Below is the specs and my main method. (ignore add and remove method for now) Specs: Part 1 - Tweet Bot To start analyzing tweets, we first need to read in and manage the state of tweets. To do this, create a class called TweetBot. A TweetBot should have the following constructor and methods: Constructor public TweetBot(List tweets) Given a List of tweets, initialize a tweet list containing all tweets from the given collection. Note that you should not initialize your tweet list to the given List reference. You should create a new data structure and copy over all of the tweets from the given List, leaving the given List unmodified after the constructor is finished executing. Throws an IllegalArgumentException if the size of the given collection is less than 1. You may assume the given collection contains only non-empty and distinct strings. Methods public int numTweets() Returns the number of tweets currently in the tweet list. public void addTweet(String tweet) Adds the given tweet to the end of the tweet list. You may assume that the given tweet does not already exist in the TweetBot's list of tweets. Below is the main method: import java.util.*; import java.io.*; public class TwitterMain { public static void main(String[] args) throws FileNotFoundException { Scanner input = new Scanner(new File("tweets.txt")); // Make Scanner over tweet file List tweets = new ArrayList<>(); while (input.hasNextLine()) { // Add each tweet in file to List tweets.add(input.nextLine()); } TweetBot bot = new TweetBot(tweets); // Create TweetBot object with list of tweets System.out.println(bot.nextTweet()); // should print "tweet about something controversial" System.out.println(bot.nextTweet()); // should print "Remember to vote!" System.out.println(bot.nextTweet()); // should print "Look at this meme:0" System.out.println(bot.nextTweet()); // should print "tweet about something controversial" (because the index has wrapped around) bot.addTweet("This is a new tweet!"); System.out.println(bot.nextTweet()); // should print "This is a new tweet!" bot.addTweet("Hii, How are everyone?"); System.out.println(bot.nextTweet()); // should print "Hii, How are everyone?" bot.removeTweet("This is a new tweet!"); //ignore System.out.println(bot.nextTweet()); System.out.println(bot.nextTweet()); System.out.println(bot.nextTweet()); System.out.println(bot.nextTweet());
Below is the specs and my main method. (ignore add and remove method for now)
Specs:
Part 1 - Tweet Bot
To start analyzing tweets, we first need to read in and manage the state of tweets. To do this, create a class called TweetBot. A TweetBot should have the following constructor and methods:
Constructor
public TweetBot(List<String> tweets)
Given a List of tweets, initialize a tweet list containing all tweets from the given collection. Note that you should not initialize your tweet list to the given List reference. You should create a new data structure and copy over all of the tweets from the given List, leaving the given List unmodified after the constructor is finished executing.
Throws an IllegalArgumentException if the size of the given collection is less than 1.
You may assume the given collection contains only non-empty and distinct strings.
Methods
public int numTweets()
Returns the number of tweets currently in the tweet list.
public void addTweet(String tweet)
Adds the given tweet to the end of the tweet list.
You may assume that the given tweet does not already exist in the TweetBot's list of tweets.
Below is the main method:
import java.util.*;
import java.io.*;
public class TwitterMain {
public static void main(String[] args) throws FileNotFoundException {
Scanner input = new Scanner(new File("tweets.txt")); // Make Scanner over tweet file
List<String> tweets = new ArrayList<>();
while (input.hasNextLine()) { // Add each tweet in file to List
tweets.add(input.nextLine());
}
TweetBot bot = new TweetBot(tweets); // Create TweetBot object with list of tweets
System.out.println(bot.nextTweet()); // should print "tweet about something controversial"
System.out.println(bot.nextTweet()); // should print "Remember to vote!"
