Create an algorithm for the following code: from turtle import * # Set up the glossary glossary = {'word1':'definition1', 'word2':'definition2', 'word3':'definition3'} def show_flashcard(): """ Show the user a random key and ask them to define it. Show the definition when the user presses return. """ random_key = choice(list(glossary)) print('Define: ', random_key) input('Press return to see the definition') print(glossary[random_key]) # Uncomment the next line and then complete the function for part aii. def look_up_definition(): word=input('Please enter your word: ') try: wdef=glossary[word] print(wdef) except KeyError: print('%s not found in glossary'%word) # The interactive loop exit = False while not exit: user_input = input('Enter s to show a flashcard and q to quit: ') if user_input == 'q': exit = True elif user_input == 's': show_flashcard() elif user_input == 'd': look_up_definition() else: print('You need to enter either q or s.')
Create an
from turtle import *
# Set up the glossary
glossary = {'word1':'definition1',
'word2':'definition2',
'word3':'definition3'}
def show_flashcard():
""" Show the user a random key and ask them
to define it. Show the definition
when the user presses return.
"""
random_key = choice(list(glossary))
print('Define: ', random_key)
input('Press return to see the definition')
print(glossary[random_key])
# Uncomment the next line and then complete the function for part aii.
def look_up_definition():
word=input('Please enter your word: ')
try:
wdef=glossary[word]
print(wdef)
except KeyError:
print('%s not found in glossary'%word)
# The interactive loop
exit = False
while not exit:
user_input = input('Enter s to show a flashcard and q to quit: ')
if user_input == 'q':
exit = True
elif user_input == 's':
show_flashcard()
elif user_input == 'd':
look_up_definition()
else:
print('You need to enter either q or s.')

Step by step
Solved in 2 steps

Suggest one further small extension or improvement of your own to the modified flashcard program. Outline what the extension does and briefly say what additional sub-problem(s) would need to be added to the initial decomposition.
Note that you are only required to describe your further extension and do not need to implement it in code.
The maximum word count is 150 words, including the added sub-problem(s).
-
Discuss briefly how you will test the
program. Only a short answer is required, and you do not need to give examples, only describe what approach you will follow.
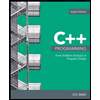
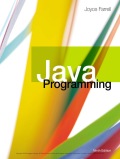
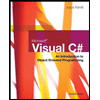
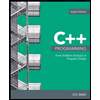
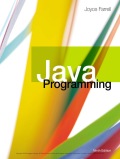
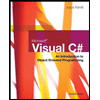
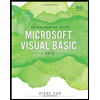