Statistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the average and max. A negative integer ends the input and is not included in the statistics. Ex: When the input is: 15 20 0 5 -1 the output is: 10 20 You can assume that at least one non-negative integer is input. This is the code I am using: import java.util.Scanner; public class LabProgram { public static void main(String[] args) { Scanner input = new Scanner(System.in); int[] a= new int[100]; int i=0, sum=0; double avg, max=0; while(true){ a[i]=input.nextInt(); if(a[i]<0) break; else sum=sum+a[i]; // sum the numbers i++; } avg=(double)sum/i; //compute average for(int j=0; j
Statistics are often calculated with varying amounts of input data. Write a program that takes any number of non-negative integers as input, and outputs the average and max. A negative integer ends the input and is not included in the statistics.
Ex: When the input is:
15 20 0 5 -1
the output is:
10 20
You can assume that at least one non-negative integer is input.
This is the code I am using:
import java.util.Scanner;
public class LabProgram {
public static void main(String[] args) {
Scanner input = new Scanner(System.in);
int[] a= new int[100];
int i=0, sum=0;
double avg, max=0;
while(true){
a[i]=input.nextInt();
if(a[i]<0)
break;
else
sum=sum+a[i]; // sum the numbers
i++;
}
avg=(double)sum/i; //compute average
for(int j=0; j<i;j++)
if(max<a[j])
max=a[j];
System.out.printf("%.2f %.2f\n", avg,max); // print result
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

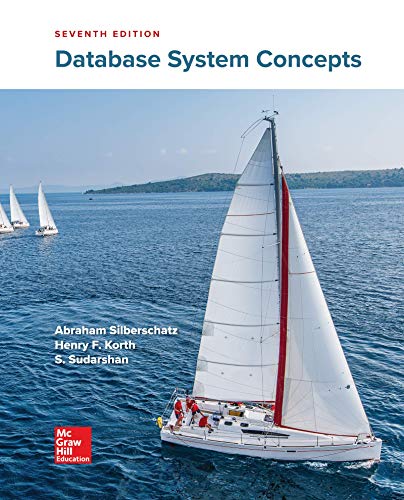
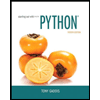
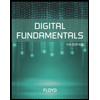
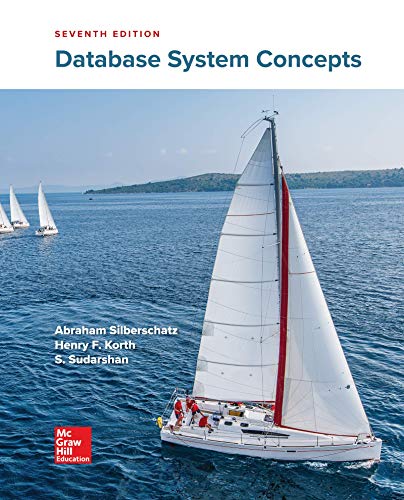
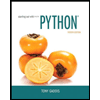
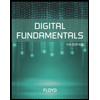
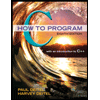
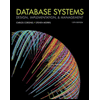
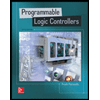