The purpose of this project is to demonstrate an acceptable level of expertise with the basic programming concepts/techniques and Python syntax addressed through the semester. This includes (but is not necessarily limited to): data types, variables, operators, expressions, statements, I/O operations, user-defined and built-in functions, modules and control structures, dictionary and files. The source code should be written in the template files that are being provided with the header and above specification. Details code comments
The purpose of this project is to demonstrate an acceptable level of expertise with the basic programming concepts/techniques and Python syntax addressed through the semester. This includes (but is not necessarily limited to): data types, variables, operators, expressions, statements, I/O operations, user-defined and built-in functions, modules and control structures, dictionary and files.
The source code should be written in the template files that are being provided with the header and above specification. Details code comments are provided in the given files
Input file: The program starts with reading in all user information from a given input file. The input file contains information of a number of users in the following order: username, first name, last name, password, miniVenmo account number and account balance. Information is separated with ‘|’. o username is a unique information, so no two users will have same username. Sample input file: User gmason has password 123456, account number of BB12 and balance of $1000. Program flows as below: 1) data is read in from the given input file, 2) it will be saved to a dictionary referred as users where each key is username and the value will be a list containing rest of the information: first name, last name, password, account number and account balance. One example of key-value pair is: 'gmason': ['George', 'Mason', '123456', 'BB12', 1000.0]. 3) user will be presented with a menu and all the operations related to menu options will be performed on this users dictionary. Main Menu: The program starts with a welcome message and displays main menu. Main menu contains following different options for a user: Main Menu 1. New User 2. Existing User Sign In 3. Exit Following is description of all the options. Black refers to user input and blue is program generated message/options. For better understanding, some comments in orange italics are added surrounded by triangle brackets <>, these are not program generated messages.
MAKE SURE TO FOLLOW THE STRUCTURED CODE BELOW
AND THE TEXT FILE THAT NEEDS TO BE USED
gmason|George|Mason|123456|BB12|1000 gwash|George|Washington|45678910|AB45|900
user_list.txt
IMAGES POSTED APPLICATION.PNG AND UTILITY.PNG USE SCREENSHOTS TO STRUCTURE CODE



Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

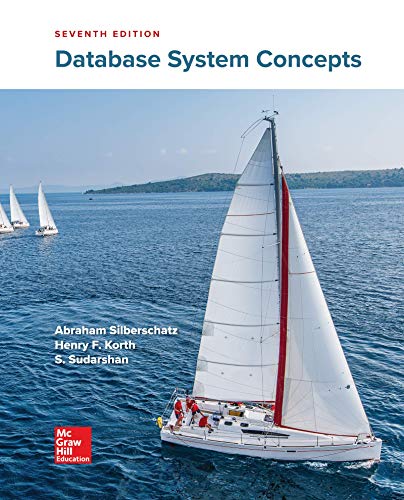
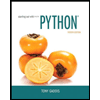
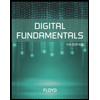
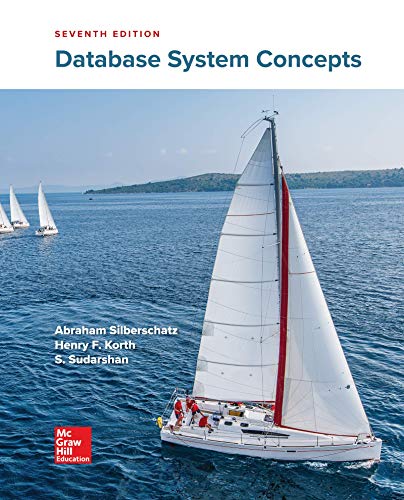
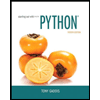
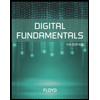
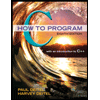
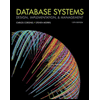
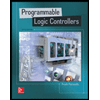