The following code prints all values less the 7 sorted to Console: using System; using System.Collections.Generic; using System.Linq; namespace ConsoleApp2 { class LINQtoArray { static void Main(string[] args) { int[] array = { 2, 6, 4, 12, 7, 8, 9, 13, 2 }; var orderedFilteredArray = from element in array where element < 7 orderby element select element; PrintArray(orderedFilteredArray, "All values less than 7 and sorted:"); } public static void PrintArray(IEnumerable arr, string message) { Console.WriteLine("{0}", message); foreach (var element in arr) Console.WriteLine(" {0}", element); Console.WriteLine(); } } } Rewrite this code so that it displays values more or equal to 6 in descending order. Use the following array, not the one above: int[] array = { 2,7,9,3,6,1,7,4,2,10 };
The following code prints all values less the 7 sorted to Console:
using System;
using System.Collections.Generic;
using System.Linq;
namespace ConsoleApp2
{
class LINQtoArray
{
static void Main(string[] args)
{
int[] array = { 2, 6, 4, 12, 7, 8, 9, 13, 2 };
var orderedFilteredArray =
from element in array
where element < 7
orderby element
select element;
PrintArray(orderedFilteredArray,
"All values less than 7 and sorted:");
}
public static void PrintArray(IEnumerable<int> arr, string message)
{
Console.WriteLine("{0}", message);
foreach (var element in arr)
Console.WriteLine(" {0}", element);
Console.WriteLine();
}
}
}
Rewrite this code so that it displays values more or equal to 6 in descending order.
Use the following array, not the one above:
int[] array = { 2,7,9,3,6,1,7,4,2,10 };

Step by step
Solved in 2 steps

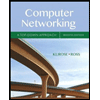
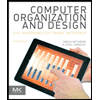
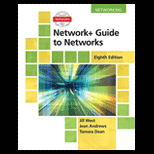
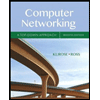
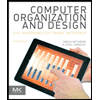
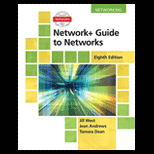
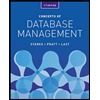
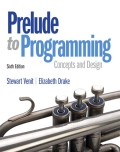
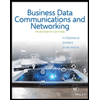