Table 1 Execution Time Arrival Time 3 unit time Task ID T1 to T2 T3 5 unit time t1 2 unit time t3 T4 T5 T6 4 unit time 6 unit time 1 unit time to t10 t14 The timeline of printing job arrival time for Table 1 is illustrated in Figure 1 (up until the last job arrived). Arrival Time to t t2 T1 T2 t3 t4 ts | t6 t7 ts T3 to t10 t11 t12 t13 t14 T5 T4 T6 Figure 1
can you add based on code below to prints the time when the print job from each student started and their waiting time. It also need to calculate the time needed to complete all printing jobs and the average waiting time. all using dynamic queue.
#include <bits/stdc++.h>
using namespace std;
struct QNode {
int data;
QNode* next;
QNode(int d)
{
data = d;
next = NULL;
}
};
struct Queue {
QNode *front, *rear;
Queue()
{
front = rear = NULL;
}
void enQueue(int x)
{
// Create a new LL node
QNode* temp = new QNode(x);
// If queue is empty, then
// new node is front and rear both
if (rear == NULL) {
front = rear = temp;
return;
}
// Add the new node at
// the end of queue and change rear
rear->next = temp;
rear = temp;
}
// Function to remove
// a key from given queue q
void deQueue()
{
// If queue is empty, return NULL.
if (front == NULL)
return;
// Store previous front and
// move front one node ahead
QNode* temp = front;
front = front->next;
// If front becomes NULL, then
// change rear also as NULL
if (front == NULL)
rear = NULL;
delete (temp);
}
};
// Driven Program
int main()
{
Queue q;
q.enQueue(10);
q.enQueue(20);
q.deQueue();
q.deQueue();
q.enQueue(30);
q.enQueue(40);
q.enQueue(50);
q.deQueue();
cout << "Queue Front : " << (q.front)->data << endl;
cout << "Queue Rear : " << (q.rear)->data;
}


Step by step
Solved in 2 steps with 1 images

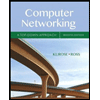
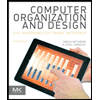
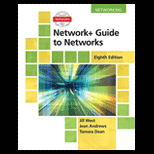
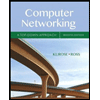
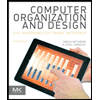
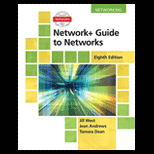
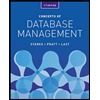
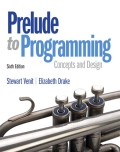
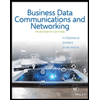