Question 9: Write a function decipher_code() that takes a string as its argument and returns a string. The function will process each sentence at a time (sentences are limited with a full-stop '.'). Each sentence will be converted to a list by string2list, then processed by flip-list to be deciphered into a meaningful text, then converted back to a string by list2string. The function will return all the deciphered sentences into one single string. Note: (1) For testing, it is guaranteed that the lengths of input strings are square numbers. 5 Example 1: H By Example 2: flip list у H B 1 2 flip list 2 4 3 4 1 3 Figure 3: Examples showing 2D lists before and after calling flip_list() function >>>decipher_code('BlHyoee 1') 'Hello Bye' >>>secret 'ttuo YrAtwoin L AundibKgSson.roelf ad YfImPAoitmr ucleoGAisrgora O' >>>decipher.code(secret) 'You Know About List And String. It Is Official You Are A Good Programmer' (2) Some sentences might be not valid (having numbers or special characters), they will then be ignored. Question 6: Write a function horizontal-flip that takes a 2D square list (a list that has an equal number of rows and columns) as its argument and flips the list horizontally. The function returns nothing and should modify the input list directly (see Fig 1). You will assume that the input list is a valid 2D square list containing any character or number. You must use nested for loops. >>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']] >>>horizontal.flip(input_list) >>>input_list [['H', 'I', 'B'], ['e', '0', 'y'], ['1', '', 'e']] Example 1: H Horizontal flip B у у B Example 2: Horizontal flip 3 4 3 Figure 1: Examples showing 2D lists before and after calling horizontal-flip() function Question 7: Write a function transpose that takes a 2D square list (a list that has an equal number of rows and columns) as its argument and transposes the list. Transposing the list will flip it over its diagonal. The function returns nothing and should modify the input list directly (see Fig. 2). You will assume that the input list is a valid 2D square list containing any character or number. You must use nested for loops. 4 Note: The transpose of a list A is the list B defined by making the first row of A the first column of B, the second row of A the second column of B, etc. In other words, when taking a transpose, the rows and columns are interchanged. See this link for an animation (Source: https://linearalgebra.math.umanitoba.ca) >>>input_list = [['H', 'I', 'B'], ['e', 'o', 'y'], ['1', '', 'e']] >>>transpose(input_list) >>>input_list [['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']] Example 1: H H Transpose y B Example 2: Transpose 1 Figure 2: Examples showing 2D lists before and after calling transpose() function Question 8: >>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']] >>>flip_list(input_list) >>>input_list [['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']] Write a function flip_list that takes a 2D square list as its argument and returns nothing. This function transforms the 2D list by changing the position of its items as shown in the example below. You will have to use the two previous functions (see Fig. 3). You will assume that the input list is a valid 2D square list containing any character or number.
Question 9: Write a function decipher_code() that takes a string as its argument and returns a string. The function will process each sentence at a time (sentences are limited with a full-stop '.'). Each sentence will be converted to a list by string2list, then processed by flip-list to be deciphered into a meaningful text, then converted back to a string by list2string. The function will return all the deciphered sentences into one single string. Note: (1) For testing, it is guaranteed that the lengths of input strings are square numbers. 5 Example 1: H By Example 2: flip list у H B 1 2 flip list 2 4 3 4 1 3 Figure 3: Examples showing 2D lists before and after calling flip_list() function >>>decipher_code('BlHyoee 1') 'Hello Bye' >>>secret 'ttuo YrAtwoin L AundibKgSson.roelf ad YfImPAoitmr ucleoGAisrgora O' >>>decipher.code(secret) 'You Know About List And String. It Is Official You Are A Good Programmer' (2) Some sentences might be not valid (having numbers or special characters), they will then be ignored. Question 6: Write a function horizontal-flip that takes a 2D square list (a list that has an equal number of rows and columns) as its argument and flips the list horizontally. The function returns nothing and should modify the input list directly (see Fig 1). You will assume that the input list is a valid 2D square list containing any character or number. You must use nested