Recursion: Complete the definition for sum_prime_digits, which returns the sum of all the prime digits of n. Recall that 0 and 1 are not prime numbers. Assume you have the function is_prime() defined already and ready to use (Ex: is_prime(5) will return True). You may use the below guidelines or you may suggest your own solution. Your solution should be recursive. def sum prime_digits (n): >>> sum prime_digits (12345) 10 >>> sum_prime_digits (4681029) 2 if return else: if return return 2 W 9 # 3 E F 3 $ 4 R DII % 5 65 T K A 6 Y 17 & 7 Prisen U 16 8
Recursion: Complete the definition for sum_prime_digits, which returns the sum of all the prime digits of n. Recall that 0 and 1 are not prime numbers. Assume you have the function is_prime() defined already and ready to use (Ex: is_prime(5) will return True). You may use the below guidelines or you may suggest your own solution. Your solution should be recursive. def sum prime_digits (n): >>> sum prime_digits (12345) 10 >>> sum_prime_digits (4681029) 2 if return else: if return return 2 W 9 # 3 E F 3 $ 4 R DII % 5 65 T K A 6 Y 17 & 7 Prisen U 16 8
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Python help now

Transcribed Image Text:### Educational Content on Recursion
**Recursion Task:**
The task is to complete the definition for the function `sum_prime_digits`, which returns the sum of all the prime digits in a given number `n`. Remember that 0 and 1 are not considered prime numbers. It is assumed that you have access to a pre-defined function `is_prime()` that can be used to check the primality of a digit. For example, `is_prime(5)` will return `True`.
You can follow the guidelines below or create your own solution. The solution must be recursive.
```python
def sum_prime_digits(n):
"""
Calculate the sum of all prime digits of n recursively.
Args:
n (int): The input number from which prime digits need to be summed.
Returns:
int: The sum of all prime digits in the input number.
"""
# Base case
if n == 0:
return 0
else:
current_digit = n % 10 # Get the last digit of n
# Recursive call with the remainder of the number
if is_prime(current_digit):
return current_digit + sum_prime_digits(n // 10)
else:
return sum_prime_digits(n // 10)
# Example usage:
>>> sum_prime_digits(12345) # Evaluates the sum of prime digits in 12345
10
>>> sum_prime_digits(4681029) # Evaluates the sum of prime digits in 4681029
2
```
**Explanation:**
- The function `sum_prime_digits` recursively determines the sum of prime digits of an integer `n`.
- The base case returns `0` if `n` is `0`.
- Uses the modulo operation to extract the last digit of `n`.
- Checks if the extracted digit is prime with `is_prime()`. If true, it adds the digit to the recursive call. If not, it simply makes the recursive call without adding the digit.
- Example calls demonstrate how the function evaluates the sum of prime digits in the numbers `12345` and `4681029`.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
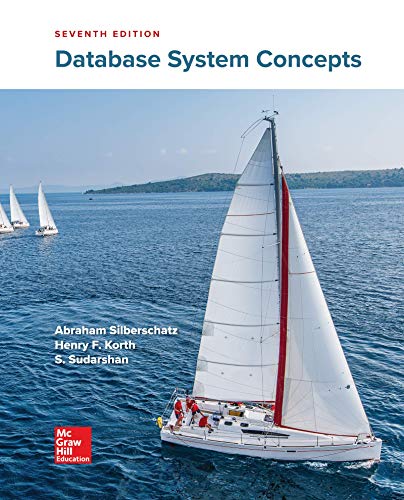
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
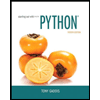
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
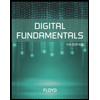
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
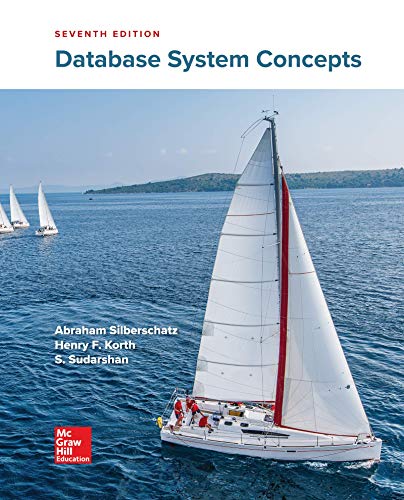
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
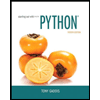
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
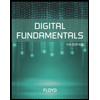
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
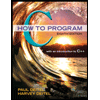
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
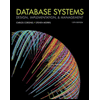
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
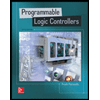
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education