The C# script below gives out this output: Boat #0201601 from WI has a 30 HP motor. The price for the license is $25.00 Boat #0201602 from MI has a 50 HP motor. The price for the license is $25.00 Boat #0201603 from MN has a 100 HP motor. The price for the license is $38.00 However I want the output to look like this: Boat #0201601 from WI has a 30 HP motor. The price for the license is $25.00 Boat #1201601 from MI has a 50 HP motor. The price for the license is $25.00 Boat #2201601 from MN has a 100 HP motor. The price for the license is $38.00 How do I change the script to get the desired output? using System; using static System.Console; using System.Globalization; class FixedDebugNine4 { static void Main() { const int STARTING_NUM = 201601; BoatLicense[] license = new BoatLicense[3]; int x; for (x = 0; x < license.Length; ++x) { license[x] = new BoatLicense(); license[x].LicenseNum = (x + STARTING_NUM).ToString("D7"); } license[0].State = "WI"; license[1].State = "MI"; license[2].State = "MN"; license[0].MotorSizeInHP = 30; license[1].MotorSizeInHP = 50; license[2].MotorSizeInHP = 100; for (x = 0; x < license.Length; ++x) Display(license[x]); } static void Display(BoatLicense lic) { WriteLine("Boat #{0} from {1} has a {2} HP motor.", lic.LicenseNum, lic.State, lic.MotorSizeInHP); WriteLine("The price for the license is {0}\n", lic.Price.ToString("C2", CultureInfo.GetCultureInfo("en-US"))); } } class BoatLicense { public const int HPCUTOFF = 50; public const double LOWFEE = 25.00; public const double HIGHFEE = 38.00; private string licenseNum; private string state; private int motorSizeInHP; private double price; public string LicenseNum { get { return licenseNum; } set { licenseNum = value; } } public string State { get { return state; } set { state = value; } } public int MotorSizeInHP { get { return motorSizeInHP; } set { motorSizeInHP = value; if (motorSizeInHP <= HPCUTOFF) price = LOWFEE; else price = HIGHFEE; } } public double Price { get { return price; } } }
The C# script below gives out this output: Boat #0201601 from WI has a 30 HP motor. The price for the license is $25.00 Boat #0201602 from MI has a 50 HP motor. The price for the license is $25.00 Boat #0201603 from MN has a 100 HP motor. The price for the license is $38.00 However I want the output to look like this: Boat #0201601 from WI has a 30 HP motor. The price for the license is $25.00 Boat #1201601 from MI has a 50 HP motor. The price for the license is $25.00 Boat #2201601 from MN has a 100 HP motor. The price for the license is $38.00 How do I change the script to get the desired output? using System; using static System.Console; using System.Globalization; class FixedDebugNine4 { static void Main() { const int STARTING_NUM = 201601; BoatLicense[] license = new BoatLicense[3]; int x; for (x = 0; x < license.Length; ++x) { license[x] = new BoatLicense(); license[x].LicenseNum = (x + STARTING_NUM).ToString("D7"); } license[0].State = "WI"; license[1].State = "MI"; license[2].State = "MN"; license[0].MotorSizeInHP = 30; license[1].MotorSizeInHP = 50; license[2].MotorSizeInHP = 100; for (x = 0; x < license.Length; ++x) Display(license[x]); } static void Display(BoatLicense lic) { WriteLine("Boat #{0} from {1} has a {2} HP motor.", lic.LicenseNum, lic.State, lic.MotorSizeInHP); WriteLine("The price for the license is {0}\n", lic.Price.ToString("C2", CultureInfo.GetCultureInfo("en-US"))); } } class BoatLicense { public const int HPCUTOFF = 50; public const double LOWFEE = 25.00; public const double HIGHFEE = 38.00; private string licenseNum; private string state; private int motorSizeInHP; private double price; public string LicenseNum { get { return licenseNum; } set { licenseNum = value; } } public string State { get { return state; } set { state = value; } } public int MotorSizeInHP { get { return motorSizeInHP; } set { motorSizeInHP = value; if (motorSizeInHP <= HPCUTOFF) price = LOWFEE; else price = HIGHFEE; } } public double Price { get { return price; } } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
The C# script below gives out this output:
Boat #0201601 from WI has a 30 HP motor. The price for the license is $25.00 Boat #0201602 from MI has a 50 HP motor. The price for the license is $25.00 Boat #0201603 from MN has a 100 HP motor. The price for the license is $38.00
However I want the output to look like this:
Boat #0201601 from WI has a 30 HP motor. The price for the license is $25.00 Boat #1201601 from MI has a 50 HP motor. The price for the license is $25.00 Boat #2201601 from MN has a 100 HP motor. The price for the license is $38.00
How do I change the script to get the desired output?
