* Removes the specified key and its associated value from this symbol table * (if the key is in this symbol table). Takes advantage of the fact that the * keys appear in increasing order to terminate` early when possible. * * @param key the key * @throws IllegalArgumentException if {@code key} is {@code null} */ public void delete(Key key) { // TODO // Change this code to make use of the fact that the list is sorted to // terminate early when possible. // Also use a loop instead of recursion! So remove the recursive helper // function below. if (key == null) throw new IllegalArgumentException("argument to delete() is null"); first = delete(first, key); } // delete key in linked list beginning at Node x // warning: function call stack too large if table is large private Node delete(Node x, Key key) { // TODO // Remove this function. Delete should no longer be recursive. if (x == null) return null; if (key.equals(x.key)) { n--; return x.next; } x.next = delete(x.next, key); return x; } You cannot use the keys() method Note: You are modifying the implementation of the methods, but not their interface or contract.
* Removes the specified key and its associated value from this symbol table
* (if the key is in this symbol table). Takes advantage of the fact that the
* keys appear in increasing order to terminate` early when possible.
*
* @param key the key
* @throws IllegalArgumentException if {@code key} is {@code null}
*/
public void delete(Key key) {
// TODO
// Change this code to make use of the fact that the list is sorted to
// terminate early when possible.
// Also use a loop instead of recursion! So remove the recursive helper
// function below.
if (key == null) throw new IllegalArgumentException("argument to delete() is null");
first = delete(first, key);
}
// delete key in linked list beginning at Node x
// warning: function call stack too large if table is large
private Node delete(Node x, Key key) {
// TODO
// Remove this function. Delete should no longer be recursive.
if (x == null) return null;
if (key.equals(x.key)) {
n--;
return x.next;
}
x.next = delete(x.next, key);
return x;
}
You cannot use the keys() method
Note: You are modifying the implementation of the methods, but not their interface or contract.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

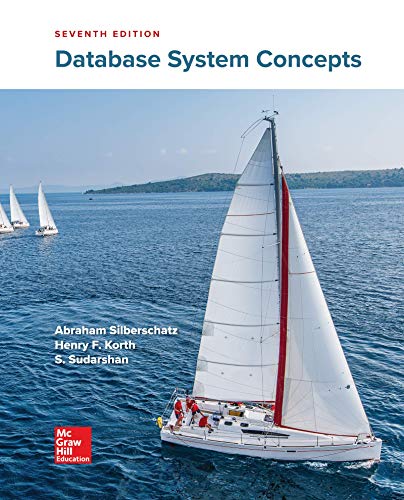
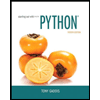
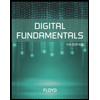
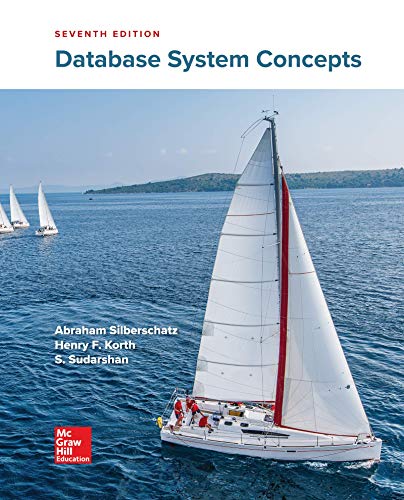
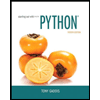
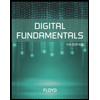
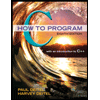
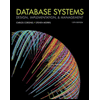
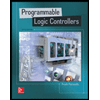