System.out.println(bot.nextTweet()); // should print "Look at this meme:0"
System.out.println(bot.nextTweet()); // should print "tweet about something controversial" (because the index has wrapped around)
bot.addTweet("This is a new tweet!");
System.out.println(bot.nextTweet()); // should print "This is a new tweet!"
bot.addTweet("Hii, How are everyone?");
System.out.println(bot.nextTweet()); // should print "Hii, How are everyone?"
bot.removeTweet("This is a new tweet!"); //ignore
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());
System.out.println(bot.nextTweet());

Here's a possible implementation of the TweetBot class:
CODE in JAVA:
import java.util.ArrayList;
import java.util.List;
public class TweetBot {
private List<String> tweetList;
private int currentTweetIndex;
public TweetBot(List<String> tweets) {
if (tweets.size() < 1) {
throw new IllegalArgumentException("The list of tweets must contain at least one tweet.");
}
this.tweetList = new ArrayList<>(tweets);
this.currentTweetIndex = 0;
}
public int numTweets() {
return tweetList.size();
}
public void addTweet(String tweet) {
tweetList.add(tweet);
}
public void removeTweet(String tweet) {
tweetList.remove(tweet);
}
public String nextTweet() {
String tweet = tweetList.get(currentTweetIndex);
currentTweetIndex = (currentTweetIndex + 1) % tweetList.size(); // wrap around index
return tweet;
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Thank you! but for nextTweet and removeTweet. can u rewrite base on the specs:
public String nextTweet()
Returns the next tweet from the tweet list. If all the tweets in the list are exhausted, cycle around to the start of the list.
For example, the following code snippet should produce the output in the comments. Suppose that tweets stores a list of Tweets ["A tweet about something controversial", "Remember to vote!", "Look at this meme :O"].
Think about how to get the next tweet from the list of tweets, and how you can cycle around to the start once you've gone through all the tweets!
One Special Case!
As you're working on your TweetBot and testing your implementation, you'll likely start thinking about different cases that your TweetBot will need to handle. There is one specific special case whose behavior is particularly tricky, so we wanted to talk about it more in depth.
Consider the case where your TweetBot is storing a single tweet (referred to as tweet1), and you call nextTweet() on your TweetBot. Then, you immediately afterwards add in another tweet (referred to as tweet2). On the next call to nextTweet(), your TweetBot should return tweet2 (the tweet that was just added in) -- NOT tweet1.
Note that the case described here is a tricky one - really there are couple of different ways that a TweetBot could reasonably handle this situation. But, since you'll be writing code to pass tests that we provide, we're telling you how you should handle this situation for this assignment!
public void removeTweet(String tweet)
Removes the given tweet from the tweet list if it is present.
Pay particular attention to making sure that the remove method should preserve the current iteration order of the nextTweet method above. In particular, using the example tweets from the last method, the following code should produce the output shown in the comments.
In other words, the nextTweet method should continue the iteration through the Tweet list, returning the Tweet immediately following the one that was last returned by nextTweet, continuing with only the removed tweet cut out of the cycle.
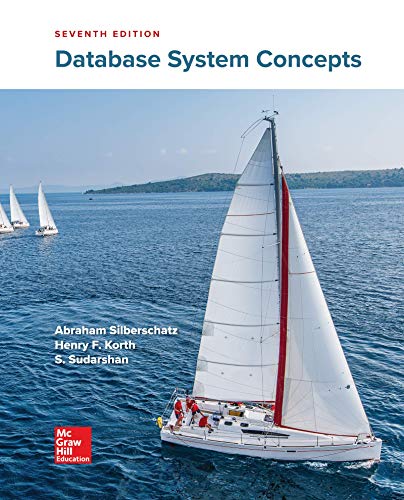
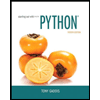
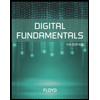
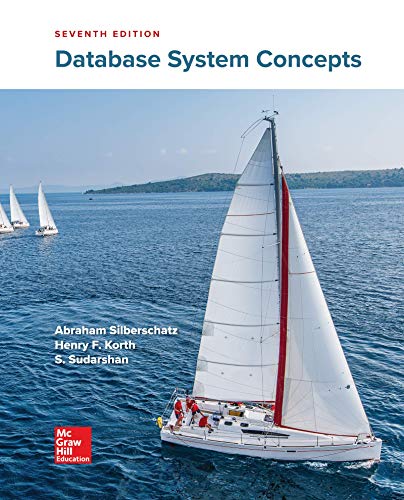
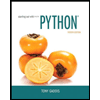
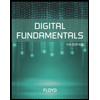
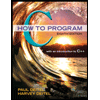
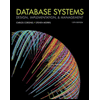
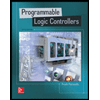