Steps to be followed: Create a class called MyThread that extends the Thread class. Declare an instance variable int limitToStop in the MyThread class. Create a parameterized constructor in the MyThread class that takes an int as a parameter to initialize a limitToStop instance variable. Override the run() method in the MyThread class. In the run() method, put a for-loop that goes from 0 to limitToStop. The block associated with the for-loop should print out only the odd numbers. Call Thread.sleep(1000) after every number you print out in the for-loop you created in the previous step. The Thread.sleep(1000) method throws an InterruptedException , which is a checked exception. Therefore, we need to enclose the Thread.sleep(1000) call in a try block. Also, add a catch block associated with the try that catches the InterruptedException and prints out the exception information using the printStackTrace() method. Create a MyThread variable using the new keyword in the main() method of the Main class. Pass 20 to the parameterized constructor when creating your variable. Finally, start() the thread created in the previous step.
Steps to be followed:
-
Create a class called MyThread that extends the Thread class.
-
Declare an instance variable int limitToStop in the MyThread class.
-
Create a parameterized constructor in the MyThread class that takes an int as a parameter to initialize a limitToStop instance variable.
-
Override the run() method in the MyThread class.
-
In the run() method, put a for-loop that goes from 0 to limitToStop. The block associated with the for-loop should print out only the odd numbers.
-
Call Thread.sleep(1000) after every number you print out in the for-loop you created in the previous step.
-
The Thread.sleep(1000) method throws an InterruptedException , which is a checked exception. Therefore, we need to enclose the Thread.sleep(1000) call in a try block. Also, add a catch block associated with the try that catches the InterruptedException and prints out the exception information using the printStackTrace() method.
-
Create a MyThread variable using the new keyword in the main() method of the Main class. Pass 20 to the parameterized constructor when creating your variable.
-
Finally, start() the thread created in the previous step.

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

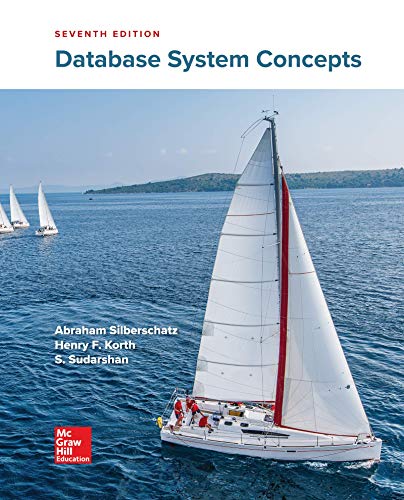
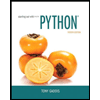
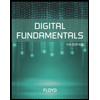
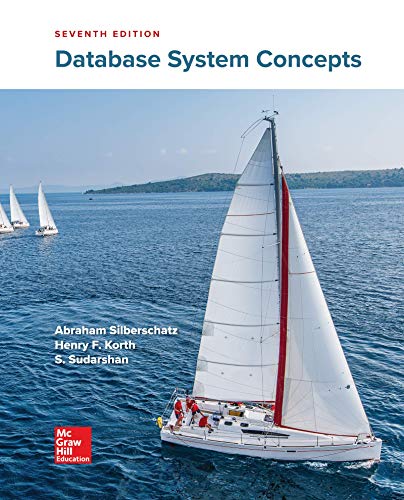
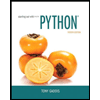
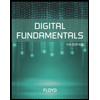
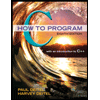
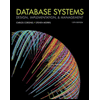
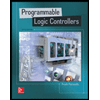