Function 1: Password Checker function _one(pwd) Create a JavaScript function that meets the following requirements: • Is passed a string representing a potential password • The function determines if the password meets the following requirements o The length of the password is between 8 – 12 characters o Contains at least 1 number (numeric character) o Contains at least 1 special character ▪ Special characters are punctuation characters that are present on a standard keyboard namely, any character encapsulated within the following double quotes “(!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~)” o Contains at least 1 capital letter • The function should NOT employ the use of regular expressions to solve the problem • The function also displays the result (console log) of its password validation as illustrated below. • The function returns a Boolean back to the caller indicating valid or not.
Function 1: Password Checker
function _one(pwd)
Create a JavaScript function that meets the following requirements:
• Is passed a string representing a potential password
• The function determines if the password meets the following requirements
o The length of the password is between 8 – 12 characters
o Contains at least 1 number (numeric character)
o Contains at least 1 special character
▪ Special characters are punctuation characters that are present on a standard keyboard
namely, any character encapsulated within the following double quotes
“(!"#$%&'()*+,-./:;<=>?@[\]^_`{|}~)”
o Contains at least 1 capital letter
• The function should NOT employ the use of regular expressions to solve the problem
• The function also displays the result (console log) of its password validation as illustrated below.
• The function returns a Boolean back to the caller indicating valid or not.
Function 2: Calculate Remainder
function _two(dividend, divisor)
Create a JavaScript function that meets the following requirements:
• Is passed two integer parameters, one representing a dividend, and the other a divisor.
• The function is NOT permitted to use the % (mod) operator
• The function can use any combination of +, -, * or / to solve the problem
• The function displays the calculated remainder (console log) as illustrated below:
• The function returns the result of the calculation back to the caller.
Function 3: Volume of Cylinder
function _three(radius, height)
Create a JavaScript function that meets the following requirements:
• Receives two parameters, one representing a radius value, the other a height value
o The function can safely assume, all parameters passed are represented in meters (m).
• The function calculates the volume of a cylinder and returns the value back to its caller.
• The function always displays the calculated volume, rounded to two significant digits
• The function displays the calculated information (console log) as illustrated below:
• The function returns the calculated volume back to the caller
function _four( array )
Create a JavaScript function that meets the following requirements:
• Receives a variable-length array of numbers, representing student grades in a course
• The function traverses the array to determine the number of passing and failing grades.
o A passing grade, is a grade greater than or equal to 50
• The function displays the count (console log) for the number of passing grades, failing grades, and overall class average as illustrated below
• Average class grade is rounded to 1 decimal point.
The function returns the array containing the number of passing grades, the number of failing grades, and the overall averages of the grades, respectively, back to the caller
Function 5: Count Occurrences
function _five(text, pattern)
Create a JavaScript function that meets the following requirements:
• Accepts two string parameters, a principal string, and pattern string.
• The function determines if the pattern string exists within the principal string.
• The function displays the result (console log) as illustrated below.
• The function returns a Boolean back to the caller, indicating if the pattern was found.
Note:
Please ensure to remove all instances of the following from your final submission solution:
• document.write()
• innerHTML()
• alert()
• any commented code
• Do not overuse the console log, spamming the console log unnecessarily, say for example.
with debug-related output may cost you marks.

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

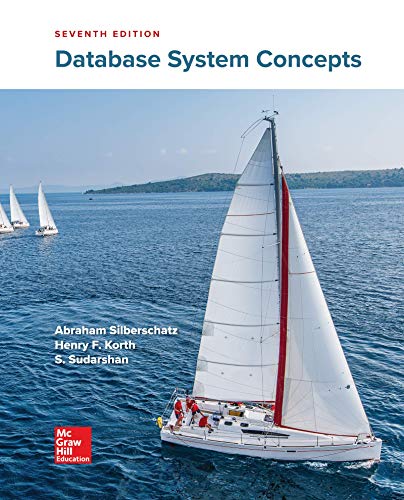
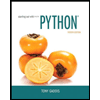
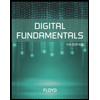
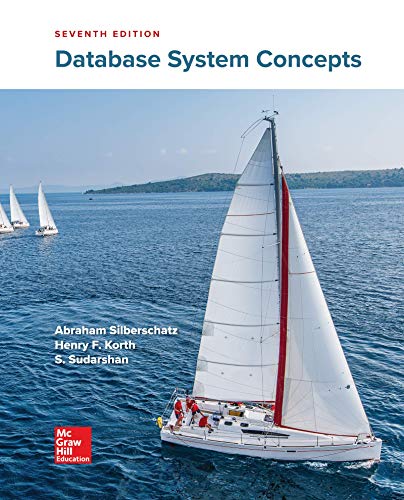
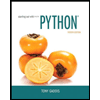
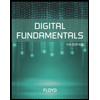
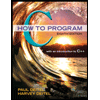
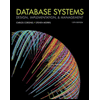
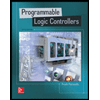