WebBrowser.” Inside it, place your main program. When run, your program will ask the user for commands. The following commands should be provided: o GOTO o DOWNLOAD o BACK o FORWARD o SHOW_DOWNLOADS o CLEAR_DOWNLOADS o SHOW_HISTORY o CLEAR_HISTORY o END • For the command above, should be any user-input String in the format of a typical web address. • Inside your WebBrowser main program, create the following: o A Stack of Strings called “backwards” o A Stack of Strings called “forwards” o A Queue of Strings called “downloads” o A List of Strings called “history” o A String called “currentURL” • The command GOTO should place the user’s entered into the currentURL. It should also add it to the “history” list. If currentURL was not empty, the previous URL should be added to the “backwards” Stack. Print out the currentURL • When the user types BACK, remove the top of the Stack “backwards” and place it in currentURL. Put the previous URL into the “forwards” Stack. If the “backwards” Stack is empty, alert the user that nothing happened. Print out the currentURL • When the user types FORWARD, remove the top of the Stack “forwards” and place it in currentURL. Put the previous URL into the “backwards” Stack. If the “forwards” Stack is empty, alert the user that nothing happened. Print out the currentURL • If a user types GOTO at any point, clear the “forwards” Stack. (Logically, once a user goes to a new site, the trail forward is lost.) • If a user types DOWNLOAD, add the currentURL to “downloads” • If a user types SHOW_DOWNLOADS, print the contents of “downloads” • If a user types CLEAR_DOWNLOADS, empty the contents of “downloads” • If a user types SHOW_HISTORY, print the contents of “history” • If a user types CLEAR_HISTORY, empty the contents of “history” • If a user types END, exit the program Include appropriate comments throughout your code.
The Web Browser Web browsers are deceptively complex programs. The simple task of opening a web page carries a lot of steps: networking calls, protocol exchanges, rendering graphics, download and storage, JavaScript functionality, etc. It’s a lot to handle. The myriad number of tasks at hand provides a great example of different situations for collecting and managing information. Perfect for trying out the Java Collections Classes. Program requirements: • Create a new class, called “WebBrowser.” Inside it, place your main program. When run, your program will ask the user for commands. The following commands should be provided: o GOTO o DOWNLOAD o BACK o FORWARD o SHOW_DOWNLOADS o CLEAR_DOWNLOADS o SHOW_HISTORY o CLEAR_HISTORY o END • For the command above, should be any user-input String in the format of a typical web address. • Inside your WebBrowser main program, create the following: o A Stack of Strings called “backwards” o A Stack of Strings called “forwards” o A Queue of Strings called “downloads” o A List of Strings called “history” o A String called “currentURL” • The command GOTO should place the user’s entered into the currentURL. It should also add it to the “history” list. If currentURL was not empty, the previous URL should be added to the “backwards” Stack. Print out the currentURL • When the user types BACK, remove the top of the Stack “backwards” and place it in currentURL. Put the previous URL into the “forwards” Stack. If the “backwards” Stack is empty, alert the user that nothing happened. Print out the currentURL • When the user types FORWARD, remove the top of the Stack “forwards” and place it in currentURL. Put the previous URL into the “backwards” Stack. If the “forwards” Stack is empty, alert the user that nothing happened. Print out the currentURL • If a user types GOTO at any point, clear the “forwards” Stack. (Logically, once a user goes to a new site, the trail forward is lost.) • If a user types DOWNLOAD, add the currentURL to “downloads” • If a user types SHOW_DOWNLOADS, print the contents of “downloads” • If a user types CLEAR_DOWNLOADS, empty the contents of “downloads” • If a user types SHOW_HISTORY, print the contents of “history” • If a user types CLEAR_HISTORY, empty the contents of “history” • If a user types END, exit the program Include appropriate comments throughout your code.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java Programming Assignment 6 – The Web Browser
Web browsers are deceptively complex programs. The simple task of opening a web page carries a lot of steps: networking calls, protocol exchanges, rendering graphics, download and storage, JavaScript functionality, etc. It’s a lot to handle. The myriad number of tasks at hand provides a great example of different situations for collecting and managing information. Perfect for trying out the Java Collections Classes.
Program requirements:
• Create a new class, called “WebBrowser.” Inside it, place your main program. When run, your program will ask the user for commands.
The following commands should be provided:
o GOTO
o DOWNLOAD
o BACK
o FORWARD
o SHOW_DOWNLOADS
o CLEAR_DOWNLOADS
o SHOW_HISTORY
o CLEAR_HISTORY
o END
• For the command above, should be any user-input String in the format of a typical web address.
• Inside your WebBrowser main program, create the following:
o A Stack of Strings called “backwards”
o A Stack of Strings called “forwards”
o A Queue of Strings called “downloads”
o A List of Strings called “history”
o A String called “currentURL”
• The command GOTO should place the user’s entered into the currentURL. It should also add it to the “history” list. If currentURL was not empty, the previous URL should be added to the “backwards” Stack. Print out the currentURL
• When the user types BACK, remove the top of the Stack “backwards” and place it in currentURL. Put the previous URL into the “forwards” Stack. If the “backwards” Stack is empty, alert the user that nothing happened. Print out the currentURL
• When the user types FORWARD, remove the top of the Stack “forwards” and place it in currentURL. Put the previous URL into the “backwards” Stack. If the “forwards” Stack is empty, alert the user that nothing happened. Print out the currentURL
• If a user types GOTO at any point, clear the “forwards” Stack. (Logically, once a user goes to a new site, the trail forward is lost.)
• If a user types DOWNLOAD, add the currentURL to “downloads”
• If a user types SHOW_DOWNLOADS, print the contents of “downloads”
• If a user types CLEAR_DOWNLOADS, empty the contents of “downloads”
• If a user types SHOW_HISTORY, print the contents of “history”
• If a user types CLEAR_HISTORY, empty the contents of “history”
• If a user types END, exit the program
Include appropriate comments throughout your code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
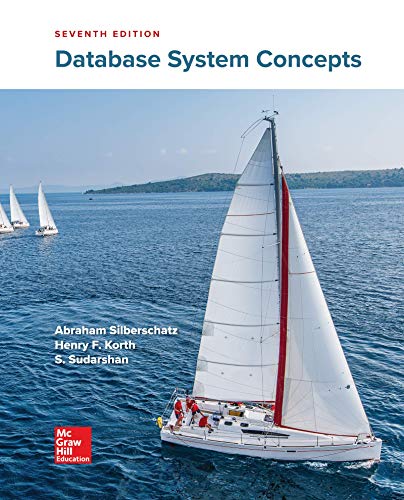
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
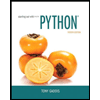
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
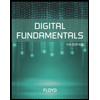
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
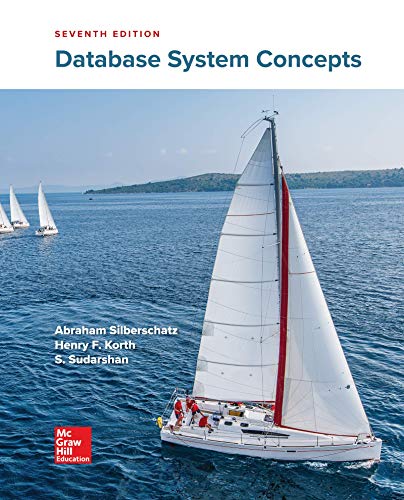
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
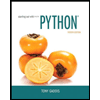
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
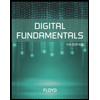
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
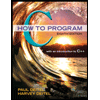
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
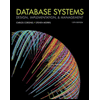
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
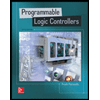
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education