Read the following program project1.cpp carefully. Complete the missing code as indicated by the comments. Then compile, debug and test your program using the command lines below before your submission: c++ -o project1 project1.cpp ./project1 n m k Where n is the size of the stack, m is the modular of the real part and imagination part of a random complex number, and k is the number of elements displayed per line. In the main function, you need to 1. Get the values n, m, and k from the command line. 2. Declare a complex number stack of size n, generate n random complex numbers and push them into the stack. Meantime display all these numbers, k numbers per line. 3. Display all elements of the stack, k elements per line. 4. Create two random complex numbers c1 and c2, display c1, c2 and the results of the addition c1+c2, the subtraction c1-c2, the multiplication c1*c2, and the division c1/c2. // project1.cpp #include #include #include using namespace std; class Complex {// complex number class float re;// real part float im;// imagination part public: Complex(float r=0.0, float i=0.0){re=r; im=i;}// constructor Complex(const Complex& c){re=c.re; im=c.im;}// copy constructor void operator =(const Complex& c){re=c.re; im=c.im;}// assignment Complex operator -()const{return Complex(-re, -im);}// unary negation Complex operator +(const Complex&) const;// addition operator Complex operator -(const Complex&) const;// subtraction operator Complex operator *(const Complex&) const;// multiplication operator Complex operator /(const Complex&) const;//division operator friend ostream& operator <<(ostream&, const Complex&);// output operator }; // overloaded addition operator, to be implemented Complex Complex::operator +(const Complex& c) const {} // overloaded subtraction operator, to be implemented Complex Complex::operator -(const Complex& c) const{} // overloaded multiplication operator, to be implemented Complex Complex::operator *(const Complex& c) const {} // overloaded division operator, to be implemented Complex Complex::operator /(const Complex& c) const {} // overloaded stream output operator, to be implemented ostream& operator <<(ostream& os, const Complex& c) {} template class myStack{ T *ptr;// storage body int size;// storage size int top;// top position public: myStack(int);// constructor ~myStack(){free(ptr);}// destructor bool empty(){return top==-1;}// empty or not bool full(){return top==size-1;}// full or not int hold(){return top+1;}// number of items hold void push(T v){ptr[++top]=v;}// put an item on top T pop(){return ptr[top--];}// take an item from top void display(int);// display items }; // constructor that creates an empty stack, to be implemented template myStack::myStack(int s){} // display function, k items per line, to be implemented template void myStack::display(int k){} // Operator Overloading and Stack Template Program int main(int argc, char **argv){ // get n, m, and k from command line and create a complex number stack s(n) // generate n number of random complex numbers and push them onto the stack, // meantime display these complex numbers, k items per line // display all complex numbers in the stack, k elements per line // create two random complex numbers c1 and c2, display c1, c2 // display the results of the addition c1+c2, the subtraction c1-c2, the multiplication c1*c2, and the division c1/c2. return 0; }
Read the following program project1.cpp carefully. Complete the missing code as indicated by the comments. Then compile, debug and test your program using the command lines below before your submission:
c++ -o project1 project1.cpp <enter>
./project1 n m k <enter>
Where n is the size of the stack, m is the modular of the real part and imagination part of a random complex number, and k is the number of elements displayed per line.
In the main function, you need to
// project1.cpp
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
class Complex {// complex number class
float re;// real part
float im;// imagination part
public:
Complex(float r=0.0, float i=0.0){re=r; im=i;}// constructor
Complex(const Complex& c){re=c.re; im=c.im;}// copy constructor
void operator =(const Complex& c){re=c.re; im=c.im;}// assignment
Complex operator -()const{return Complex(-re, -im);}// unary negation
Complex operator +(const Complex&) const;// addition operator
Complex operator -(const Complex&) const;// subtraction operator
Complex operator *(const Complex&) const;// multiplication operator
Complex operator /(const Complex&) const;//division operator
friend ostream& operator <<(ostream&, const Complex&);// output operator
};
// overloaded addition operator, to be implemented
Complex Complex::operator +(const Complex& c) const {}
// overloaded subtraction operator, to be implemented
Complex Complex::operator -(const Complex& c) const{}
// overloaded multiplication operator, to be implemented
Complex Complex::operator *(const Complex& c) const {}
// overloaded division operator, to be implemented
Complex Complex::operator /(const Complex& c) const {}
// overloaded stream output operator, to be implemented
ostream& operator <<(ostream& os, const Complex& c) {}
template <class T> class myStack{
T *ptr;// storage body
int size;// storage size
int top;// top position
public:
myStack(int);// constructor
~myStack(){free(ptr);}// destructor
bool empty(){return top==-1;}// empty or not
bool full(){return top==size-1;}// full or not
int hold(){return top+1;}// number of items hold
void push(T v){ptr[++top]=v;}// put an item on top
T pop(){return ptr[top--];}// take an item from top
void display(int);// display items
};
// constructor that creates an empty stack, to be implemented
template <class T> myStack<T>::myStack(int s){}
// display function, k items per line, to be implemented
template <class T> void myStack<T>::display(int k){}
// Operator Overloading and Stack Template Program
int main(int argc, char **argv){
// get n, m, and k from command line and create a complex number stack s(n)
// generate n number of random complex numbers and push them onto the stack,
// meantime display these complex numbers, k items per line
// display all complex numbers in the stack, k elements per line
// create two random complex numbers c1 and c2, display c1, c2
// display the results of the addition c1+c2, the subtraction c1-c2, the multiplication c1*c2, and the division c1/c2.
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps

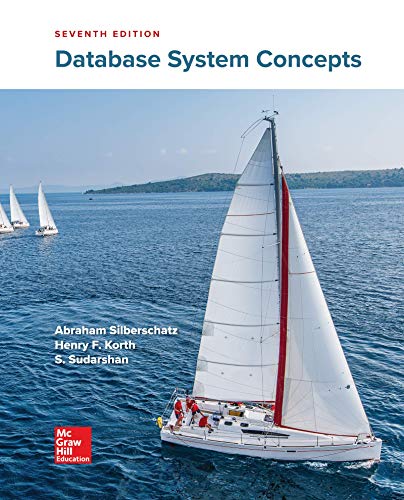
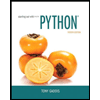
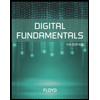
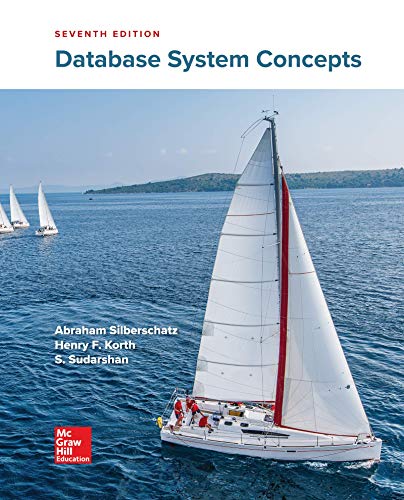
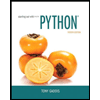
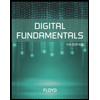
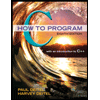
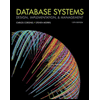
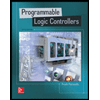