Pseudocode is a useful tool for mapping out complex algorithms in a language-independent way before even writing one line of code. GoogleStepwise Refinement and Divide-and-Conquer. We’re going to practice this approach in detail here. We’ll start with a very high level description of the program, and flesh out bits of detail in each stepwise refinement. Given the small amount of pseudocode below, can you take a small, incremental step to describe in more detail the steps required to accomplish this task? Pseudocode Refinement Step 1–The Problem Statement This should be as short as possible, yet convey the full requirement of the program (however vague). Consider the following starting point for your stepwise refinement, which was pulled from the homework description. “Given a target file to find and a starting directory, determine if and where the target file exists.” Pseudocode Refinement Step 2 Using the above sentence as an incomplete guide split the embedded concepts into 4 distinct steps, such as: Setup & Initialization (for the program, or a specific function, similar to preconditions) 2. Input 3. Processing 4. Output Pseudocode Refinement Step 3 to (N-1) Using Step 2 above as a slightly more complete guide, split up the processing phase into more discrete steps required to searcha directory. For example, a start on the next step might be... //just for the (3) step above (Processing)) while(more directories to look at) { look at one file or directory and check for a match and return it if found if the current item is a directory, we must repeat these steps for this directory as well } target not found at this point (Spoiler Alert!)Stepwise Refinement, Step N Compare your final stepwise refinement to the refinement below of the processing step we examined above. Does the logic appear similar? Submit your stepwise refinement as a separate file in your homework. Add the given directory to some structure to manage directories while(more directories to examine) { Get a directory If a file, check for match If a directory, for(each file and directory in the directory) { if a file, check for a match and return if found if a directory, save this in a structure } } return not found 7.2 From Pseudocode to Java -File Search, Version 1.0 Build a linear search following the pseudocode approach above. You can put this code in simply a main, or you could design a static helper function in your HP static class that searches for files. Did you notice we used a stack to accomplish this directory searching? Using the main below and the outline above, complete the iterative file searching algorithm in the method searchFiles(). Use fileObject.listFiles(), fileObject.isDirectory(), and fileObject.getName() to accomplish this below. public static void main(String[] args) { System.out.println( searchFiles(new File("c:\\"), "hw3.zip") ); } public static String searchFiles(File path, String target) { //todo } 7.3 File Search, Version 2.0 We made use of an explicit stack to track new directories as they appear in the above iterative code. Do you think we could use the method call stack in place of our Java stack to accomplish this recursively? If we remove the stack definition and code above and produce a recursive function, might that be shorter? Change the main function above to call recursiveSearch() instead, which is a new method that you will create. This method will take the same input as searchFiles, but instead of searching through files using explicit for loops and a Stack from java.util, we’ll be using recursive function calls to facilitate some of the looping and use the method call stack to store our directory list instead. Build a recursive linear search following the pseudocode you have refined to this point, or using the starter pseudocode approach below. Note that multiple distinct correct solutions exist to this problem, so don’t worry if your pseudocode isn’t exactly like the code below. public static String searchFiles(File path, String target) { check if path is actually a dir, abort if not loop over all files & dirs in the current directory if a file, check for a match and return if found if a directory, repeat these steps if found in the directory, return found return “Not found”; //if we made it here, we didn’t find it }
Pseudocode is a useful tool for mapping out complex algorithms in a language-independent way before even writing one line of code. GoogleStepwise Refinement and Divide-and-Conquer. We’re going to practice this approach in detail here. We’ll start with a very high level description of the program, and flesh out bits of detail in each stepwise refinement. Given the small amount of pseudocode below, can you take a small, incremental step to describe in more detail the steps required to accomplish this task?
Pseudocode Refinement Step 1–The Problem Statement
This should be as short as possible, yet convey the full requirement of the program (however vague). Consider the following starting point for your stepwise refinement, which was pulled from the homework description.
“Given a target file to find and a starting directory, determine if and where the target file exists.”
Pseudocode Refinement Step 2
Using the above sentence as an incomplete guide split the embedded concepts into 4 distinct steps, such as:
- Setup & Initialization (for the program, or a specific function, similar to preconditions)
2. Input
3. Processing
4. Output
Pseudocode Refinement Step 3 to (N-1)
Using Step 2 above as a slightly more complete guide, split up the processing phase into more discrete steps required to searcha directory. For example, a start on the next step might be...
//just for the (3) step above (Processing))
while(more directories to look at) {
look at one file or directory and check for a match and return it if found
if the current item is a directory, we must repeat these steps for this directory as well
}
target not found at this point
(Spoiler Alert!)Stepwise Refinement, Step N
Compare your final stepwise refinement to the refinement below of the processing step we examined above. Does the logic appear similar? Submit your stepwise refinement as a separate file in your homework.
