The language I am using is Java The source code for Shortest Job First Algorithm is already given. Please help me to create a GUI for my code for CPU Scheduling: Shortest Job First. I have created the design for the GUI using windows builder in Java Eclipse. I can't fully upload the code for my GUI because of the word limit in Bartleby. Therefore, you can do your own design of the GUI with the code in it. I need a functional and working code for the GUI where I can input the desired number I want for the burst time and arrival time. I hope you can help me with this. Thank you so much. package test; import java.util.*; import javax.swing.JOptionPane; public class SJF { public static void main(String args[]) {
The language I am using is Java
The source code for Shortest Job First
Please help me to create a GUI for my code for CPU Scheduling: Shortest Job First. I have created the design for the GUI using windows builder in Java Eclipse.
I can't fully upload the code for my GUI because of the word limit in Bartleby. Therefore, you can do your own design of the GUI with the code in it. I need a functional and working code for the GUI where I can input the desired number I want for the burst time and arrival time. I hope you can help me with this. Thank you so much.
package test;
import java.util.*;
import javax.swing.JOptionPane;
public class SJF {
public static void main(String args[])
{
Scanner sc = new Scanner(System.in);
int x= Integer.parseInt(JOptionPane.showInputDialog("Enter number of process"));
int processID[] = new int[x]; //To display the process ID
int arTime[] = new int[x]; // arTime means arrival time
int burstTime[] = new int[x]; // burstTime means burst time
int complTime[] = new int[x]; // complTime means complete time
int TAT[] = new int[x]; // TAT means turn around time
int waitingTime[] = new int[x]; //waitingTime means waiting time
int process[] = new int[x]; // Checks if the process is completed
int ganttChart[] = new int[x]; //To display the Gantt Chart
int startTime=0, totalProcess=0; //startTime = start time and total process time to check if the process if finished to break from the while loop
float avrgWT=0, avrgTAT=0;
for(int i=0;i<x;i++) //for loop to get the arrival time and burst time
{
arTime[i] = Integer.parseInt(JOptionPane.showInputDialog("Input Arrival Time " + (i+1)));
burstTime[i] = Integer.parseInt(JOptionPane.showInputDialog("Input Burst Time " + (i+1)));
processID[i] = i+1;
process[i] = 0;
}
while(true)
{
int c=x, min=1000; //Looking for the smallest burst time
if (totalProcess == x) // total no of process = completed process loop will be terminated
break;
for (int i=0; i<x; i++)
{
if ((arTime[i] <= startTime) && (process[i] == 0) && (burstTime[i]<min))
{
min=burstTime[i];
c=i;
}
}
if (c==x)
startTime++;
else
{
complTime[c]=startTime+burstTime[c];
startTime+=burstTime[c];
TAT[c]=complTime[c]-arTime[c];
waitingTime[c]=TAT[c]-burstTime[c];
process[c]=1;
ganttChart[totalProcess] = c + 1;
totalProcess++;
}
}
for(int i=0;i<x;i++)
{
avrgWT+= waitingTime[i];
avrgTAT+= TAT[i];
System.out.println("Process ID: "+processID[i]+"\t"+"Arrival Time: "+arTime[i]+"\t"+" Burst Time: "+burstTime[i]+"\t"+"Completion Time: "+complTime[i]+"\t"+"Turn Around Time: "+TAT[i]+"\t"+"Waiting Time: "+waitingTime[i]);
}
System.out.println ("\nAverage TAT is "+ (float)(avrgTAT/x));
System.out.println ("Average Waiting Time is "+ (float)(avrgWT/x));
sc.close();
System.out.print("The Gantt Chart is ");
for(int i=0; i<x; i++)
{
System.out.print("P"+ganttChart[i]+ " ");
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

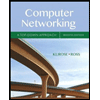
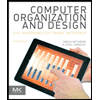
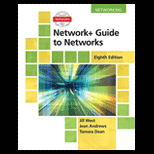
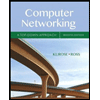
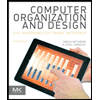
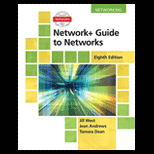
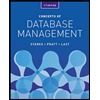
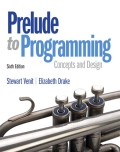
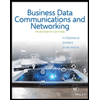