Recall that an application of the Stack ADT is postfix expression evaluation. As a reminder, here is pseudocode for the algorithm: scan given postfix expression; for each symbol in postfix if operand then push its value onto a stack S; if operator then ( pop operand2; pop operand1; apply operator to compute operand1 op operand2; push result back onto stack S; return value at top of stack; Evaluate the following postfix expression: 6 5 3 + 2 12 5 % +
Recall that an application of the Stack ADT is postfix expression evaluation. As a reminder, here is pseudocode for the algorithm: scan given postfix expression; for each symbol in postfix if operand then push its value onto a stack S; if operator then ( pop operand2; pop operand1; apply operator to compute operand1 op operand2; push result back onto stack S; return value at top of stack; Evaluate the following postfix expression: 6 5 3 + 2 12 5 % +
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![### Postfix Expression Evaluation Using Stack ADT
An application of the Stack ADT (Abstract Data Type) is the evaluation of postfix expressions. The following is a pseudocode algorithm for the postfix expression evaluation:
1. **Scan the given postfix expression.**
2. **For each symbol in the postfix expression:**
- If the symbol is an operand, push its value onto a stack (Stack S).
- If the symbol is an operator, perform the following steps:
- Pop the top value from the stack; assign it to `operand2`.
- Pop the next top value from the stack; assign it to `operand1`.
- Apply the operator to `operand1` and `operand2` (i.e., compute `operand1 op operand2`).
- Push the result back onto the stack.
3. **Return the value that is on the top of the stack.**
#### Pseudocode:
```
scan given postfix expression;
for each symbol in postfix
if operand
then push its value onto a stack S;
if operator
then {
pop operand2;
pop operand1;
apply operator to compute operand1 op operand2;
push result back onto stack S;
}
return value at top of stack;
```
#### Example:
Evaluate the following postfix expression: `6 5 3 + 2 12 * + 5 % +`
To solve this expression using the stack algorithm:
1. **Scan** `6` and push to the stack.
Stack: `[6]`
2. **Scan** `5` and push to the stack.
Stack: `[6, 5]`
3. **Scan** `3` and push to the stack.
Stack: `[6, 5, 3]`
4. **Scan** `+` (operator); pop `3` and `5`, compute `5 + 3 = 8`, then push the result `8` back to the stack.
Stack: `[6, 8]`
5. **Scan** `2` and push to the stack.
Stack: `[6, 8, 2]`
6. **Scan** `12` and push to the stack.
Stack: `[6, 8, 2, 12]`
7. **Scan** `*` (operator); pop `12` and `2`,](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8a000fe0-342d-4f80-8d89-1b56a9b64020%2Fe844269e-9a3f-4225-b1eb-822068327c36%2Fa49nd5b_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Postfix Expression Evaluation Using Stack ADT
An application of the Stack ADT (Abstract Data Type) is the evaluation of postfix expressions. The following is a pseudocode algorithm for the postfix expression evaluation:
1. **Scan the given postfix expression.**
2. **For each symbol in the postfix expression:**
- If the symbol is an operand, push its value onto a stack (Stack S).
- If the symbol is an operator, perform the following steps:
- Pop the top value from the stack; assign it to `operand2`.
- Pop the next top value from the stack; assign it to `operand1`.
- Apply the operator to `operand1` and `operand2` (i.e., compute `operand1 op operand2`).
- Push the result back onto the stack.
3. **Return the value that is on the top of the stack.**
#### Pseudocode:
```
scan given postfix expression;
for each symbol in postfix
if operand
then push its value onto a stack S;
if operator
then {
pop operand2;
pop operand1;
apply operator to compute operand1 op operand2;
push result back onto stack S;
}
return value at top of stack;
```
#### Example:
Evaluate the following postfix expression: `6 5 3 + 2 12 * + 5 % +`
To solve this expression using the stack algorithm:
1. **Scan** `6` and push to the stack.
Stack: `[6]`
2. **Scan** `5` and push to the stack.
Stack: `[6, 5]`
3. **Scan** `3` and push to the stack.
Stack: `[6, 5, 3]`
4. **Scan** `+` (operator); pop `3` and `5`, compute `5 + 3 = 8`, then push the result `8` back to the stack.
Stack: `[6, 8]`
5. **Scan** `2` and push to the stack.
Stack: `[6, 8, 2]`
6. **Scan** `12` and push to the stack.
Stack: `[6, 8, 2, 12]`
7. **Scan** `*` (operator); pop `12` and `2`,
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
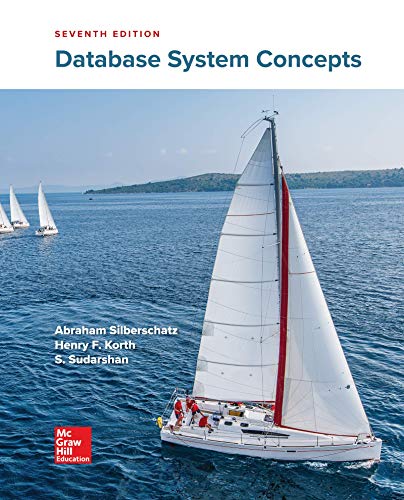
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
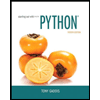
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
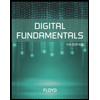
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
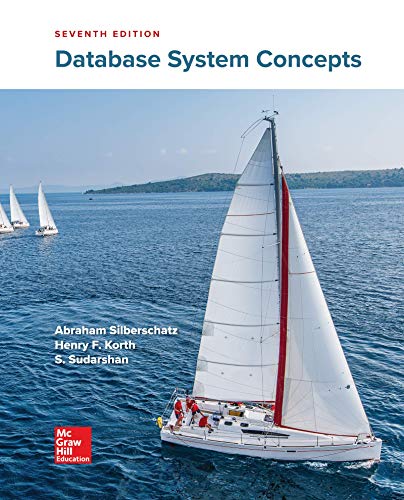
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
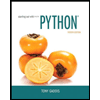
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
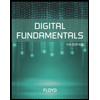
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
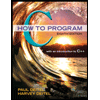
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
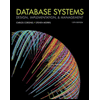
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
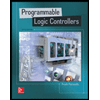
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education