Rewrite the following code using a while loop. int sum = 0; int count = 0; for(int i = 1; i < 1048; i i*2) { sum = sum + i; count++; II
Rewrite the following code using a while loop. int sum = 0; int count = 0; for(int i = 1; i < 1048; i i*2) { sum = sum + i; count++; II
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Attached is a question, using java.

Transcribed Image Text:**Rewriting a for Loop as a while Loop**
**Original Code Using a for Loop:**
```cpp
int sum = 0;
int count = 0;
for(int i = 1; i < 1048; i = i*2) {
sum = sum + i;
count++;
}
```
In this code snippet, we use a `for` loop to sum the values of `i`, where `i` starts from 1 and is multiplied by 2 every iteration until it reaches 1048. Additionally, the `count` variable is incremented with each iteration to keep track of how many times the loop has executed.
**Equivalent Code Using a while Loop:**
```cpp
int sum = 0;
int count = 0;
int i = 1; // Initialize iterator variable outside of the loop
while(i < 1048) {
sum = sum + i;
count++;
i = i * 2; // Update the iterator variable inside the loop
}
```
### Explanation:
1. **Initial Setup**: Similar to the `for` loop, we initialize the variables `sum`, `count`, and `i` before entering the `while` loop.
2. **Loop Condition**: The `while` loop continues to execute as long as `i` is less than 1048.
3. **Inside the Loop**: Within the `while` loop, we:
- Add the current value of `i` to `sum`.
- Increment the `count` variable.
- Multiply `i` by 2 to get the next value in the sequence.
By making these adjustments, we achieve the same functionality as the original `for` loop using a `while` loop.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
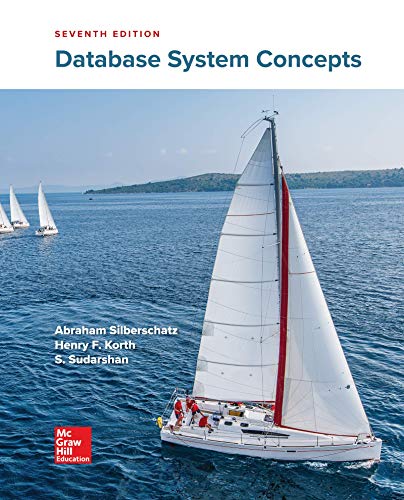
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
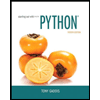
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
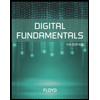
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
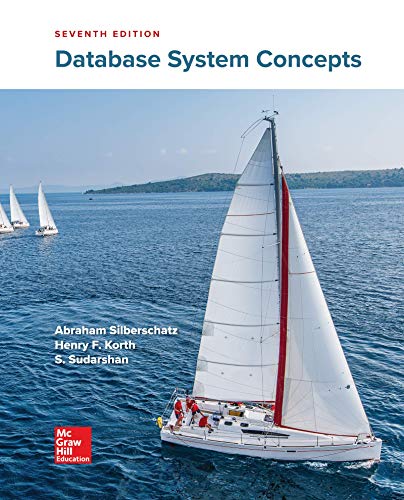
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
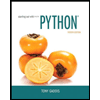
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
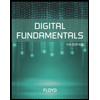
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
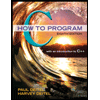
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
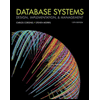
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
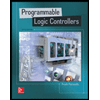
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education