7.2 From Pseudocode to Java -File Search, Version 1.0
Build a linear search following the pseudocode approach above. You can put this code in simply a main, or you could design a static helper function in your HP static class that searches for files. Did you notice we used a stack to accomplish this directory searching? Using the main below and the outline above, complete the iterative file searching
7.3 File Search, Version 2.0
We made use of an explicit stack to track new directories as they appear in the above iterative code. Do you think we could use the method call stack in place of our Java stack to accomplish this recursively? If we remove the stack definition and code above and produce a recursive function, might that be shorter? Change the main function above to call recursiveSearch() instead, which is a new method that you will create. This method will take the same input as searchFiles, but instead of searching through files using explicit for loops and a Stack from java.util, we’ll be using recursive function calls to facilitate some of the looping and use the method call stack to store our directory list instead. Build a recursive linear search following the pseudocode you have refined to this point, or using the starter pseudocode approach below. Note that multiple distinct correct solutions exist to this problem, so don’t worry if your pseudocode isn’t exactly like the code below.

Hello, I have implemented the required file searching method, which will recursively search for a file starting from a given path, satisfying all the requirements in the question. I have written enough comments in every statements to let you understand how things work. Thanks.
// FileSearch.java
import java.io.File;
public class FileSearch {
public static void main(String[] args) {
System.out.println("File is being searched, please wait...");
// replace with your directory name and target file
System.out
.println(searchFiles(new File("D:\\work"), "marshmallow.txt"));
}
/**
* method to search for a file recursively
*
* @param path
* - starting directory
* @param target
* - target file name
* @return - 'Not a valid directory' if the path is invalid, 'Not found' if
* the file is not found, else will display the path in which the
* file is found
*/
public static String searchFiles(File path, String target) {
if (!path.isDirectory()) {
/**
* path is not a valid directory
*/
return "Not a valid directory";
} else {
/**
* listing all files in the current path
*/
File files[] = path.listFiles();
/**
* checking if the array is null. if the array is null, it means
* that there are no files in the path
*/
if (files != null) {
/**
* looping through all files
*/
for (File f : files) {
if (f.getName().equalsIgnoreCase(target)) {
/**
* found
*/
return "File " + target
+ " is found in the directory: " + path;
} else if (f.isDirectory()) {
/**
* it is a directory, so we perform an inner search and
* store the result in a variable
*/
String innerSearch = searchFiles(f, target);
/**
* if the result doesn't return 'Not found' then the
* file is found, so we will return the result (or else
* it will move on to the next file in the loop)
*/
if (!innerSearch.equalsIgnoreCase("Not found")) {
return innerSearch;
}
}
}
}
}
/**
* if the control reach here, it means the file is not found in the
* current path, so returning not found message
*/
return "Not found";
}
}
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

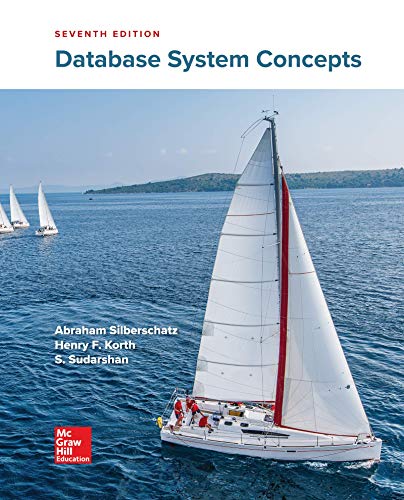
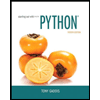
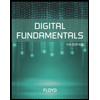
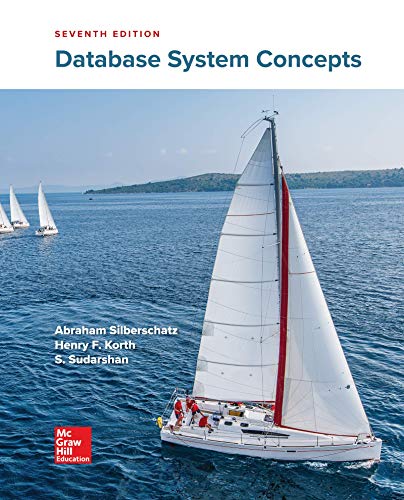
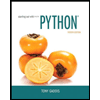
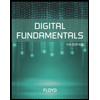
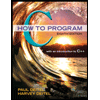
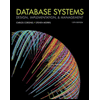
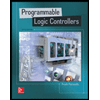