String widthDataFile is read from input. The try block opens a file named widthDataFile for output. Two floating-point numbers are read from input, and each floating-point number is written into the file. Write the finally block to perform the following tasks, if the output file is open: 1. Write to the file "Number of input values read: ", followed by numValues Read and a newline. 2. If numValuesRead is greater than zero, write to the file "Last value is: ", followed by widthLast and a newline. 3. Close the file. Ex: If the input is width.txt 12.0 11.0, then width.txt contains: 12.0 11.0 Number of input values read: 2 Last value is: 11.0 Ex: If the input is widthFile.txt invalid 11.0, then widthFile.txt contains: Number of input values read: 0 Ex: If the input is /nowhere.txt, then the output is: Error! Note: Data output to a file may be lost if the file is not closed. 1 import java.util.Scanner; 2 import java.io.FileOutputStream; 3 import java.io.Printwriter; 4 5 public class WriteLastWidthFile { 6 7 8 9 10 11 12 13 14 15 16 17 public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Printwriter foutWriter = null; String widthDataFile; double valueRead; int numValues Read = 0; double widthLast = 0.0; widthDataFile = scnr.next(); try { foutWriter = new Printwriter(new FileOutputStream(widthDataFile));
String widthDataFile is read from input. The try block opens a file named widthDataFile for output. Two floating-point numbers are read from input, and each floating-point number is written into the file. Write the finally block to perform the following tasks, if the output file is open: 1. Write to the file "Number of input values read: ", followed by numValues Read and a newline. 2. If numValuesRead is greater than zero, write to the file "Last value is: ", followed by widthLast and a newline. 3. Close the file. Ex: If the input is width.txt 12.0 11.0, then width.txt contains: 12.0 11.0 Number of input values read: 2 Last value is: 11.0 Ex: If the input is widthFile.txt invalid 11.0, then widthFile.txt contains: Number of input values read: 0 Ex: If the input is /nowhere.txt, then the output is: Error! Note: Data output to a file may be lost if the file is not closed. 1 import java.util.Scanner; 2 import java.io.FileOutputStream; 3 import java.io.Printwriter; 4 5 public class WriteLastWidthFile { 6 7 8 9 10 11 12 13 14 15 16 17 public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Printwriter foutWriter = null; String widthDataFile; double valueRead; int numValues Read = 0; double widthLast = 0.0; widthDataFile = scnr.next(); try { foutWriter = new Printwriter(new FileOutputStream(widthDataFile));
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
New JAVA code can only be added between lines 27 and 29. As seen in image.

Transcribed Image Text:**Handling File Input and Output in Java**
This tutorial demonstrates how to read from an input file and write to an output file using Java. The process involves reading floating-point numbers from a specified input file, processing them, and writing the results to an output file.
### Code Overview
1. **Imports**:
- `java.util.Scanner`: For scanning input from the user.
- `java.io.FileOutputStream` and `java.io.PrintWriter`: For writing to a file.
2. **Main Class and Method**:
- The class `WriteLastWidthFile` contains the `main` method. A `Scanner` object is used to read from `System.in`.
- The input file name is read as a string from the user.
- A `PrintWriter` is used to write to the output file.
3. **Variables**:
- `widthDataFile`: A string representing the file name.
- `numValuesRead`: An integer counting the number of valid inputs read.
- `widthLast`: A double to store the last valid width value read.
4. **Workflow**:
- Attempt to open the specified file for writing within a `try` block.
- Read two floating-point numbers from the input.
- Write these numbers to the output file.
- In the `finally` block, the following tasks are executed:
1. Write "Number of input values read:", followed by the count.
2. If there are valid inputs, write the last value.
3. Close the file to ensure data is saved.
5. **Examples**:
- If the input from `width.txt` is valid (e.g., `12.0 11.0`), the output file will contain:
```
12.0
11.0
Number of input values read: 2
Last value is: 11.0
```
- If there is invalid input (e.g., `invalid 11.0`), it will contain:
```
Number of input values read: 0
```
- If the input file path is incorrect (e.g., `/nowhere.txt`), the output will display an error message.
6. **Note**:
- Always close the file output stream to prevent data loss.
This example illustrates basic file handling operations in Java, including reading input

Transcribed Image Text:The image shows a section of Java code designed for reading and writing data. Below is the transcription of the code snippet:
```java
try {
fOutWriter = new PrintWriter(new FileOutputStream(widthDataFile));
for (numValuesRead = 0; numValuesRead < 2; ++numValuesRead) {
valueRead = scnr.nextDouble();
fOutWriter.println(valueRead);
widthLast = valueRead;
}
}
catch (Exception e) {
System.out.println("Error!");
}
/* Your code goes here */
```
### Code Explanation:
- **Lines 16-25 (Try Block):**
- A `PrintWriter` is instantiated with a `FileOutputStream` for `widthDataFile`. This setup is meant for writing data to a file.
- A `for` loop runs, intended to read two double values from an input source (`scnr` assumed to be a `Scanner`).
- Each value read is printed to the output file and stored in the variable `widthLast`.
- **Lines 26-29 (Catch Block):**
- Catches any `Exception` that might occur in the try block and prints "Error!" to the console, serving as basic error handling.
- **Line 28 (Comment):**
- Contains a comment placeholder where additional code can be added.
This snippet highlights file writing operations and control structures in Java, commonly used for managing data input and output efficiently.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
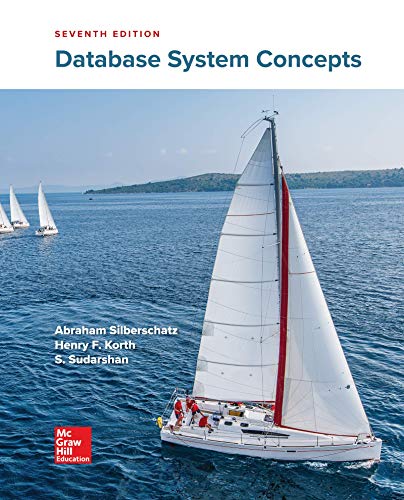
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
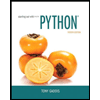
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
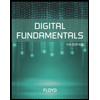
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
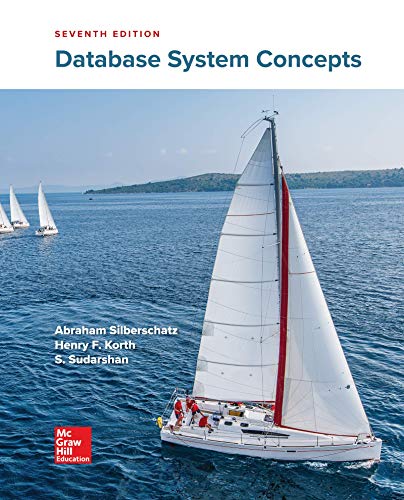
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
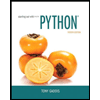
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
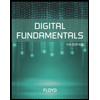
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
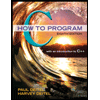
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
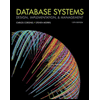
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
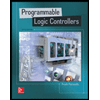
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education