Task 1. Write a C program that asks the user to select the command that needs to be performed. The commands that need to be supported are listed in the following table: command q I a b с AB+ +* t PED Р m d operation quit program create matrix A equal to the identity matrix print matrix A print matrix B print matrix C create matrix A copy matrix A to matrix B add matrix A to matrix B, placing the result in matrix C transpose matrix A multiply matrix A and B, placing the result in matrix C print matrixA elements in reverse order compute a minor of matrix A, placing the result in matrix C compute the determinant of matrix A In this task you only need to implement the quit command. In later tasks you will implement the remainder. Hint: make a loop in which you ask for a single character command, like this: char cmd; do { printf ("Command? "); scanf("%c",&cmd); // notice the space before the % } while (cmd != 'q');
Task 1. Write a C program that asks the user to select the command that needs to be performed. The commands that need to be supported are listed in the following table: command q I a b с AB+ +* t PED Р m d operation quit program create matrix A equal to the identity matrix print matrix A print matrix B print matrix C create matrix A copy matrix A to matrix B add matrix A to matrix B, placing the result in matrix C transpose matrix A multiply matrix A and B, placing the result in matrix C print matrixA elements in reverse order compute a minor of matrix A, placing the result in matrix C compute the determinant of matrix A In this task you only need to implement the quit command. In later tasks you will implement the remainder. Hint: make a loop in which you ask for a single character command, like this: char cmd; do { printf ("Command? "); scanf("%c",&cmd); // notice the space before the % } while (cmd != 'q');
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
100%

Transcribed Image Text:Task 1. Write a C program that asks the user to select the command that needs to be performed. The
commands that need to be supported are listed in the following table:
command operation
a
Am ++ * PET
Р
m
d
quit program
create matrix A equal to the identity matrix
print matrix A
print matrix B
print matrix C
create matrix A
copy matrix A to matrix B
add matrix A to matrix B, placing the result in matrix C
transpose matrix A
multiply matrix A and B, placing the result in matrix C
print matrixA elements in reverse order
compute a minor of matrix A, placing the result in matrix C
compute the determinant of matrix A
In this task you only need to implement the quit command. In later tasks you will implement the remainder.
Hint: make a loop in which you ask for a single character command, like this:
char cmd;
do {
printf ("Command? ");
scanf("%c",&cmd); // notice the space before the %
} while (cmd != 'q');
![Task 2. Define three two-dimensional matrices (matrixA, matrixB, matrixC) in your main function of
MAXSIZE by MAXSIZE float elements and initialise all elements to zero. You also need to define the integer
variables rows A, columnsA, rowsB, columnsB, rowsC, columnsC that indicate the size of each matrix. They
are initialised to 1.
Write a print function that prints a matrix of rows rows and columns columns. It prints the floating point
numbers of each matrix row on one line, separated by a single space. The formatting of the float is a total
of 7 positions with an accuracy of 3 positions. The name character is the name of the array. Use the letter 'a'
for the command to print matrixA using this function.
#define MAXSIZE 10
void print (float matrix [MAXSIZE] [MAXSIZE], int rows, int columns, char name) { ...
int main (void) {
print (matrixA, rowsA, columnsA, 'A');
}
A 3x3 matrix would be formatted like this:
Command? a
Matrix A (3 X 3):
1.000 0.000 0.000
0.000 1.000 0.000
0.000 0.000 1.000
Since the matrix matrixA has been initialised to a size of 1x1 with value 0 this should be the result of your
program:
Command? a
Matrix A (1 X 1):
0.000
Command? q
Bye!
138%](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F0b36f4fe-6962-4315-bbea-02248ca00e23%2F3294027d-5aef-4b42-a8ba-31b9815b5057%2Fqvnzn2_processed.png&w=3840&q=75)
Transcribed Image Text:Task 2. Define three two-dimensional matrices (matrixA, matrixB, matrixC) in your main function of
MAXSIZE by MAXSIZE float elements and initialise all elements to zero. You also need to define the integer
variables rows A, columnsA, rowsB, columnsB, rowsC, columnsC that indicate the size of each matrix. They
are initialised to 1.
Write a print function that prints a matrix of rows rows and columns columns. It prints the floating point
numbers of each matrix row on one line, separated by a single space. The formatting of the float is a total
of 7 positions with an accuracy of 3 positions. The name character is the name of the array. Use the letter 'a'
for the command to print matrixA using this function.
#define MAXSIZE 10
void print (float matrix [MAXSIZE] [MAXSIZE], int rows, int columns, char name) { ...
int main (void) {
print (matrixA, rowsA, columnsA, 'A');
}
A 3x3 matrix would be formatted like this:
Command? a
Matrix A (3 X 3):
1.000 0.000 0.000
0.000 1.000 0.000
0.000 0.000 1.000
Since the matrix matrixA has been initialised to a size of 1x1 with value 0 this should be the result of your
program:
Command? a
Matrix A (1 X 1):
0.000
Command? q
Bye!
138%
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
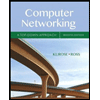
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
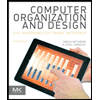
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
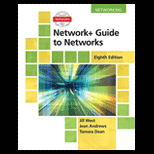
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
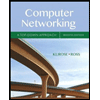
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
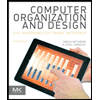
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
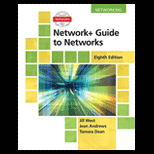
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
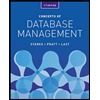
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
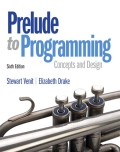
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
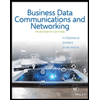
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY