Rewrite the following code using a for loop. long prod = 5; 1 = int x = 3; boolean valid = true; while (x <= 22 && valid) { prod = prod * prod; x = x + 5;
Rewrite the following code using a for loop. long prod = 5; 1 = int x = 3; boolean valid = true; while (x <= 22 && valid) { prod = prod * prod; x = x + 5;
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Attached is a question, using java.

Transcribed Image Text:### Converting a While Loop to a For Loop
In this section, we will learn how to rewrite a `while` loop using a `for` loop. Consider the following code snippet:
```java
long prod = 5;
int x = 3;
boolean valid = true;
while(x <= 22 && valid) {
prod = prod * prod;
x = x + 5;
}
```
#### Explanation of the Given Code
1. **Initialization:**
- A `long` variable `prod` is initialized to `5`.
- An `int` variable `x` is initialized to `3`.
- A `boolean` variable `valid` is set to `true`.
2. **While Loop Condition:**
The loop will continue to execute as long as both conditions are true:
- `x` is less than or equal to `22`.
- `valid` is `true`.
3. **Loop Body:**
- `prod` is multiplied by itself and the result is stored back in `prod`.
- `x` is incremented by `5`.
#### Conversion to a For Loop
To rewrite this `while` loop using a `for` loop, we need to incorporate the initialization, condition, and increment/decrement steps in the syntax of the `for` loop. Here's how you can do this:
```java
long prod = 5;
boolean valid = true;
for(int x = 3; x <= 22 && valid; x += 5) {
prod = prod * prod;
}
```
- **Initialization:** `int x = 3` is placed in the initialization section of the `for` loop.
- **Condition:** `x <= 22 && valid` remains as the condition for the loop.
- **Increment:** `x = x + 5` is translated to `x += 5` and placed in the increment section of the `for` loop.
- **Loop Body:** The body of the loop remains unchanged.
By using a `for` loop, we can write more concise and potentially more readable code.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
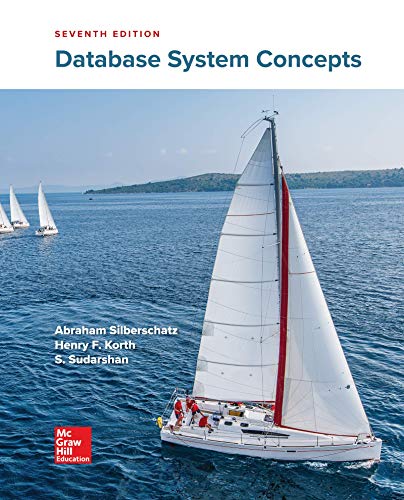
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
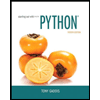
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
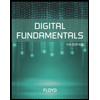
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
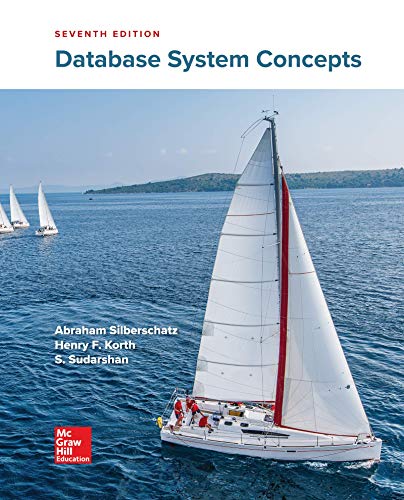
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
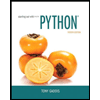
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
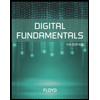
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
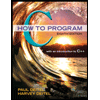
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
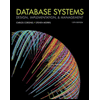
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
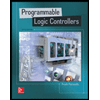
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education