for loops. >>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']] >>>horizontal.flip(input_list) >>>input_list [['H', 'I', 'B'], ['e', '0', 'y'], ['1', '', 'e']] Example 1: H Horizontal flip B у у B Example 2: Horizontal flip 3 4 3 Figure 1: Examples showing 2D lists before and after calling horizontal-flip() function Question 7: Write a function transpose that takes a 2D square list (a list that has an equal number of rows and columns) as its argument and transposes the list. Transposing the list will flip it over its diagonal. The function returns nothing and should modify the input list directly (see Fig. 2). You will assume that the input list is a valid 2D square list containing any character or number. You must use nested for loops. 4 Note: The transpose of a list A is the list B defined by making the first row of A the first column of B, the second row of A the second column of B, etc. In other words, when taking a transpose, the rows and columns are interchanged. See this link for an animation (Source: https://linearalgebra.math.umanitoba.ca) >>>input_list = [['H', 'I', 'B'], ['e', 'o', 'y'], ['1', '', 'e']] >>>transpose(input_list) >>>input_list [['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']] Example 1: H H Transpose y B Example 2: Transpose 1 Figure 2: Examples showing 2D lists before and after calling transpose() function Question 8: >>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']] >>>flip_list(input_list) >>>input_list [['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']] Write a function flip_list that takes a 2D square list as its argument and returns nothing. This function transforms the 2D list by changing the position of its items as shown in the example below. You will have to use the two previous functions (see Fig. 3). You will assume that the input list is a valid 2D square list containing any character or number.
C++ Programming: From Problem Analysis to Program Design
8th Edition
ISBN:9781337102087
Author:D. S. Malik
Publisher:D. S. Malik
Chapter17: Linked Lists
Section: Chapter Questions
Problem 8PE
Related questions
Question
-
Do not use the built-in methods: find, index, count, break, continue a 50% penalty will be applied to your total point value for the question.
-
You may use the built-in method: join, append and the functions len, range
-
Do not use the index() or find() or replace() or count() methods or any other method/function that we didn’t see in class,
-
You HAVE to use a loop to traverse a string or a list.

Transcribed Image Text:Question 9:
Write a function decipher_code() that takes a string as its argument and returns a string.
The function will process each sentence at a time (sentences are limited with a full-stop '.').
Each sentence will be converted to a list by string2list, then processed by flip-list to
be deciphered into a meaningful text, then converted back to a string by list2string. The
function will return all the deciphered sentences into one single string.
Note:
(1) For testing, it is guaranteed that the lengths of input strings are square numbers.
5
Example 1:
H
By
Example 2:
flip list
у
H
B
1 2
flip list
2
4
3
4
1
3
Figure 3: Examples showing 2D lists before and after calling flip_list() function
>>>decipher_code('BlHyoee 1')
'Hello Bye'
>>>secret 'ttuo YrAtwoin L AundibKgSson.roelf ad YfImPAoitmr ucleoGAisrgora O'
>>>decipher.code(secret)
'You Know About List And String. It Is Official You Are A Good Programmer'
(2) Some sentences might be not valid (having numbers or special characters), they will then
be ignored.
![Question 6:
Write a function horizontal-flip that takes a 2D square list (a list that has an equal
number of rows and columns) as its argument and flips the list horizontally. The function
returns nothing and should modify the input list directly (see Fig 1). You will assume that
the input list is a valid 2D square list containing any character or number. You must use
nested for loops.
>>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']]
>>>horizontal.flip(input_list)
>>>input_list
[['H', 'I', 'B'], ['e', '0', 'y'], ['1', '', 'e']]
Example 1:
H
Horizontal flip
B у
у
B
Example 2:
Horizontal flip
3 4
3
Figure 1: Examples showing 2D lists before and after calling horizontal-flip() function
Question 7:
Write a function transpose that takes a 2D square list (a list that has an equal number of
rows and columns) as its argument and transposes the list. Transposing the list will flip it
over its diagonal. The function returns nothing and should modify the input list directly (see
Fig. 2). You will assume that the input list is a valid 2D square list containing any character
or number. You must use nested for loops.
