The following code I have written is supposed to check if the first character of the first given command line argument is the same as the last character of the last command line argument. However, I keep getting false when I am running it. Why is this occurring? #include <iostream>#include <string>using namespace std; #define N 1000 int checkChar(char str[]) { //method to check first and last char in string int n = strlen(str); char first = str[0], last = str[n - 1]; //if first equals to last then returns 1 if (first == last) return 1; //otherwise 0 else return 0; } //end of checkChar int main(int argc, char* argv[]) { //main method int check; //check variable which stores either 0 or 1 // new str string with initial size N char str[N]; for (int i = 1; i < argc; i++) { //for-loop to copy argv array element in str char type array //strcat_s(str, argv[i]);str[i] = *argv[i]; } //calling method checkChar by passing argument str check = checkChar(str); if (check) // when check have 1 (both are same) cout << "true\n"; else //when check have 0 (both are not same) cout << "false\n"; return 0; } //end of main
The following code I have written is supposed to check if the first character of the first given command line argument is the same as the last character of the last command line argument. However, I keep getting false when I am running it. Why is this occurring?
#include <iostream>
#include <string>
using namespace std;
#define N 1000
int checkChar(char str[]) { //method to check first and last char in string
int n = strlen(str);
char first = str[0], last = str[n - 1];
//if first equals to last then returns 1
if (first == last) return 1;
//otherwise 0
else return 0;
} //end of checkChar
int main(int argc, char* argv[]) { //main method
int check; //check variable which stores either 0 or 1
// new str string with initial size N
char str[N];
for (int i = 1; i < argc; i++) { //for-loop to copy argv array element in str char type array
//strcat_s(str, argv[i]);
str[i] = *argv[i];
}
//calling method checkChar by passing argument str
check = checkChar(str);
if (check)
// when check have 1 (both are same)
cout << "true\n";
else
//when check have 0 (both are not same)
cout << "false\n";
return 0;
} //end of main

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

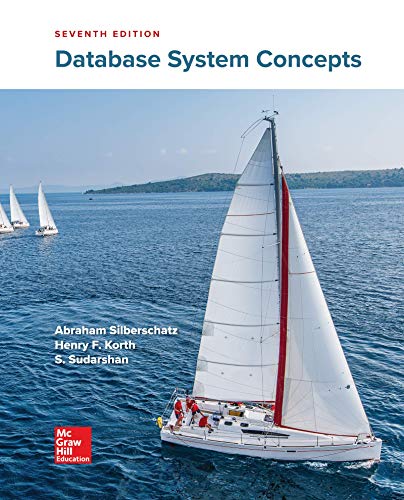
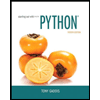
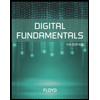
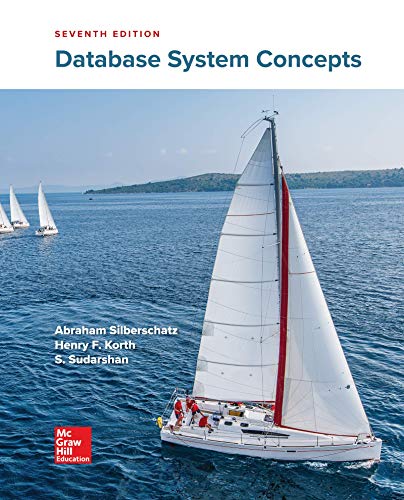
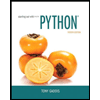
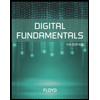
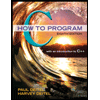
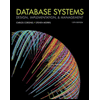
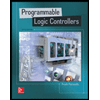