Sentence Capitalizer. Complete the program that capitalizes the first letter of every sentence. The program contains a function prototype for the function named sentenceCapitalizer. The function receives a C- string as the parameter. The function does not have any value to return. The main function reads a string from the user and then displays the string as is. It then calls sentenceCapitalizer to perform the function and prints the result afterwards. Your job is to write the function definition for sentenceCapitalizer In the function body, walk through the string one position at a time. For each character in the string, determine whether it is the first letter of a sentence and capitalize it accordingly. The sentences may be separated with no spaces or any number of spaces. Your algorithm should not assume that the first letter is always one space away from the previous end of a sentence marker. An end of sentence marker could be: a period (.), a question mark (?) or an escalation point(!). Test the program with the following input (*) including the blanks at the beginning: pointers are extremely useful for writing functions that process c-strings. if the starting address of a string is pass ed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that hold s the null terminator, are part of the string. it isn't necessary to know the length of the array that holds the string. The output should look exactly as follows. The text before the modification: pointers are extremely useful for writing functions that process c-strings. if the sta rting address of a string is passed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that holds the null terminator, are part of the string. he array that holds the string. it isn’t necessary to know the length of t The text after the modification: Pointers are extremely useful for writing functions that process c-strings. If the star ting address of a string is passed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that holds the null terminator, are part of the string. he array that holds the string. It isn't necessary to know the length of t
Sentence Capitalizer. Complete the program that capitalizes the first letter of every sentence. The program contains a function prototype for the function named sentenceCapitalizer. The function receives a C- string as the parameter. The function does not have any value to return. The main function reads a string from the user and then displays the string as is. It then calls sentenceCapitalizer to perform the function and prints the result afterwards. Your job is to write the function definition for sentenceCapitalizer In the function body, walk through the string one position at a time. For each character in the string, determine whether it is the first letter of a sentence and capitalize it accordingly. The sentences may be separated with no spaces or any number of spaces. Your algorithm should not assume that the first letter is always one space away from the previous end of a sentence marker. An end of sentence marker could be: a period (.), a question mark (?) or an escalation point(!). Test the program with the following input (*) including the blanks at the beginning: pointers are extremely useful for writing functions that process c-strings. if the starting address of a string is pass ed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that hold s the null terminator, are part of the string. it isn't necessary to know the length of the array that holds the string. The output should look exactly as follows. The text before the modification: pointers are extremely useful for writing functions that process c-strings. if the sta rting address of a string is passed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that holds the null terminator, are part of the string. he array that holds the string. it isn’t necessary to know the length of t The text after the modification: Pointers are extremely useful for writing functions that process c-strings. If the star ting address of a string is passed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that holds the null terminator, are part of the string. he array that holds the string. It isn't necessary to know the length of t
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
Unless specifically instructed, do not prompt the user for input during the execution of your program. Do not pause at the end of the execution waiting for the user's input.
The first one is the required template that is said in the description
![#include <iostream>
#include <iomanip>
#include <string>
#include <cctype>
using namespace std;
void sentenceCapitalizer(char *userString);
int main() {
const int MAX_SIZE = 1024;
char userString[MAX_SIZE];
//get user string
cin.getline(userString, MAX_SIZE);
//print userString uncapitalized
cout << "The text before the modification:";
cout << userString << '\n';
//capitalize userString
sentenceCapitalizer(userString);
//print userstring again
cout << "The text after the modification:";
cout << userString << '\n';
return 0;
}
//****
***
//* Function name: sentenceCapitalizer
//*
//* This function capitalizes the first letter in each sentence contained
//* in a passed C-string.
//* Parameters:
// *
userString
pointer to the caller's C-string to be capitalized
//*
//* Returns:
//*
//*
void
//*
/*
**************
**************
void sentenceCapitalizer(char *userString) {
// Write your code here ..](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8d2af6b8-35d3-4327-94c1-a05ff77beaf0%2F3d7c82dd-4c25-453b-8139-828369044b91%2Fdgdbz7d_processed.png&w=3840&q=75)
Transcribed Image Text:#include <iostream>
#include <iomanip>
#include <string>
#include <cctype>
using namespace std;
void sentenceCapitalizer(char *userString);
int main() {
const int MAX_SIZE = 1024;
char userString[MAX_SIZE];
//get user string
cin.getline(userString, MAX_SIZE);
//print userString uncapitalized
cout << "The text before the modification:";
cout << userString << '\n';
//capitalize userString
sentenceCapitalizer(userString);
//print userstring again
cout << "The text after the modification:";
cout << userString << '\n';
return 0;
}
//****
***
//* Function name: sentenceCapitalizer
//*
//* This function capitalizes the first letter in each sentence contained
//* in a passed C-string.
//* Parameters:
// *
userString
pointer to the caller's C-string to be capitalized
//*
//* Returns:
//*
//*
void
//*
/*
**************
**************
void sentenceCapitalizer(char *userString) {
// Write your code here ..

Transcribed Image Text:Download the file Lab1d.cpp t. Use an IDE to complete it according to the instruction below.
Sentence Capitalizer.
Complete the program that capitalizes the first letter of every sentence.
The program contains a function prototype for the function named sentenceCapitalizer. The function receives a C-
string as the parameter. The function does not have any value to return.
The main function reads a string from the user and then displays the string as is. It then calls sentenceCapitalizer to
perform the function and prints the result afterwards.
Your job is to write the function definition for sentenceCapitalizer
In the function body, walk through the string one position at a time. For each character in the string, determine whether
it is the first letter of a sentence and capitalize it accordingly. The sentences may be separated with no spaces or any
number of spaces. Your algorithm should not assume that the first letter is always one space away from the previous
end of a sentence marker. An end of sentence marker could be: a period (.), a question mark (?) or an escalation point(!).
Test the program with the following input (*) including the blanks at the beginning:
pointers are extremely useful for writing functions that process c-strings.
ed into a pointer parameter variable, it can be assumed that all the characters, from that address up to the byte that hold
s the null terminator, are part of the string.
if the starting address of a string is pass
it isn't necessary to know the length of the array that holds the string.
The output should look exactly as follows.
The text before the modification:
pointers are extremely useful for writing functions that process c-strings. if the sta
rting address of a string is passed into a pointer parameter variable, it can be assumed that all the characters, from that
address up to the byte that holds the null terminator, are part of the string.
he array that holds the string.
it isn't necessary to know the length of t
The text after the modification:
Pointers are extremely useful for writing functions that process c-strings. If the star
ting address of a string is passed into a pointer parameter variable, it can be assumed that all the characters, from that
address up to the byte that holds the null terminator, are part of the string.
he array that holds the string.
It isn’t necessary to know the length of t
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Recommended textbooks for you
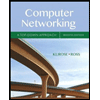
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
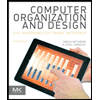
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
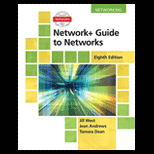
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
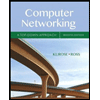
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
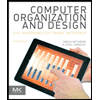
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
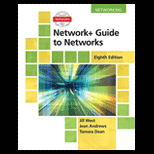
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
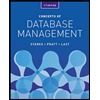
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
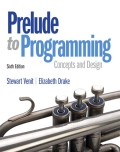
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
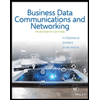
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY