The first image is the spec. The second image is my code, what/where should I fix?
The first image is the spec. The second image is my code, what/where should I fix?
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The first image is the spec.
The second image is my code, what/where should I fix?
![```java
import java.util.*;
public class CountEvens {
public static void main(String[] args) {
Random r = new Random();
// Generate 10 values between 0 and 100
System.out.println(countEvens(10, 100, r));
// Generate 100 values between 0 and 10
System.out.println(countEvens(100, 10, r));
// Generate 1 value between 0 and 5
System.out.println(countEvens(1, 5, r));
// Generate 8 values between 0 and 37
System.out.println(countEvens(8, 37, r));
}
// Write your countEvens method here
public static int countEvens(int times, int max, Random r) {
int number = r.nextInt(max - 0 + 1) + 0;
if (number % 2 == 0) {
System.out.print(number);
}
return number;
}
}
```
### Description
This Java program defines a class `CountEvens` which includes a `main` method that uses a `countEvens` method to generate random numbers within specified ranges and counts how many of these numbers are even.
- **Lines 1-2:** Import necessary Java utilities.
- **Line 4:** Declares the main class `CountEvens`.
- **Line 5:** The `main` method begins execution, setting up a random number generator `r`.
### Code Execution:
- **Lines 8-17:** Several calls to `countEvens` output the number of even numbers generated. The ranges and times of generation are specified:
- **Line 8:** Generates 10 random numbers between 0 and 100.
- **Line 11:** Generates 100 random numbers between 0 and 10.
- **Line 14:** Generates 1 random number between 0 and 5.
- **Line 17:** Generates 8 random numbers between 0 and 37.
### `countEvens` Method:
- **Lines 22-27:** The `countEvens` method generates a number of random integers and checks if each one is even.
- **Line 23:** Generates a random integer within the specified range (0 to `max`).
- **Lines 24-26:** Checks](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F6c680976-d041-43f6-b2b7-59630778639d%2Fde8e486f-3630-43e2-b70e-a009e995bc7c%2Fc55nled_processed.jpeg&w=3840&q=75)
Transcribed Image Text:```java
import java.util.*;
public class CountEvens {
public static void main(String[] args) {
Random r = new Random();
// Generate 10 values between 0 and 100
System.out.println(countEvens(10, 100, r));
// Generate 100 values between 0 and 10
System.out.println(countEvens(100, 10, r));
// Generate 1 value between 0 and 5
System.out.println(countEvens(1, 5, r));
// Generate 8 values between 0 and 37
System.out.println(countEvens(8, 37, r));
}
// Write your countEvens method here
public static int countEvens(int times, int max, Random r) {
int number = r.nextInt(max - 0 + 1) + 0;
if (number % 2 == 0) {
System.out.print(number);
}
return number;
}
}
```
### Description
This Java program defines a class `CountEvens` which includes a `main` method that uses a `countEvens` method to generate random numbers within specified ranges and counts how many of these numbers are even.
- **Lines 1-2:** Import necessary Java utilities.
- **Line 4:** Declares the main class `CountEvens`.
- **Line 5:** The `main` method begins execution, setting up a random number generator `r`.
### Code Execution:
- **Lines 8-17:** Several calls to `countEvens` output the number of even numbers generated. The ranges and times of generation are specified:
- **Line 8:** Generates 10 random numbers between 0 and 100.
- **Line 11:** Generates 100 random numbers between 0 and 10.
- **Line 14:** Generates 1 random number between 0 and 5.
- **Line 17:** Generates 8 random numbers between 0 and 37.
### `countEvens` Method:
- **Lines 22-27:** The `countEvens` method generates a number of random integers and checks if each one is even.
- **Line 23:** Generates a random integer within the specified range (0 to `max`).
- **Lines 24-26:** Checks

Transcribed Image Text:### Java Programming: Writing a Method to Count Even Numbers
#### Objective
Create a static method called `countEvens()` that generates a series of random numbers and returns the count of even numbers. This method should take three parameters:
- `int times` - the number of random numbers to generate.
- `int max` - the maximum possible value for the generated numbers.
- `Random r` - the `Random` object used for number generation.
#### Method Details
The method should generate `times` random integers between 0 and `max` (inclusive) utilizing the `Random` object `r`. It should then return the count of numbers that are even. You can assume that both `times` and `max` are positive integers greater than 0.
#### Hint
Utilize the Java modulo (`%`) operator to determine even numbers.
#### Testing the Method
A main method has been provided to test your `countEvens` method. Ensure your method is written to avoid errors with the provided main method. Note that these tests are not exhaustive.
#### Code Example
```java
public static int countEvens(int times, int max, Random r) {
int number = 0;
for (int i = 1; i <= times; i++) {
number = r.nextInt(max + 1);
if (number % 2 == 0) {
System.out.print(number + " ");
}
}
return number;
}
```
#### Console Output Example
This section displays generated numbers from several method calls:
- `34 72 0 46 4 74 16 61`
- `8 8 4 2 8 0 4 8 4 2 0 2 4 8 8 2 4 6 4 0 2`
The output represents the numbers generated during each run, with even numbers highlighted.
#### Conclusion
This exercise helps in understanding how to work with random number generation and condition checking in Java. Review the results to ensure the method works correctly, especially with random inputs.
Ensure your code is robust by testing edge cases. Click "Expand" to see grading criteria for this problem.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 3 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
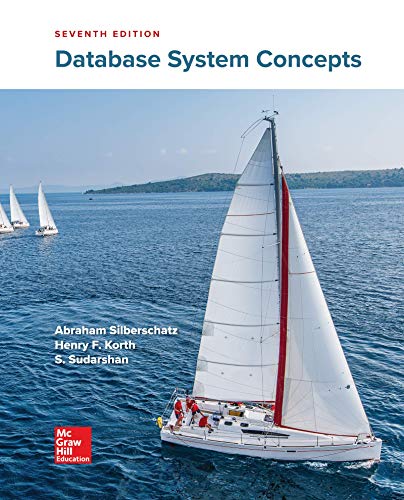
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
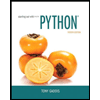
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
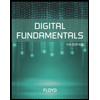
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
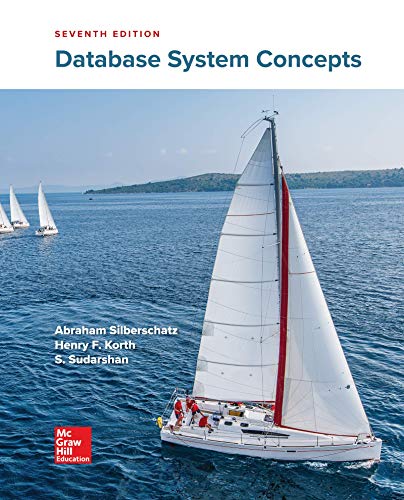
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
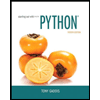
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
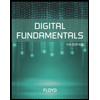
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
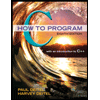
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
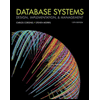
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
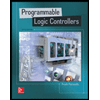
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education