surve in your ive been made available. Each contains data for one household. A single record includes an integer identification number, the annual income for the household, and the number of members of the household. You may assume that no more than 25 households were surveyed. Write a program to store the survey results into an array of user-defined structures of type household. The program should input the survey results from the user._The program should also perform the following operations: (Your program must provide a function for each of the following operations) • Calculate the average household income, and list the identification number and income of each household whose income exceeds the average. • Calculate the percentage of households having incomes below the poverty level. The poverty level, P, may be computed using the formula P = 2000 + 1000 • (m – 2) where m is the number of members in the household. Sample Input 24 5000 4 36 10000 5 45 2000 4 50 4000 2 55 6000 4


Since you are not mentioning the programming language, here we are using C++ to complete the program.
PROGRAM:
//Header files
#include <iostream>
#include <iomanip>
#include<stdio.h>
//Using namespace
using namespace std;
//Defining average()
void average(int id[],int annual_income[],int household_number[],int count)
{
int total=0;
for(int i=0;i<count;i++)
{
//Finding the total income
total=total+annual_income[i];
}
//Finding the average
cout<<"\n"<<total/count<<endl;
for(int i=0;i<count;i++)
{
if(annual_income[i]>total/count)
{
//Finding the highest average id and income
cout<<id[i]<<" "<<annual_income[i]<<endl;
}
}
}
//Defining the percentage_hse() for poverty
void percentage_hse(int annual_income[],int household_number[],int count)
{
int next=0;
for(int i=0;i<count;i++)
{
//Computing poverty level
int p=2000+1000*(household_number[i]-2);
//Comparing with incomes
if(annual_income[i]<p)
next+=1;
}
//Computing the percentage
float percentage=(float)next/(float)count;
//Printing the percentage
cout<<fixed << setprecision(2) <<percentage;
}
//Defining the main()
int main()
{
//Declaring array to store the survey details
int id[100],annual_income[100],household_number[100];
//Declaring the variables
int id_no,income,hse_no,count=0,total;
//Getting total survey count
cout<<"Enter number of surveys: ";
cin>>total;
//Getting survey details
while(total!=0)
{
cin>>id_no>>income>>hse_no;
id[count]=id_no;
annual_income[count]=income;
household_number[count]=hse_no;
count++;
total-=1;
}
//Calling the functions
average(id,annual_income,household_number,count);
percentage_hse(annual_income,household_number,count);
}
Step by step
Solved in 2 steps with 1 images

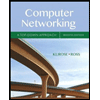
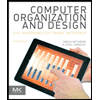
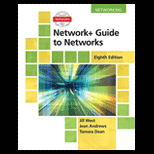
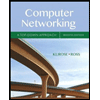
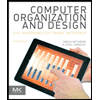
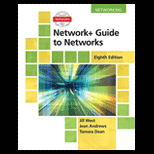
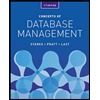
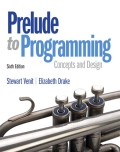
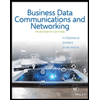