Write a method expunge that accepts a stack of integers as a parameter and makes sure that the stack's elements are in descending order from top to bottom, by removing from the stack any element that is larger than any element(s) on top of it. For example, suppose a variable s stores the following elements: bottom [4, 20, 15, 15, 8, 5, 7, 12, 8, 10, 9, 9, 16] top The element values 10, 12, 5, 8, 15, 15, and 20 should be removed because each has an element above it with a smaller value. So the call of expunge(s); should change the stack to store the following elements in this order: bottom [4, 5, 7, 8, 9, 9, 16] top
Write a method expunge that accepts a stack of integers as a parameter and makes sure that the stack's elements are in descending order from top to bottom, by removing from the stack any element that is larger than any element(s) on top of it. For example, suppose a variable s stores the following elements:
The element values 10, 12, 5, 8, 15, 15, and 20 should be removed because each has an element above it with a smaller value.
So the call of expunge(s); should change the stack to store the following elements in this order:
Notice that now the elements are in non-increasing order from top to bottom. If the stack is empty or has just one element, nothing changes. You may assume that the stack passed is not null.
Problem Requirements
-
You should solve this problem using one auxiliary Stack. You may not use other structures (arrays, lists, etc.), but you can have as many simple variables as you like.
-
You should solve this problem using the Stack/Queue methods discussed in class and use them in stack/queue like ways. You should use interface types when appropriate. See below for a list of appropriate methods.
-
The method you write should work with the provided main as written, as this is showing how we expect your method to run in our tests. You may want to edit main to test whether your code works for all expected cases, but make sure you do not change how the method is called.
-
explicitly permitted Stack/Queue methods:
Stack
-
push
-
pop
-
peek
-
size
-
isEmpty
Queue
-
add
-
remove
-
peek
-
size
-
isEmpty
This is the code:
import java.util.*;
public class StackQueueQuestion {
public static void main(String[] args) {
Stack<Integer> s = new Stack<>();
// [4, 20, 15, 15, 8, 5, 7, 12, 8, 10, 9, 9 16]
s.push(4);
s.push(20);
s.push(15);
s.push(15);
s.push(8);
s.push(5);
s.push(7);
s.push(12);
s.push(8);
s.push(10);
s.push(9);
s.push(9);
s.push(16);System.out.println("s before = " + s);
expunge(s);
System.out.println("s after = " + s);
}// TODO write your code here
}screeshot the output and the entire code plz once ur finished.
-

Java:
Java is a general purpose, high level, class based object oriented programming language.
It is simple and secure.
It is robust.
It supports multithreading.
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 4 images

thank you, but when the stack is empty, I dont wanna use return in the public static void. what else can I write
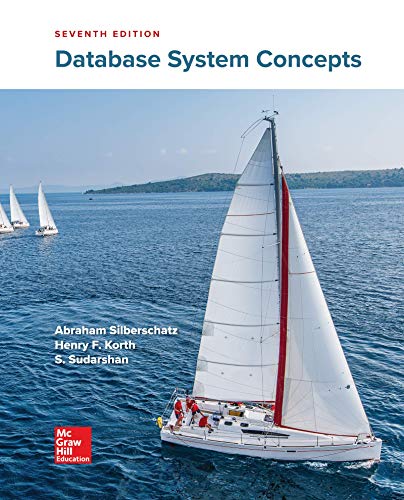
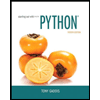
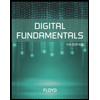
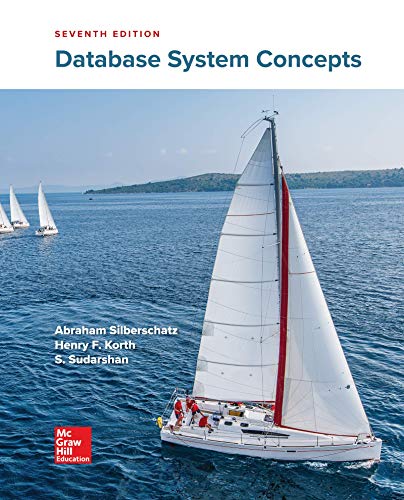
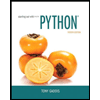
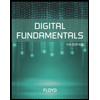
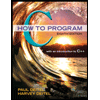
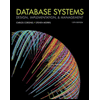
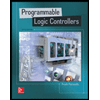