(Python) Username and password creation exercies print("----------------------------------------------") print("\n\tCreate Account\n") username = input("Please enter a new username: ") """ Check if username consists of only characters from A - Z or a - z; if not, keep asking the user to enter a valid new username.""" # 1) VALIDATION CODE FOR USERNAME GOES HERE password = input("Please enter a new password: ") """ Check if password consists of only characters or digits; if not, keep asking the user to enter a valid new password.""" # 2) VALIDATION CODE FOR PASSWORD GOES HERE print("\nAccount created!\n") run = True tries_left = 3 while run is True: # while run is true, program will keep asking for sign in login_success = False login_check = input("Would you like to log in? (y/n): ") if login_check.lower() == 'y': # .lower() converts input to lowercase print("You will have 3 attempts.") '''1. For the for loop below, It should loop 3 times, once for each attempt. ''' tries_left = 3 for attempt_num in range( tries_left): # Alternatively you can change this into a while loop if you prefer to use a while structure print("\n----------------------------------------------\n") print("\tLogin\n") check_user = input("Username: ") check_pw = input("Password: ") # if login information is correct if (check_user == username and check_pw == password): print("\nLogin succeeded!") print("\n----------------------------------------------\n") login_success = True break # this break stmt, if reached, program will exit the for loop else: # if login information is incorrect print("Incorrect credentials.") tries_left -= 1 print('\nYou have', tries_left, 'attempts remaining.') if tries_left == 0: run = False break while login_success is True: # if login is successful, go to menu print("\tMenu\n") print("1. Change Username") print("2. Change Password") print("\nPress any other key to log out.") choice = input("\nEnter number of a menu option: ") print("\n----------------------------------------------\n") if choice == "1": # if user selects Change Username print("\tChange Username\n") """Ask the user to enter their username and password:""" check_user = input("Enter your current username: ") check_pw = input("Enter your current password: ") ''' COMPLETE THIS SECTION 1. Check to see if username and password are correct 2. Prompt user for a new username 3. Ask user to confirm username 4. If the new username and confirmation match, tell the user that their username is changed, print out their new username,and return to menu. If they don't match, tell the user that the usernames did not match and return to the menu NOTE: The program should handle any invalid input (wrong passwords, etc.). ''' # 3) USERNAME AND PASSWORD CHECK """ Check if username consists of only characters from A - Z or a - z; if not, keep asking the user to enter a valid new username.""" # 4) USERNAME VALIDATION print("\n----------------------------------------------\n") elif choice == "2": # if user selects Change Password print("\tChange Password\n") """Ask the user to enter their username and password:""" check_user = input("Enter your current username: ") check_pw = input("Enter your current password: ") ''' COMPLETE THIS SECTION 1. Check to see if username and password are correct 2. Prompt user for a new password 3. Ask user to confirm password 4. if the new password and confirmation match, tell the user that their password is changed and return to menu. If they don't match, tell the user that the usernames did not match and return to the menu. NOTE: The program should handle any invalid input (wrong passwords, etc.) ''' # 5) USERNAME AND PASSWORD CHECK """ Check if password consists of only characters or digits; if not, keep asking the user to enter a valid new password.""" # 6) PASSWORD VALIDATION print("\n----------------------------------------------\n") else: # This section is reached if user does not enter 1 or 2 at menu login_success = False print("Logging out...") print("\n----------------------------------------------\n") else: # this is reached when the user does not enter y or Y at login prompt run = False # This line is only reached after the user chooses to exit the program # This is outisde of the main loop input("Program exiting, press any key to continue...") quit() # This Python function terminates execution of the script
Max Function
Statistical function is of many categories. One of them is a MAX function. The MAX function returns the largest value from the list of arguments passed to it. MAX function always ignores the empty cells when performing the calculation.
