public static void connectToInputFile() { Scanner inputStream = null; String inputFileName = getFileName("Enter name of input file: "); try { inputStream = new Scanner(new File("SortedA.txt")); } catch(FileNotFoundException e) { System.out.println("File " + inputFileName + " not found. "); } catch(IOException e) { System.out.println("Error opening input file: " + inputFileName); } } private static String getFileName(String prompt) { String fileName = null; System.out.println(prompt); Scanner keyboard = new Scanner (System.in); fileName = keyboard.next(); return fileName; } public static void connectToOutputFile() { String outputFileName = getFileName("Enter name of output file: "); try { outputStream = new ObjectOutputStream(new FileOutputStream("SortedArray.csv")); } catch(IOException e) { System.out.println("Error opening output file" + outputFileName); System.out.println(e.getMessage()); } } public static void closeFiles() { try { inputStream.close(); outputStream.close(); } catch(IOException e) { System.out.println("Error closing files " + e.getMessage()); } } Hi, I am planning to use the SortedA.txt file to populate the SortedArray.csv file with the data inside the text file. But for now, it only manages to create a csv file but without any data inside. How can I modify the code above to let the csv file be populated with the data from the txt file?
public static void connectToInputFile()
{
Scanner inputStream = null;
String inputFileName = getFileName("Enter name of input file: ");
try
{
inputStream = new Scanner(new File("SortedA.txt"));
}
catch(FileNotFoundException e)
{
System.out.println("File " + inputFileName + " not found. ");
}
catch(IOException e)
{
System.out.println("Error opening input file: " + inputFileName);
}
}
private static String getFileName(String prompt)
{
String fileName = null;
System.out.println(prompt);
Scanner keyboard = new Scanner (System.in);
fileName = keyboard.next();
return fileName;
}
public static void connectToOutputFile()
{
String outputFileName = getFileName("Enter name of output file: ");
try
{
outputStream = new ObjectOutputStream(new FileOutputStream("SortedArray.csv"));
}
catch(IOException e)
{
System.out.println("Error opening output file" + outputFileName);
System.out.println(e.getMessage());
}
}
public static void closeFiles()
{
try
{
inputStream.close();
outputStream.close();
}
catch(IOException e)
{
System.out.println("Error closing files " + e.getMessage());
}
}
Hi, I am planning to use the SortedA.txt file to populate the SortedArray.csv file with the data inside the text file. But for now, it only manages to create a csv file but without any data inside. How can I modify the code above to let the csv file be populated with the data from the txt file?

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

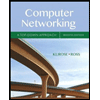
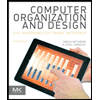
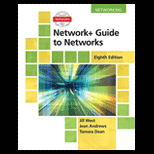
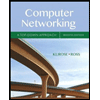
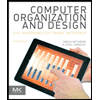
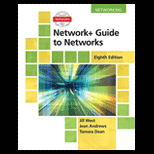
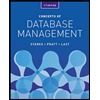
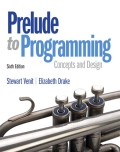
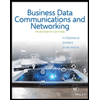