Section 1: FIFO Page Replacement Algorithm In FIFO page replacement, the pages are simply placed in a queue when they enter the system; when a replacement occurs, the page at the beginning of the queue (the "first-in" page) is evicted. FIF0 has one great strength: it is quite simple to implement. Developing the FIFO page replacement algorithm For the FIFO page replacement algorithm, read the number of pages in the sequence, the page number sequence string, and the number of frames. Identify the page faults and calculate the total number of page faults. FIRST IN FIRST OUT (FIFO) Number of Frames: Number of Pages: 3 20 Page numbers: 7 01 11 21 3 4 2 1 2 1 7 1 Page Frame 1 Page Frame 2 Page Frame 3 7 7 2 2 2 2 4 4 4 7 7 7 3 3 2 2 2 1 1 1 1 1 1 1 3 3 3 3 2 2 2 2 Page Fault # 1 2 3 4 4 7 8. 9. 10 10 10 11 12 12 12 13 14 15 3. 2.
Filll in the below code in C program:
#include <stdio.h>
void main()
{
int pageNumbers[25], frameContents[25];
int i = 0, j = 0, k = 0;
int numPages = 0, numFrames = 0, totalPageFaults = 0;
int frameNumber = 0;
printf("\n Page Replacement Method: FIFO");
printf("\n Enter the number of pages in the page sequence: ");
// 20
scanf("%d", &numPages);
printf("\n Enter the page numbers in sequence as a string: ");
// 7 0 1 2 0 3 0 4 2 3 0 3 2 1 2 0 1 7 0 1
for (i = 0; i < numPages; i++)
scanf("%d", &pageNumbers[i]);
printf("\n Enter the number of frames: ");
// 3
scanf("%d", &numFrames);
for (i = 0; i < numFrames; i++)
frameContents[i] = -1;
printf("\n The Page Replacement Process is \n");
printf("\tREF STRING");
for (j = 0; j < numFrames; j++)
printf("\tPAGE_FRAME_%d", j + 1);
printf("\tPAGE_FAULT_NUMBER\n");
/* Find the occurance of page faults and the total number of page faults */
printf("\n\n Total number of Page Faults using FIFO: %d \n", totalPageFaults);
}



Step by step
Solved in 4 steps with 3 images

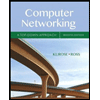
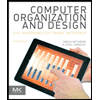
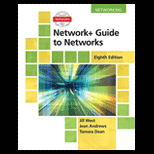
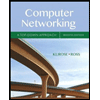
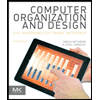
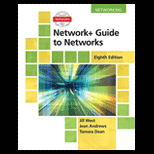
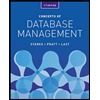
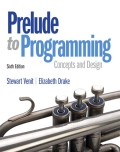
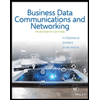