Take the following partially completed RoundRobin class that is Iterable, and supplies an Iterator to clients via the iterator() method. Finish the Iterator nested class such that a collection of iterators (any number) can be traversed and accessed in “round robin” sequence. That is, your iterator produces one value from each iterator in the list of iterators before cycling back to the beginning of the list. It should proceed until all iterators in the given list are exhausted. Not all iterators in the list necessarily have the same number of elements; if an iterator is exhausted, but others have elements remaining, it is skipped in the rotation. See the code in main() below for example usage and output. You may throw any exceptions you deem needed. import java.util.ArrayList; import java.util.Iterator; public class RoundRobin implements Iterable { private List> collectionOfIterators; private int index = 0; public RoundRobin(List> collectionOfIterators) { this.collectionOfIterators = collectionOfIterators; } public Iterator iterator() { return new Iterator() { @Override public boolean hasNext() { // YOU WRITE THIS! } @Override public T next() { // YOU WRITE THIS! } }; } public static void main(String [] args) { ArrayList names = new ArrayList(); names.add("Wanda Bids: "); names.add("Vision Bids: "); names.add("Quicksilver Bids: "); names.add("Captain Marvel Bids: "); TriangleSeries ts = new TriangleSeries(2,6); Iterator tsi = ts.iterator(); ArrayList bids = new ArrayList<>(); while(tsi.hasNext()) { bids.add("$ " + Integer.toString(tsi.next())); } ArrayList winner = new ArrayList(); winner.add("Wanda has winning bid"); winner.add("Vision has winning bid"); winner.add("Quicksilver has winning bid"); winner.add("Captain Marvel has winning bid"); winner.add("What about Thanos?"); winner.add("I SAID: What about Thanos???"); ArrayList> it = new ArrayList<>(); it.add(names.iterator()); it.add(bids.iterator()); it.add(winner.iterator()); RoundRobin robin = new RoundRobin(it); Iterator roundRobinIterator = robin.iterator(); while(roundRobinIterator.hasNext()) { System.out.println(roundRobinIterator.next()); } } }
Take the following partially completed RoundRobin class that is Iterable, and supplies an Iterator to clients via the iterator() method. Finish the Iterator<T> nested class such that a collection of iterators (any number) can be traversed and accessed in “round robin” sequence. That is, your iterator produces one value from each iterator in the list of iterators before cycling back to the beginning of the list. It should proceed until all iterators in the given list are exhausted. Not all iterators in the list necessarily have the same number of elements; if an iterator is exhausted, but others have elements remaining, it is skipped in the rotation. See the code in main() below for example usage and output. You may throw any exceptions you deem needed.
import java.util.ArrayList;
import java.util.Iterator;
public class RoundRobin<T> implements Iterable<T> {
private List<Iterator<T>> collectionOfIterators;
private int index = 0;
public RoundRobin(List<Iterator<T>> collectionOfIterators) {
this.collectionOfIterators = collectionOfIterators;
}
public Iterator<T> iterator() {
return new Iterator<T>() {
@Override
public boolean hasNext() {
// YOU WRITE THIS!
}
@Override
public T next() {
// YOU WRITE THIS!
}
};
}
public static void main(String [] args) {
ArrayList<String> names = new ArrayList<String>();
names.add("Wanda Bids: ");
names.add("Vision Bids: ");
names.add("Quicksilver Bids: ");
names.add("Captain Marvel Bids: ");
TriangleSeries ts = new TriangleSeries(2,6);
Iterator<Integer> tsi = ts.iterator();
ArrayList<String> bids = new ArrayList<>();
while(tsi.hasNext()) {
bids.add("$ " + Integer.toString(tsi.next()));
}
ArrayList<String> winner = new ArrayList<String>();
winner.add("Wanda has winning bid");
winner.add("Vision has winning bid");
winner.add("Quicksilver has winning bid");
winner.add("Captain Marvel has winning bid");
winner.add("What about Thanos?");
winner.add("I SAID: What about Thanos???");
ArrayList<Iterator<String>> it = new ArrayList<>();
it.add(names.iterator());
it.add(bids.iterator());
it.add(winner.iterator());
RoundRobin robin = new RoundRobin(it);
Iterator<String> roundRobinIterator = robin.iterator();
while(roundRobinIterator.hasNext()) {
System.out.println(roundRobinIterator.next());
}
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

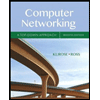
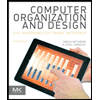
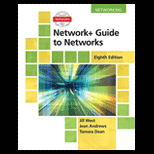
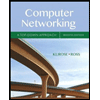
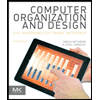
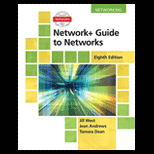
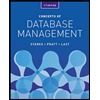
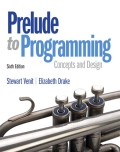
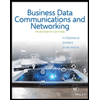