Rewrite this while statement as a for statement. int count = 0; while(counter < width) { rect(x, y, 30, 50); counter ++; }
Rewrite this while statement as a for statement. int count = 0; while(counter < width) { rect(x, y, 30, 50); counter ++; }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question

Transcribed Image Text:**Objective: Convert a While Loop to a For Loop**
In this task, we are asked to rewrite the given `while` statement as a `for` statement. Here's the original code:
```c
int count = 0;
while(counter < width)
{
rect(x, y, 30, 50);
counter++;
}
```
### Steps to Convert
1. **Initialization**: The variable `count` is initialized to 0. However, note that within the `while` loop, the variable `counter` is the one being used. Let's assume `counter` should be initialized, possibly in a different section of your code.
2. **Condition**: The loop continues as long as `counter` is less than `width`.
3. **Increment**: Inside the loop, `counter` is incremented by 1 in each iteration.
4. **Action**: A rectangle is drawn using the `rect` function with parameters `(x, y, 30, 50)`.
### Converted Code
Below is the equivalent `for` loop statement:
```c
for (int counter = 0; counter < width; counter++)
{
rect(x, y, 30, 50);
}
```
### Explanation
- **Initialization**: The loop initialization `int counter = 0` is done in the `for` loop declaration.
- **Condition**: `counter < width` serves as the loop continuation condition.
- **Increment**: The increment operation `counter++` is placed as the third expression in the `for` loop header, ensuring it runs after each iteration.
- **Action**: The `rect` function is called within the loop body as before.
This refactoring provides a more concise and clear structure, often preferred in professional programming for loops with simple counting logic.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Recommended textbooks for you
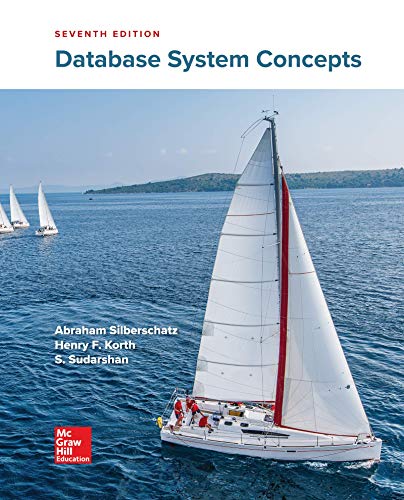
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
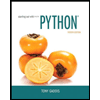
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
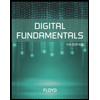
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
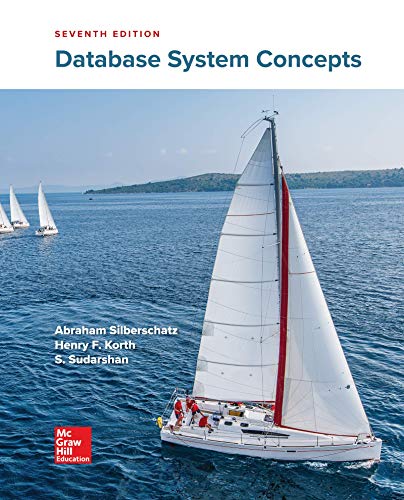
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
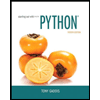
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
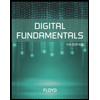
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
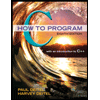
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
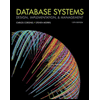
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
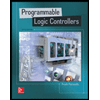
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education