4
Note: The transpose of a list A is the list B defined by making the first row of A the first
column of B, the second row of A the second column of B, etc. In other words, when taking a
transpose, the rows and columns are interchanged. See this link for an animation (Source:
https://linearalgebra.math.umanitoba.ca)
>>>input_list = [['H', 'I', 'B'], ['e', 'o', 'y'], ['1', '', 'e']]
>>>transpose(input_list)
>>>input_list
[['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']]
Example 1:
H
H
Transpose
y
B
Example 2:
Transpose
1
Figure 2: Examples showing 2D lists before and after calling transpose() function
Question 8:
>>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']]
>>>flip_list(input_list)
>>>input_list
[['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']]
Write a function flip_list that takes a 2D square list as its argument and returns nothing.
This function transforms the 2D list by changing the position of its items as shown in the
example below. You will have to use the two previous functions (see Fig. 3). You will assume
that the input list is a valid 2D square list containing any character or number.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F75248f5c-2719-4287-81a4-5e6a0d930fd1%2F949310b1-6c08-4a8e-9470-9ad29aed4243%2F19irc99_processed.png&w=3840&q=75)
Transcribed Image Text:Question 6:
Write a function horizontal-flip that takes a 2D square list (a list that has an equal
number of rows and columns) as its argument and flips the list horizontally. The function
returns nothing and should modify the input list directly (see Fig 1). You will assume that
the input list is a valid 2D square list containing any character or number. You must use
nested for loops.
>>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']]
>>>horizontal.flip(input_list)
>>>input_list
[['H', 'I', 'B'], ['e', '0', 'y'], ['1', '', 'e']]
Example 1:
H
Horizontal flip
B у
у
B
Example 2:
Horizontal flip
3 4
3
Figure 1: Examples showing 2D lists before and after calling horizontal-flip() function
Question 7:
Write a function transpose that takes a 2D square list (a list that has an equal number of
rows and columns) as its argument and transposes the list. Transposing the list will flip it
over its diagonal. The function returns nothing and should modify the input list directly (see
Fig. 2). You will assume that the input list is a valid 2D square list containing any character
or number. You must use nested for loops.
4
Note: The transpose of a list A is the list B defined by making the first row of A the first
column of B, the second row of A the second column of B, etc. In other words, when taking a
transpose, the rows and columns are interchanged. See this link for an animation (Source:
https://linearalgebra.math.umanitoba.ca)
>>>input_list = [['H', 'I', 'B'], ['e', 'o', 'y'], ['1', '', 'e']]
>>>transpose(input_list)
>>>input_list
[['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']]
Example 1:
H
H
Transpose
y
B
Example 2:
Transpose
1
Figure 2: Examples showing 2D lists before and after calling transpose() function
Question 8:
>>>input_list = [['B', 'I', 'H'], ['y', 'o', 'e'], ['e', '', '1']]
>>>flip_list(input_list)
>>>input_list
[['H', 'e', '1'], ['I', '0', ''], ['B', 'y', 'e']]
Write a function flip_list that takes a 2D square list as its argument and returns nothing.
This function transforms the 2D list by changing the position of its items as shown in the
example below. You will have to use the two previous functions (see Fig. 3). You will assume
that the input list is a valid 2D square list containing any character or number.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
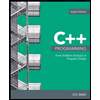
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
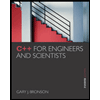
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
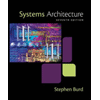
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
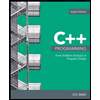
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
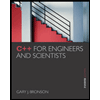
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
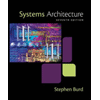
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning
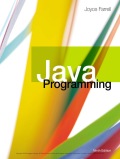
EBK JAVA PROGRAMMING
Computer Science
ISBN:
9781337671385
Author:
FARRELL
Publisher:
CENGAGE LEARNING - CONSIGNMENT
COMPREHENSIVE MICROSOFT OFFICE 365 EXCE
Computer Science
ISBN:
9780357392676
Author:
FREUND, Steven
Publisher:
CENGAGE L
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage