Read the java program carefully, compile the program and understand the compilation errors. Don’t change access specifier for variables. What needs to be done to correct the compilation errors? class Box { private double width; private double height; private double depth; // compute and return volume double volume() { return width * height * depth; } } public class BoxDemo { public static void main(String args[]) { Box myBox1 = new Box(); Box myBox2 = new Box(); myBox1.width = 10; myBox1.height = 20; myBox1.depth = 30; myBox2.width = 40; myBox2.height = 40; myBox2.depth = 40; double vol; // get volume of first box vol = myBox1.volume(); System.out.println("Volume for myBox1 is " + vol); // get volume of second box vol = myBox2.volume(); System.out.println("Volume is " + vol); } }
Read the java program carefully, compile the program and understand the compilation errors. Don’t change access specifier for variables. What needs to be done to correct the compilation errors?
class Box {
private double width;
private double height;
private double depth;
// compute and return volume
double volume() {
return width * height * depth;
}
}
public class BoxDemo {
public static void main(String args[]) {
Box myBox1 = new Box();
Box myBox2 = new Box();
myBox1.width = 10;
myBox1.height = 20;
myBox1.depth = 30;
myBox2.width = 40;
myBox2.height = 40;
myBox2.depth = 40;
double vol;
// get volume of first box
vol = myBox1.volume();
System.out.println("Volume for myBox1 is " + vol);
// get volume of second box
vol = myBox2.volume();
System.out.println("Volume is " + vol);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

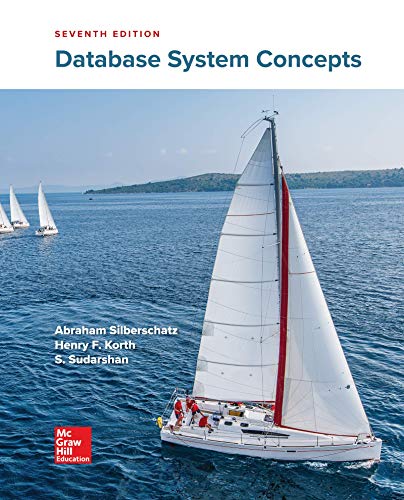
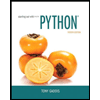
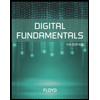
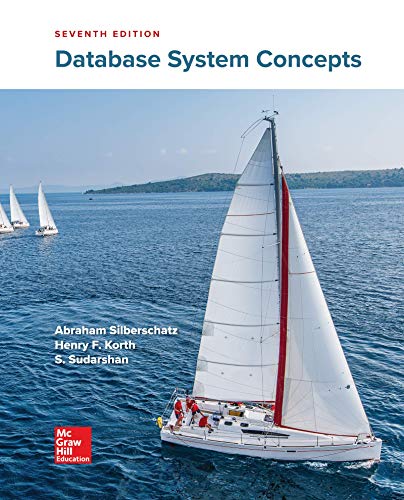
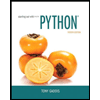
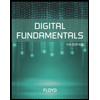
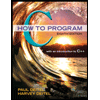
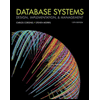
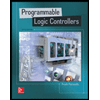