the best and worst case
Please help me with this
suppose we modified the QuickSort algorithm such that we run InsertionSort on the first 10% of A in the Partition
method. You may assume the selection of the pivot will be the last element in the range
[p, r]. What would be the best and worst case running time of this new algorithm? Explain
your reasoning.
// quickSort() method for integer array
public void quickSort(int[] A, int p, int r) {
if(p < r) {
int q = partition(A, p, r);
quickSort(A, p, q - 1);
quickSort(A, q + 1, r);
}
}
// partition() method for integer array
public int partition(int[] A, int p, int r) {
int x = A[selectPivot(A, p, r)];
int i = p - 1;
for(int j = p; j < r; j++) {
if(order) {
if(A[j] > x) {
i = i + 1;
exchange(A, i, j);
}
} else {
if(A[j] <= x) {
i = i + 1;
exchange(A, i, j);
}
}
}
exchange(A, (i + 1), r);
return (i + 1);
}
// exchange() method for integer array
public void exchange(int[] A, int i, int j) {
int temp = A[i];
A[i] = A[j];
A[j] = temp;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

how would these run times differ from an unmodified quick sort? which would be more efficient?
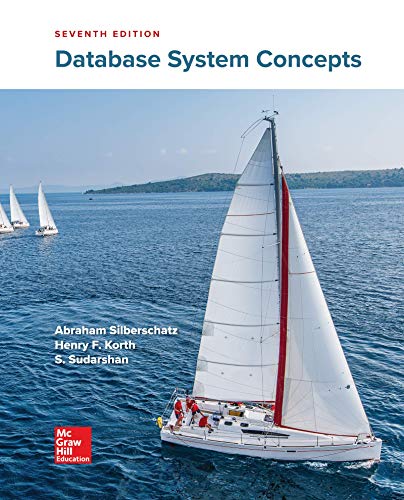
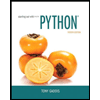
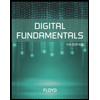
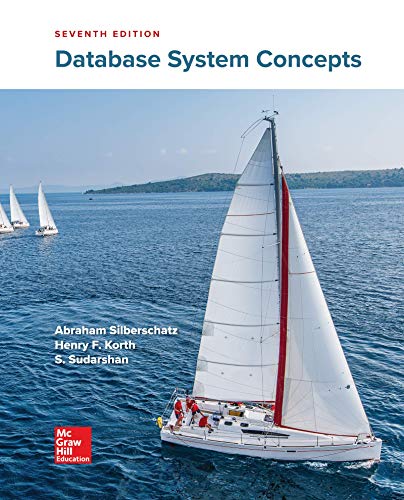
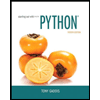
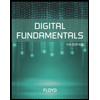
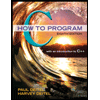
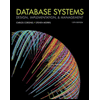
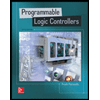