quadratic operator +( const quadratic& q1, const quadratic& q2 ); // Postcondition: The return value is the // quadratic expression obtained by adding // q1 and q2. For example, the c coefficient // of the return value is the sum of ql's c // coefficient and q2's c coefficient. quadratic operator *( double r, const quadratic& q ); // Postcondition: The return value is the // quadratic expression obtained by // multiplying each of q's // coefficients by the number r. Notice that the left argument of the overloaded operator* is a double number (rather than a qua- dratic expression). This allows expressions such as 3.14 * q, where q is a quadratic expression. This project is a continuation of the previous 9 project. For a quadratic expression such as ax² + bx+c, a real root is any double number .x such that ax²+bx+c = 0. For example, the quadratic expression 2x² + 8x +6 has one of its real roots at x = -3, because substituting x = -3 in the formula 2x² + 8x+6 yields the value: 2× (-3²) +8 × (-3) +6 = 0 There are six rules for finding the real roots of a qua- dratic expression: (1) If a, b, and c are all zero, then every value of x is a real root. (2) If a and bare zero, but c is nonzero, then there are no real roots. (3) If a is zero, and bis nonzero, then the only real root is x = -c/b. (4) If a is nonzero and b² <4ac, then there are no real roots. (5) If a is nonzero and b² = 4ac, then there is one real root x = -b/2a. (6) If a is nonzero, and b²2>4ac, then there are two real roots: x = -b-√√b². 2a - 4ac _b+√√b²-4ac 2a Write a new member function that returns the num- ber of real roots of a quadratic expression. This an- swer could be 0, or 1, or 2, or infinity. In the case of an infinite number of real roots, have the member function return 3. (Yes, we know that 3 is not infin- ity, but for this purpose it is close enough!) Write two other member functions that calculate and re- turn the real roots of a quadratic expression. The pre- condition for both functions is that the expression has at least one real root. If there are two real roots, then one of the functions returns the smaller of the two roots, and the other function returns the larger of the two roots. If every value of x is a real root, then both functions should return zero. 10 Specify, design, and implement a class that can be used to simulate a lunar lander, which is a small spaceship that transports astro- nauts from lunar orbit to the surface of the moon. When a lunar lander is constructed, the following items should be specified, with default values as in- dicated: (1) Current fuel flow rate as a fraction of the maximum fuel flow (default zero) (2) Vertical speed of the lander (default zero meters/sec) (3) Altitude of the lander (default 1000 meters) (4) Amount of fuel (default 1700 kg) (5) Mass of the lander when it has no fuel (de- fault 900 kg) (6) Maximum fuel consumption rate (default 10 kg/sec) (7) Maximum thrust of the lander's engine (de- fault 5000 newtons) Don't worry about other properties (such as horizon- tal speed). The lander has constant member functions that allow a program to retrieve the current values of any of these seven items. There are only two modifica- tion member functions, described next. The first modification function changes the current fuel flow rate to a new value ranging from 0.0 to 1.0. This value is expressed as a fraction of the maximum fual flom
quadratic operator +( const quadratic& q1, const quadratic& q2 ); // Postcondition: The return value is the // quadratic expression obtained by adding // q1 and q2. For example, the c coefficient // of the return value is the sum of ql's c // coefficient and q2's c coefficient. quadratic operator *( double r, const quadratic& q ); // Postcondition: The return value is the // quadratic expression obtained by // multiplying each of q's // coefficients by the number r. Notice that the left argument of the overloaded operator* is a double number (rather than a qua- dratic expression). This allows expressions such as 3.14 * q, where q is a quadratic expression. This project is a continuation of the previous 9 project. For a quadratic expression such as ax² + bx+c, a real root is any double number .x such that ax²+bx+c = 0. For example, the quadratic expression 2x² + 8x +6 has one of its real roots at x = -3, because substituting x = -3 in the formula 2x² + 8x+6 yields the value: 2× (-3²) +8 × (-3) +6 = 0 There are six rules for finding the real roots of a