Task 4 (30 points) [Testing: Variables and expression, strings, conditional-statements, loops, functions] Function task4() shows the results of several custom string manipulation functions you will write. For this task, you need to define the following three functions: countCases (param: string) returns two integer values This function takes a string and returns two values. The first value is the number of uppercase letters in the string. The second value is the number of lowercase letters in the string. For example: "EECS1015 Fal1 2021" has 5 uppercase (red) and 3 lowercase (green). flipCase(param: string) returns a string This function takes a string and returns a new string where the cases of the string are swapped. For example: "EECS1015 Fall 2021" would be converted to "eecs1015 FALL 2021" cutQuotedText(param: string) returns a string This function takes a string with a single "word" in douubles quotes and removes aIl characters within the
Task 4 (30 points) [Testing: Variables and expression, strings, conditional-statements, loops, functions] Function task4() shows the results of several custom string manipulation functions you will write. For this task, you need to define the following three functions: countCases (param: string) returns two integer values This function takes a string and returns two values. The first value is the number of uppercase letters in the string. The second value is the number of lowercase letters in the string. For example: "EECS1015 Fal1 2021" has 5 uppercase (red) and 3 lowercase (green). flipCase(param: string) returns a string This function takes a string and returns a new string where the cases of the string are swapped. For example: "EECS1015 Fall 2021" would be converted to "eecs1015 FALL 2021" cutQuotedText(param: string) returns a string This function takes a string with a single "word" in douubles quotes and removes aIl characters within the
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter6: User-defined Functions
Section: Chapter Questions
Problem 23PE
Related questions
Question
![Task 4 (30 points) [Testing: Variables and expression, strings, conditional-statements, loops, functions]
Function task4() shows the results of several custom string manipulation functions you will write. For this
task, you need to define the following three functions:
countCases (param: string) returns two integer values
This function takes a string and returns two values. The first value is the number of uppercase letters in the
string. The second value is the number of lowercase letters in the string.
For example: "EECS1015 Fall 2021" has 5 uppercase (red) and 3 lowercase (green).
flipCase(param: string) returns a string
This function takes a string and returns a new string where the cases of the string are swapped.
For example: "EECS1015 Fall 2021" would be converted to "eecs1015 FALL 2021"
cutQuotedText(param: string) returns a string
This function takes a string with a single "word" in doubles quotes and removes all characters within the
quote, including the quotes. To simplify things, we will assume that only two " characters appear in the
string. If exactly two quote characters are not in the string, we will return a failure string message as shown
below:
For example (proper input):
Input to function: 'I'm taking "EECS1015" this semester.'
Return string:'I'm taking this semester."
<- quoted text has been removed.
If there isn't exactly one quoted text (i.e., only two quotes), return 'ERROR! No quoted text.'
For example (failure input):
Input to function: 'EECS1015'
Return string: 'ERROR! No quoted text.'
Another failure example:
Input to function: 'This "is" a test".'
Return string: 'ERROR! No quoted text.
<- considered a failure because more than two "
Using the three functions above, implement task4() function as follows:
(1) Prompt the user to enter a long string with one quoted word.
(2) Pass string to countCases() function.
(3) Pass string to flipCase() function.
(4) Pass string to cutQuotedText() function.
(5) Print out the results of the functions as shown below.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbeb43acd-4bfb-4c49-84a8-7d6db7b72498%2Fec221f4d-285c-4f88-b19c-a44aed516f41%2Fldt8i5n_processed.png&w=3840&q=75)
Transcribed Image Text:Task 4 (30 points) [Testing: Variables and expression, strings, conditional-statements, loops, functions]
Function task4() shows the results of several custom string manipulation functions you will write. For this
task, you need to define the following three functions:
countCases (param: string) returns two integer values
This function takes a string and returns two values. The first value is the number of uppercase letters in the
string. The second value is the number of lowercase letters in the string.
For example: "EECS1015 Fall 2021" has 5 uppercase (red) and 3 lowercase (green).
flipCase(param: string) returns a string
This function takes a string and returns a new string where the cases of the string are swapped.
For example: "EECS1015 Fall 2021" would be converted to "eecs1015 FALL 2021"
cutQuotedText(param: string) returns a string
This function takes a string with a single "word" in doubles quotes and removes all characters within the
quote, including the quotes. To simplify things, we will assume that only two " characters appear in the
string. If exactly two quote characters are not in the string, we will return a failure string message as shown
below:
For example (proper input):
Input to function: 'I'm taking "EECS1015" this semester.'
Return string:'I'm taking this semester."
<- quoted text has been removed.
If there isn't exactly one quoted text (i.e., only two quotes), return 'ERROR! No quoted text.'
For example (failure input):
Input to function: 'EECS1015'
Return string: 'ERROR! No quoted text.'
Another failure example:
Input to function: 'This "is" a test".'
Return string: 'ERROR! No quoted text.
<- considered a failure because more than two "
Using the three functions above, implement task4() function as follows:
(1) Prompt the user to enter a long string with one quoted word.
(2) Pass string to countCases() function.
(3) Pass string to flipCase() function.
(4) Pass string to cutQuotedText() function.
(5) Print out the results of the functions as shown below.

Transcribed Image Text:-------Task 4-
Enter string with one word with "quotes": This is a "test" input.
This string has 1 uppercase characters.
This string has 15 lowercase characters.
Case flip: 'THIS IS A "TEST" INPUT.'
Quote removed: 'This is a
Print case flip and quote removed results
with single quotes around the strings.
input.'
(more examples of task 4 on next page)
Page 8/12
----Task 4------
Enter string with one word with "quotes": Another "example" of good input.
This string has 1 uppercase characters.
This string has 24 lowercase characters.
Case flip: 'ANOTHER "EXAMPLE" OF GOOD INPUT. '
Quote removed: 'Another
of good input.
-Task 4-
Enter string with one word with "quotes": Example with BAD input.
This string has 4 uppercase characters.
This string has 15 lowercase characters.
Case flip: 'EXAMPLE WITH bad INPUT.
Quote removed: 'ERROR! No quoted text.
--Task 4-
Enter string with one word with "quotes" Example with "more" than two " chars.
This string has 1 upper case characters
This string has 26 lower case characters
Case flip: 'EXAMPLE WITH "MORE" THAN TWO
Quote removed: 'ERROR! No quoted text.'
CHARS.'
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
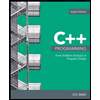
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
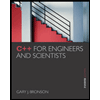
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
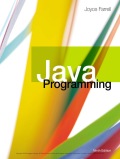
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
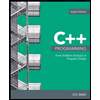
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
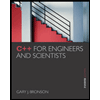
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
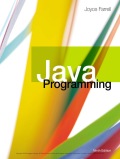
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
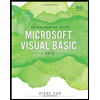
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
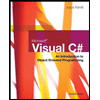
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,