The polynomial is of the generic form: p(x) = anx"+an-1 Xn-¹+an-2 X-2+...+a2 x²+a₁ x+ao We will assume that the coefficient values a0 through an are all known and constant and stored in an array. This means that the evaluation of the polynomial has only the value x as its input and will return the resulting polynomial value as it output. The standard evaluation algorithm is straightforward: Evaluate (x) { //x the value to use for evaluation of the polynomial result=a[0]+ a[1]"x xPower=x for i=2 to n do xPower=xPower"x| result=result +a[i]"xPower end for return result. 1- Find the number of additions and multiplications outside the for loop 2- Find the number of additions inside the for loop 3- Find the number of multiplications inside the for loop 4- Find the number of times the for loop executes 5- Find the number of operations of the function Evaluate(x)
The polynomial is of the generic form: p(x) = anx"+an-1 Xn-¹+an-2 X-2+...+a2 x²+a₁ x+ao We will assume that the coefficient values a0 through an are all known and constant and stored in an array. This means that the evaluation of the polynomial has only the value x as its input and will return the resulting polynomial value as it output. The standard evaluation algorithm is straightforward: Evaluate (x) { //x the value to use for evaluation of the polynomial result=a[0]+ a[1]"x xPower=x for i=2 to n do xPower=xPower"x| result=result +a[i]"xPower end for return result. 1- Find the number of additions and multiplications outside the for loop 2- Find the number of additions inside the for loop 3- Find the number of multiplications inside the for loop 4- Find the number of times the for loop executes 5- Find the number of operations of the function Evaluate(x)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Hello can you please help me do this exercise. Can you do part 1, 2, 3, 4 and 5 (marked in red please)

The polynomial is of the generic form:
\[ p(x) = a_n x^n + a_{n-1} x^{n-1} + a_{n-2} x^{n-2} + \ldots + a_2 x^2 + a_1 x + a_0 \]
We will assume that the coefficient values \( a_0 \) through \( a_n \) are all known and constant and stored in an array. This means that the evaluation of the polynomial has only the value \( x \) as its input and will return the resulting polynomial value as its output.
The standard evaluation algorithm is straightforward:
```
Evaluate (x) {
// x the value to use for evaluation of the polynomial
result = a[0] + a[1] * x
xPower = x
for i = 2 to n do
xPower = xPower * x
result = result + a[i] * xPower
end for
return result.
}
```
1. **Find the number of additions and multiplications outside the for loop.**
2. **Find the number of additions inside the for loop.**
3. **Find the number of multiplications inside the for loop.**
4. **Find the number of times the for loop executes.**
5. **Find the number of operations of the function Evaluate(x).**](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F549651ba-b33d-474d-abf4-48863f7a8bc4%2F81238564-75bb-4ca2-9eef-89758af7bc01%2F1t7rqgi_processed.png&w=3840&q=75)
Transcribed Image Text:**Exercise 4:**
The following algorithm computes the sum of a polynomial of power n.
For an explanation of what a polynomial is, consult:
[Polynomial Explanation](https://www.mathcentre.ac.uk/resources/uploaded/mc-ty-polynomial-2009-1.pdf)
The polynomial is of the generic form:
\[ p(x) = a_n x^n + a_{n-1} x^{n-1} + a_{n-2} x^{n-2} + \ldots + a_2 x^2 + a_1 x + a_0 \]
We will assume that the coefficient values \( a_0 \) through \( a_n \) are all known and constant and stored in an array. This means that the evaluation of the polynomial has only the value \( x \) as its input and will return the resulting polynomial value as its output.
The standard evaluation algorithm is straightforward:
```
Evaluate (x) {
// x the value to use for evaluation of the polynomial
result = a[0] + a[1] * x
xPower = x
for i = 2 to n do
xPower = xPower * x
result = result + a[i] * xPower
end for
return result.
}
```
1. **Find the number of additions and multiplications outside the for loop.**
2. **Find the number of additions inside the for loop.**
3. **Find the number of multiplications inside the for loop.**
4. **Find the number of times the for loop executes.**
5. **Find the number of operations of the function Evaluate(x).**
![**Polynomial Evaluation Using Factorization**
To efficiently evaluate a polynomial, it can be factored into the following form:
\[ p(x) = ((\ldots((a_nx + a_{n-1})x + a_{n-2})x + \ldots + a_2)x + a_1)x + a_0 \]
This can be expressed algorithmically as:
**BetterEvaluate(x)**
- **// x**: the value to use for evaluation of the polynomial
- **result = a[n]**
For **i = n-1** down to **0** do
- **result = result * x**
- **result = result + a[i]**
End For
Return **result**.
**Exercises**
1. Answer questions 1-5 regarding the algorithm BetterEvaluate(x).
2. How many multiplications were saved by applying BetterEvaluate(x) rather than Evaluate(x)?
**Extra Credit (20 pts)**
- Implement each of the algorithms for **x = 2** and \(\text{a[10]} = \{1, 2, 3, 4, 5, 6, 7, 8, 9, 10\}\).](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F549651ba-b33d-474d-abf4-48863f7a8bc4%2F81238564-75bb-4ca2-9eef-89758af7bc01%2F8mfci6s_processed.png&w=3840&q=75)
Transcribed Image Text:**Polynomial Evaluation Using Factorization**
To efficiently evaluate a polynomial, it can be factored into the following form:
\[ p(x) = ((\ldots((a_nx + a_{n-1})x + a_{n-2})x + \ldots + a_2)x + a_1)x + a_0 \]
This can be expressed algorithmically as:
**BetterEvaluate(x)**
- **// x**: the value to use for evaluation of the polynomial
- **result = a[n]**
For **i = n-1** down to **0** do
- **result = result * x**
- **result = result + a[i]**
End For
Return **result**.
**Exercises**
1. Answer questions 1-5 regarding the algorithm BetterEvaluate(x).
2. How many multiplications were saved by applying BetterEvaluate(x) rather than Evaluate(x)?
**Extra Credit (20 pts)**
- Implement each of the algorithms for **x = 2** and \(\text{a[10]} = \{1, 2, 3, 4, 5, 6, 7, 8, 9, 10\}\).
Expert Solution

Step 1: Introduce polynomial evoulution:
Polynomial evaluation is a fundamental mathematical operation where a polynomial expression is computed for a given value of its variable. The generic form of a polynomial consists of coefficients stored in an array, and a standard evaluation algorithm is used to calculate the polynomial's value efficiently. Understanding the number of additions, multiplications, and loop iterations in the evaluation process is essential for optimizing computational efficiency.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
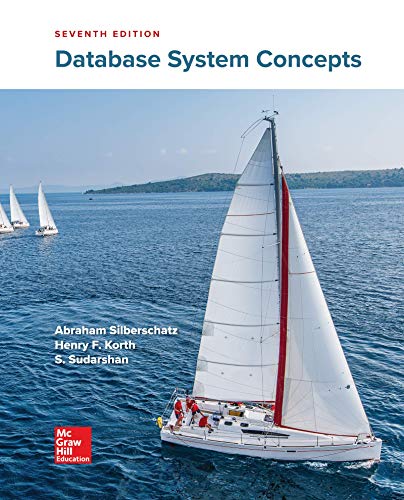
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
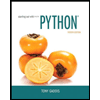
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
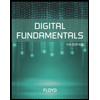
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
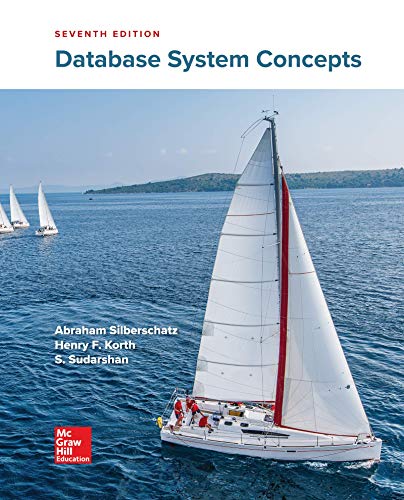
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
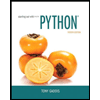
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
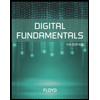
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
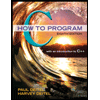
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
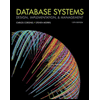
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
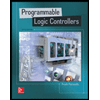
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education