qua- dratic expression: (1) If a, b, and c are all zero, then every value of x is a real root. (2) If a and bare zero, but c is nonzero, then there are no real roots. (3) If a is zero, and bis nonzero, then the only real root is x = -c/b. (4) If a is nonzero and b² <4ac, then there are no real roots. (5) If a is nonzero and b² = 4ac, then there is one real root x = -b/2a. (6) If a is nonzero, and b²2>4ac, then there are two real roots: x = -b-√√b². 2a - 4ac _b+√√b²-4ac 2a Write a new member function that returns the num- ber of real roots of a quadratic expression. This an- swer could be 0, or 1, or 2, or infinity. In the case of an infinite number of real roots, have the member function return 3. (Yes, we know that 3 is not infin- ity, but for this purpose it is close enough!) Write two other member functions that calculate and re- turn the real roots of a quadratic expression. The pre- condition for both functions is that the expression has at least one real root. If there are two real roots, then one of the functions returns the smaller of the two roots, and the other function returns the larger of the two roots. If every value of x is a real root, then both functions should return zero. 10 Specify, design, and implement a class that can be used to simulate a lunar lander, which is a small spaceship that transports astro- nauts from lunar orbit to the surface of the moon. When a lunar lander is constructed, the following items should be specified, with default values as in- dicated: (1) Current fuel flow rate as a fraction of the maximum fuel flow (default zero) (2) Vertical speed of the lander (default zero meters/sec) (3) Altitude of the lander (default 1000 meters) (4) Amount of fuel (default 1700 kg) (5) Mass of the lander when it has no fuel (de- fault 900 kg) (6) Maximum fuel consumption rate (default 10 kg/sec) (7) Maximum thrust of the lander's engine (de- fault 5000 newtons) Don't worry about other properties (such as horizon- tal speed). The lander has constant member functions that allow a program to retrieve the current values of any of these seven items. There are only two modifica- tion member functions, described next. The first modification function changes the current fuel flow rate to a new value ranging from 0.0 to 1.0. This value is expressed as a fraction of the maximum fual flom
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Concept explainers
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
Question
i need this in c++ please and only 8 and 9

Transcribed Image Text:A one-variable quadratic expression
arithmetic expression
of the
ax²+bx+c, where a, b, and e are some
fixed numbers (called the coefficients) and x is a
variable that can take on different values. Specify,
design, and implement a class that can store infor-
mation about a quadratic expression. The default
constructor should set all three coefficients to zero,
and another member function should allow you to
change these coefficients. There should be constant
member functions to retrieve the current values of
the coefficients. There should also be a member
function to allow you to "evaluate" the quadratic ex-
pression at a particular value of x (i.c., the function
has one parameter x, and returns the value of the ex-
pression ax² + bx+c).
is an
form
8

Transcribed Image Text:quadratic operator +(
const quadratic& q1,
const quadratic& q2
);
// Postcondition: The return value is the
// quadratic expression obtained by adding
// q1 and q2. For example, the c coefficient
// of the return value is the sum of ql's c
// coefficient and q2's c coefficient.
quadratic operator *(
double r,
const quadratic& q
);
// Postcondition: The return value is the
// quadratic expression obtained by
// multiplying each of q's
// coefficients by the number r.
Notice that the left argument of the overloaded
operator* is a double number (rather than a qua-
dratic expression). This allows expressions such as
3.14 * q, where q is a quadratic expression.
This project is a continuation of the previous
9 project. For a quadratic expression such
as ax² + bx+c, a real root is any double
number.x such that ax²+bx+c = 0. For example,
the quadratic expression 2x² + 8x +6 has one of its
real roots at x = -3, because substituting x = -3
in the formula 2x² +8x+6 yields the value:
2x (-3²) +8x(-3) +6 = 0
There are six rules for finding the real roots of a qua-
dratic expression:
(1) If a, b, and c are all zero, then every value of
x is a real root.