using System;
using static System.Console;
using System.Globalization;
class FixedDebugNine4
{
static void Main()
{
const int STARTING_NUM = 201601;
BoatLicense[] license = new BoatLicense[3];
int x;
for (x = 0; x < license.Length; ++x)
{
license[x] = new BoatLicense();
license[x].LicenseNum = (x + STARTING_NUM).ToString("D7");
}
license[0].State = "WI";
license[1].State = "MI";
license[2].State = "MN";
license[0].MotorSizeInHP = 30;
license[1].MotorSizeInHP = 50;
license[2].MotorSizeInHP = 100;
for (x = 0; x < license.Length; ++x)
Display(license[x]);
}
static void Display(BoatLicense lic)
{
WriteLine("Boat #{0} from {1} has a {2} HP motor.", lic.LicenseNum, lic.State, lic.MotorSizeInHP);
WriteLine("The price for the license is {0}\n", lic.Price.ToString("C2", CultureInfo.GetCultureInfo("en-US")));
}
}
class BoatLicense
{
public const int HPCUTOFF = 50;
public const double LOWFEE = 25.00;
public const double HIGHFEE = 38.00;
private string licenseNum;
private string state;
private int motorSizeInHP;
private double price;
public string LicenseNum
{
get { return licenseNum; }
set { licenseNum = value; }
}
public string State
{
get { return state; }
set { state = value; }
}
public int MotorSizeInHP
{
get { return motorSizeInHP; }
set
{
motorSizeInHP = value;
if (motorSizeInHP <= HPCUTOFF)
price = LOWFEE;
else
price = HIGHFEE;
}
}
public double Price
{
get { return price; }
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
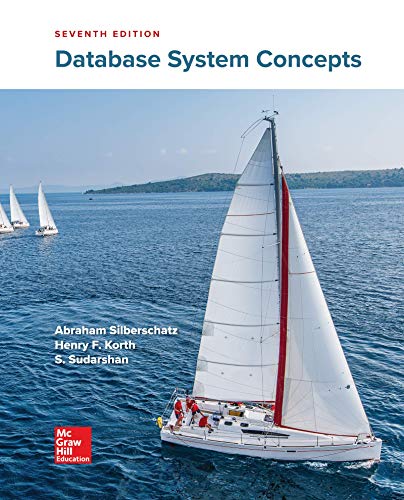
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
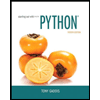
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
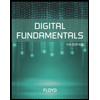
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
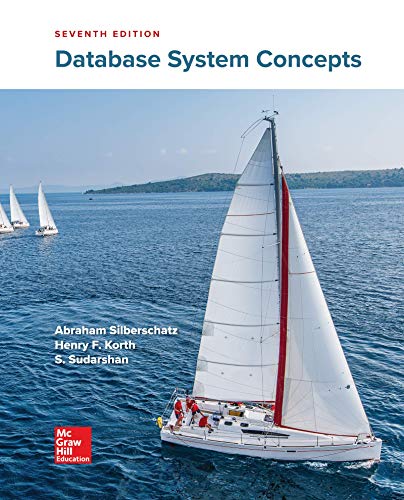
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
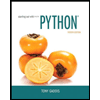
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
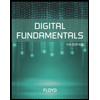
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
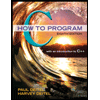
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
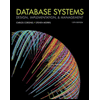
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
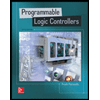
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education