ss Node: def __init__(self, value): self.value = va
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Binary Search Tree Homework
Starter Code:
class Node:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def __str__(self):
return ("Node({})".format(self.value))
__repr__ = __str__
class BinarySearchTree:
'''
>>> x=BinarySearchTree()
>>> x.isEmpty()
True
>>> x.insert(9)
>>> x.insert(4)
>>> x.insert(11)
>>> x.insert(2)
>>> x.insert(5)
>>> x.insert(10)
>>> x.insert(9.5)
>>> x.insert(7)
>>> x.getMin
Node(2)
>>> x.getMax
Node(11)
>>> 67 in x
False
>>> 9.5 in x
True
>>> x.isEmpty()
False
>>> x.getHeight(x.root) # Height of the tree
3
>>> x.getHeight(x.root.left.right)
1
>>> x.getHeight(x.root.right)
2
>>> x.getHeight(x.root.right.left)
1
>>> x.printInorder
2 : 4 : 5 : 7 : 9 : 9.5 : 10 : 11 :
>>> new_tree = x.mirror()
11 : 10 : 9.5 : 9 : 7 : 5 : 4 : 2 :
>>> new_tree.root.right
Node(4)
>>> x.printInorder
2 : 4 : 5 : 7 : 9 : 9.5 : 10 : 11 :
'''
def __init__(self, value):
self.value = value
self.left = None
self.right = None
def __str__(self):
return ("Node({})".format(self.value))
__repr__ = __str__
class BinarySearchTree:
'''
>>> x=BinarySearchTree()
>>> x.isEmpty()
True
>>> x.insert(9)
>>> x.insert(4)
>>> x.insert(11)
>>> x.insert(2)
>>> x.insert(5)
>>> x.insert(10)
>>> x.insert(9.5)
>>> x.insert(7)
>>> x.getMin
Node(2)
>>> x.getMax
Node(11)
>>> 67 in x
False
>>> 9.5 in x
True
>>> x.isEmpty()
False
>>> x.getHeight(x.root) # Height of the tree
3
>>> x.getHeight(x.root.left.right)
1
>>> x.getHeight(x.root.right)
2
>>> x.getHeight(x.root.right.left)
1
>>> x.printInorder
2 : 4 : 5 : 7 : 9 : 9.5 : 10 : 11 :
>>> new_tree = x.mirror()
11 : 10 : 9.5 : 9 : 7 : 5 : 4 : 2 :
>>> new_tree.root.right
Node(4)
>>> x.printInorder
2 : 4 : 5 : 7 : 9 : 9.5 : 10 : 11 :
'''
def __init__(self):
self.root = None
def insert(self, value):
if self.root is None:
self.root=Node(value)
else:
self._insert(self.root, value)
def _insert(self, node, value):
if(value<node.value):
if(node.left==None):
node.left = Node(value)
else:
self._insert(node.left, value)
else:
if(node.right==None):
node.right = Node(value)
else:
self._insert(node.right, value)
@property
def printInorder(self):
if self.isEmpty():
return None
else:
self._inorderHelper(self.root)
def _inorderHelper(self, node):
if node is not None:
self._inorderHelper(node.left)
print(node.value, end=' : ')
self._inorderHelper(node.right)
def mirror(self):
# Creates a new BST that is a mirror of self:
# Elements greater than the root are on the left side, and smaller
values on the right side
# Do NOT modify any given code
if self.root is None:
return None
else:
newTree = BinarySearchTree()
newTree.root = self._mirrorHelper(self.root)
newTree.printInorder
return newTree
def isEmpty(self):
# YOUR CODE STARTS HERE
pass
self.root = None
def insert(self, value):
if self.root is None:
self.root=Node(value)
else:
self._insert(self.root, value)
def _insert(self, node, value):
if(value<node.value):
if(node.left==None):
node.left = Node(value)
else:
self._insert(node.left, value)
else:
if(node.right==None):
node.right = Node(value)
else:
self._insert(node.right, value)
@property
def printInorder(self):
if self.isEmpty():
return None
else:
self._inorderHelper(self.root)
def _inorderHelper(self, node):
if node is not None:
self._inorderHelper(node.left)
print(node.value, end=' : ')
self._inorderHelper(node.right)
def mirror(self):
# Creates a new BST that is a mirror of self:
# Elements greater than the root are on the left side, and smaller
values on the right side
# Do NOT modify any given code
if self.root is None:
return None
else:
newTree = BinarySearchTree()
newTree.root = self._mirrorHelper(self.root)
newTree.printInorder
return newTree
def isEmpty(self):
# YOUR CODE STARTS HERE
pass
def _mirrorHelper(self, node):
# YOUR CODE STARTS HERE
pass
@property
def getMin(self):
# YOUR CODE STARTS HERE
pass
@property
def getMax(self):
# YOUR CODE STARTS HERE
pass
def __contains__(self,value):
# YOUR CODE STARTS HERE
pass
def getHeight(self, node):
# YOUR CODE STARTS HERE
pass
# YOUR CODE STARTS HERE
pass
@property
def getMin(self):
# YOUR CODE STARTS HERE
pass
@property
def getMax(self):
# YOUR CODE STARTS HERE
pass
def __contains__(self,value):
# YOUR CODE STARTS HERE
pass
def getHeight(self, node):
# YOUR CODE STARTS HERE
pass
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
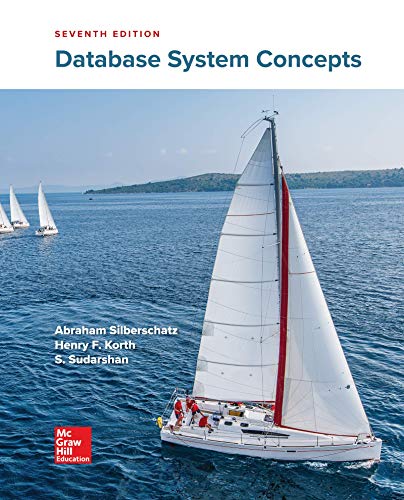
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
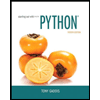
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
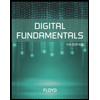
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
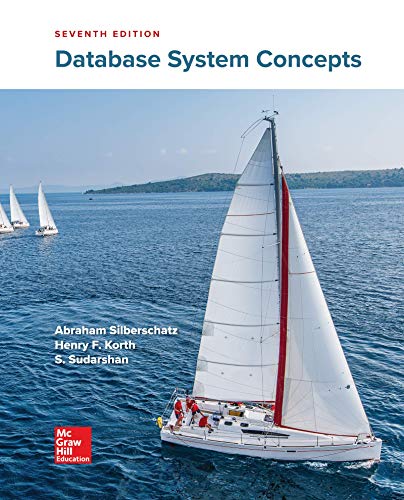
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
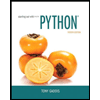
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
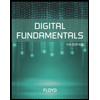
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
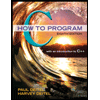
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
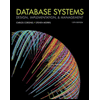
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
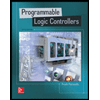
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education