(2) If a and b are zero, but c is nonzero, then there
are no real roots.
(3) If a is zero, and b is nonzero, then the only
real root is x = -c/b.
(4) If a is nonzero and b² <4ac, then there are
no real roots.
(5) If a is nonzero and b²
one real root x = -b/2a.
(6) If a is nonzero, and b²>4ac, then there are
=
4ac, then there is
two real roots:
x =
x =
2
-b-√√b²-4ac
2a
-b+√√b²-4ac
2a
Write a new member function that returns the num-
ber of real roots of a quadratic expression. This an-
swer could be 0, or 1, or 2, or infinity. In the case of
an infinite number of real roots, have the member
function return 3. (Yes, we know that 3 is not infin-
ity, but for this purpose it is close enough!) Write
two other member functions that calculate and re-
turn the real roots of a quadratic expression. The pre-
condition for both functions is that the expression
has at least one real root. If there are two real roots,
then one of the functions returns the smaller of the
two roots, and the other function returns the larger of
the two roots. If every value of x is a real root, then
both functions should return zero.
10
Specify, design, and implement a class that
can be used to simulate a lunar lander, which
is a small spaceship that transports astro-
nauts from lunar orbit to the surface of the moon.
When a lunar lander is constructed, the following
items should be specified, with default values as in-
dicated:
(1) Current fuel flow rate as a fraction of the
maximum fuel flow (default zero)
(2) Vertical speed of the lander (default zero
meters/sec)
(3) Altitude of the lander (default 1000 meters)
(4) Amount of fuel (default 1700 kg)
(5) Mass of the lander when it has no fuel (de-
fault 900 kg)
(6) Maximum fuel consumption rate (default
10 kg/sec)
(7) Maximum thrust of the lander's engine (de-
fault 5000 newtons)
Don't worry about other properties (such as horizon-
tal speed).
The lander has constant member functions that
allow a program to retrieve the current values of any
of these seven items. There are only two modifica-
tion member functions, described next.
The first modification function changes the
current fuel flow rate to a new value ranging from
0.0 to 1.0. This value is expressed as a fraction of the
maximum fuel flow.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
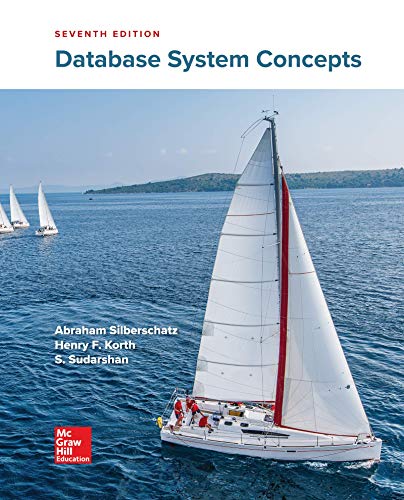
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
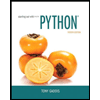
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
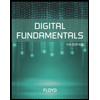
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
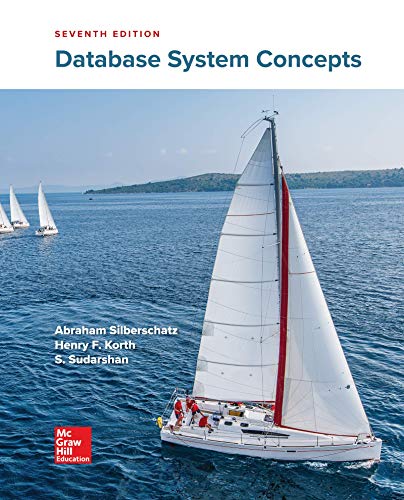
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
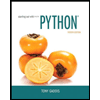
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
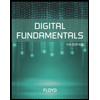
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
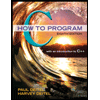
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
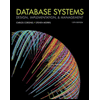
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
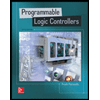
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education