Power Function
A power function is a type of single-term function. Its definition states that it is a variable containing a base value raised to a constant value acting as an exponent. This variable may also have a coefficient. For instance, the area of a circle can be given as:
(Python) Username and password creation exercies
print("----------------------------------------------")
print("\n\tCreate Account\n")
username = input("Please enter a new username: ")
""" Check if username consists of only characters from A - Z or a - z;
if not, keep asking the user to enter a valid new username."""
# 1) VALIDATION CODE FOR USERNAME GOES HERE
password = input("Please enter a new password: ")
""" Check if password consists of only characters or digits;
if not, keep asking the user to enter a valid new password."""
# 2) VALIDATION CODE FOR PASSWORD GOES HERE
print("\nAccount created!\n")
run = True
tries_left = 3
while run is True: # while run is true, program will keep asking for sign in
login_success = False
login_check = input("Would you like to log in? (y/n): ")
if login_check.lower() == 'y': # .lower() converts input to lowercase
print("You will have 3 attempts.")
'''1. For the for loop below, It should loop 3 times, once for each attempt.
'''
tries_left = 3
for attempt_num in range(
tries_left): # Alternatively you can change this into a while loop if you prefer to use a while structure
print("\n----------------------------------------------\n")
print("\tLogin\n")
check_user = input("Username: ")
check_pw = input("Password: ")
# if login information is correct
if (check_user == username and check_pw == password):
print("\nLogin succeeded!")
print("\n----------------------------------------------\n")
login_success = True
break # this break stmt, if reached, program will exit the for loop
else: # if login information is incorrect
print("Incorrect credentials.")
tries_left -= 1
print('\nYou have', tries_left, 'attempts remaining.')
if tries_left == 0:
run = False
break
while login_success is True: # if login is successful, go to menu
print("\tMenu\n")
print("1. Change Username")
print("2. Change Password")
print("\nPress any other key to log out.")
choice = input("\nEnter number of a menu option: ")
print("\n----------------------------------------------\n")
if choice == "1": # if user selects Change Username
print("\tChange Username\n")
"""Ask the user to enter their username and password:"""
check_user = input("Enter your current username: ")
check_pw = input("Enter your current password: ")
''' COMPLETE THIS SECTION
1. Check to see if username and password are correct
2. Prompt user for a new username
3. Ask user to confirm username
4. If the new username and confirmation match,
tell the user that their username is changed, print out their new
username,and return to menu. If they don't match, tell the user
that the usernames did not match and return to the menu
NOTE: The program should handle any invalid input (wrong passwords, etc.).
'''
# 3) USERNAME AND PASSWORD CHECK
""" Check if username consists of only characters from A - Z or a - z;
if not, keep asking the user to enter a valid new username."""
# 4) USERNAME VALIDATION
print("\n----------------------------------------------\n")
elif choice == "2": # if user selects Change Password
print("\tChange Password\n")
"""Ask the user to enter their username and password:"""
check_user = input("Enter your current username: ")
check_pw = input("Enter your current password: ")
''' COMPLETE THIS SECTION
1. Check to see if username and password are correct
2. Prompt user for a new password
3. Ask user to confirm password
4. if the new password and confirmation match,
tell the user that their password is changed and return to menu.
If they don't match, tell the user that the usernames did not match
and return to the menu.
NOTE: The program should handle any invalid input (wrong passwords, etc.)
'''
# 5) USERNAME AND PASSWORD CHECK
""" Check if password consists of only characters or digits;
if not, keep asking the user to enter a valid new password."""
# 6) PASSWORD VALIDATION
print("\n----------------------------------------------\n")
else: # This section is reached if user does not enter 1 or 2 at menu
login_success = False
print("Logging out...")
print("\n----------------------------------------------\n")
else: # this is reached when the user does not enter y or Y at login prompt
run = False # This line is only reached after the user chooses to exit the program
# This is outisde of the main loop
input("Program exiting, press any key to continue...")
quit() # This Python function terminates execution of the script

Step by step
Solved in 2 steps

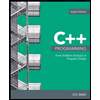
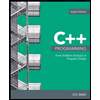