The circular buffer should be placed in shared memory so that each process can access the buffer to support inter-process communication. The following code snippets can be used as guidance for implementing a shared memory buffer. Your objective is to implement the shared circular buffer along with put() and get() functions to access the buffer. Each process function (i.e., producer() and consumer()) will access the circular buffer where your objective is to ensure that the producer is always ahead of the consumer and the consumer does not have to wait. This is illustrated in the following diagram: (Image below) Signals should be used between the two processes. The consumer should put itself to sleep if there is no data in the buffer and wait for a signal from the producer indicating data has been placed in the buffer. The producer should put itself to sleep if the buffer is full and wait for a signal from the consumer that there is room in the buffer to start writing again. An explanation of your approach to implementation and its reasoning. Coding results: All the source code files. Comment your code, describing the solution and identifying the programmer. Screenshots showing successful and correct execution of the code.
The circular buffer should be placed in shared memory so that each process can access the buffer to support inter-process communication. The following code snippets can be used as guidance for implementing a shared memory buffer.
Your objective is to implement the shared circular buffer along with put() and get() functions to access the buffer. Each process function (i.e., producer() and consumer()) will access the circular buffer where your objective is to ensure that the producer is always ahead of the consumer and the consumer does not have to wait. This is illustrated in the following diagram:
(Image below)
Signals should be used between the two processes. The consumer should put itself to sleep if there is no data in the buffer and wait for a signal from the producer indicating data has been placed in the buffer. The producer should put itself to sleep if the buffer is full and wait for a signal from the consumer that there is room in the buffer to start writing again.
An explanation of your approach to implementation and its reasoning.
Coding results:
-
- All the source code files. Comment your code, describing the solution and identifying the programmer.
- Screenshots showing successful and correct execution of the code.

![// Shared Circular Buffer
struct CIRCULAR_BUFFER
{
int count;
int lower;
int upper;
int buffer[100];
// Number of items in the buffer
// Next slot to read in the buffer
// Next slot to write in the buffer
};\_\
struct CIRCULAR_BUFFER *buffer = NULL;
// Create shared memory for the Circular Buffer to be shared between the Parent and Child Processes
buffer= (struct CIRCULAR_BUFFER*)mmap(0, sizeof (buffer), PROT_READ | PROT_WRITE, MAP_SHARED | MAP_ANONYMOUS, -1, 0);
buffer->count = 0;
buffer->lower = 0;
buffer->upper = 0;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Ffe488bf4-ebd7-4956-8308-f4f73186902c%2F5d27f4bc-b93e-4c74-ae37-ccacd3204224%2F99vavx_processed.png&w=3840&q=75)

Step by step
Solved in 6 steps with 1 images

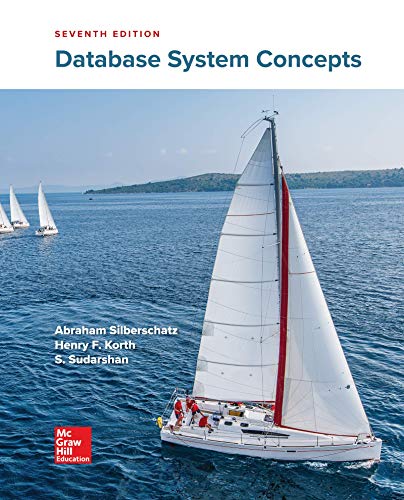
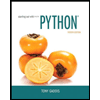
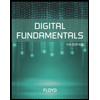
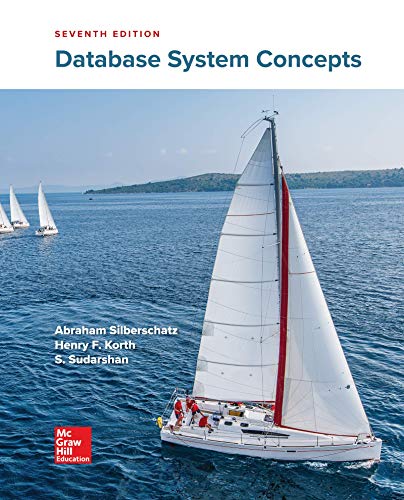
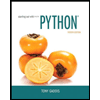
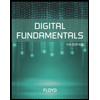
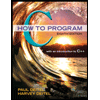
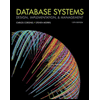
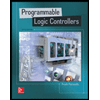