std; const int ROWS = 3; const int COLS = 3; const int MIN = 1; const int MAX = 9; bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size); bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max); bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int siz
C++, create flowchart for this code:
#include <iostream>
using namespace std;
const int ROWS = 3;
const int COLS = 3;
const int MIN = 1;
const int MAX = 9;
bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max);
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
bool checkDiagSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size);
int main(){
int arrayRow1[ROWS], arrayRow2[ROWS], arrayRow3[ROWS];
char ch;
do{
fillArray(arrayRow1, arrayRow2, arrayRow3, ROWS);
showArray(arrayRow1, arrayRow2, arrayRow3, ROWS);
if(!isMagicSquare(arrayRow1, arrayRow2, arrayRow3, ROWS))
cout<<"This is not a Lo Shu magic square\n";
else
cout<<"This is a Lo Shu magic square\n";
cout<<"\n\nDo you want to try again?"; cin>>ch;
}while(ch=='y'||ch=='Y');
}
bool isMagicSquare(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
if(!checkRange(arrayRow1, arrayRow2, arrayRow3, ROWS, 1, 9))
return false;
else if(!checkUnique(arrayRow1, arrayRow2, arrayRow3, ROWS))
return false;
else if(!checkRowSum(arrayRow1, arrayRow2, arrayRow3, ROWS))
return false;
else if(!checkColSum(arrayRow1, arrayRow2, arrayRow3, ROWS))
return false;
else if(!checkDiagSum(arrayRow1, arrayRow2, arrayRow3, ROWS))
return false;
return true;
}
bool checkRange(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size, int min, int max){
for(int i = 0; i<size; i++){
if(arrayRow1[i]<min || arrayRow1[i]>max)
return false;
else if(arrayRow2[i]<min || arrayRow2[i]>max)
return false;
else if(arrayRow3[i]<min || arrayRow3[i]>max)
return false;
}
return true;
}
bool checkUnique(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
int list[] = {0,0,0,0,0,0,0,0,0};
for(int i = 0; i<size; i++){
if(list[arrayRow1[i]] == 1)
return false;
else
list[arrayRow1[i]] = 1;
if(list[arrayRow2[i]] == 1)
return false;
else
list[arrayRow2[i]] = 1;
if(list[arrayRow3[i]] == 1)
return false;
else
list[arrayRow3[i]] = 1;
}
return true;
}
bool checkRowSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
int sum = 0;
int sumnew = 0;
for(int i = 0; i<size; i++)
sum += arrayRow1[i];
for(int i = 0; i<size; i++)
sumnew += arrayRow1[i];
if(sumnew!=sum)
return false;
sumnew = 0;
for(int i = 0; i<size; i++)
sumnew += arrayRow1[i];
if(sumnew!=sum)
return false;
return true;
}
bool checkColSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
int sum = 0;
int sumnew = 0;
sum = arrayRow1[0] + arrayRow2[0] + arrayRow3[0];
sumnew = arrayRow1[1] + arrayRow2[1] + arrayRow3[1];
if(sum!=sumnew)
return false;
sumnew = 0;
sumnew = arrayRow1[2] + arrayRow2[2] + arrayRow3[2];
if(sum!=sumnew)
return false;
return true;
}
bool checkDiagSum(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
int sum = 0;
sum = arrayRow1[0] + arrayRow2[1] + arrayRow3[2];
int sumnew = arrayRow1[2] + arrayRow2[1] + arrayRow3[0];
if(sum!=sumnew)
return false;
return true;
}
void fillArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
for(int i = 0; i<size; i++){
cout<<"Enter elements of row 0 and column "<<i<<": ";
cin>>arrayRow1[i];
}
for(int i = 0; i<size; i++){
cout<<"Enter elements of row 1 and column "<<i<<": ";
cin>>arrayRow2[i];
}
for(int i = 0; i<size; i++){
cout<<"Enter elements of row 2 and column "<<i<<": ";
cin>>arrayRow3[i];
}
}
void showArray(int arrayRow1[], int arrayRow2[], int arrayRow3[], int size){
for(int i = 0; i<size; i++)
cout<<arrayRow1[i]<<" ";
cout<<"\n";
for(int i = 0; i<size; i++)
cout<<arrayRow2[i]<<" ";
cout<<"\n";
for(int i = 0; i<size; i++)
cout<<arrayRow3[i]<<" ";
cout<<"\n";
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

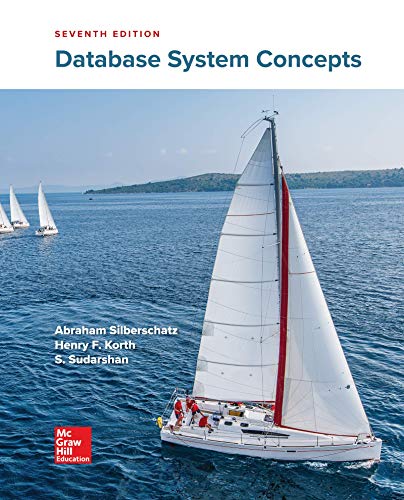
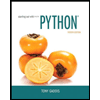
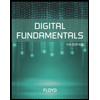
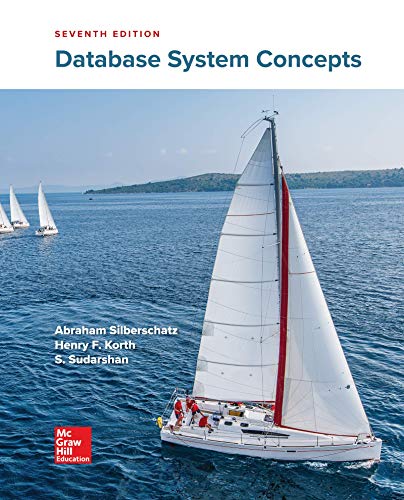
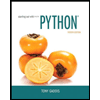
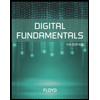
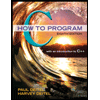
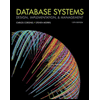
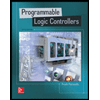