Problem: Encode the file by adding 5 to every byte in the file. Write a program that prompts the useer to enter an input file name and an output file name and saves the encrypted version of the input file to the output file. I don't get any encrypted file. Why? package encrypt; import java.io.*; import java.util.*; public class Encrypt { public static void main(String[] args){ // Input from user Scanner input = new Scanner(System.in); System.out.print("Enter a file to encrupt:"); // Input file from user File inputfile = new File(input.nextLine()); System.out.print("Enter the output file:"); // Output file from user File outputfile = new File(input.nextLine()); try { // Create BufferedInputStream from bis BufferedInputStream bis = new BufferedInputStream (new FileInputStream(inputfile)); // Create BufferedOutputStream from bos BufferedOutputStream bos = new BufferedOutputStream (new FileOutputStream(outputfile)); // Declare the variable num int num; // Checks content in the variable num is not equal to -1 while ((num=bis.read())!=-1) { // Call the function Write() bos.write(num+5); } } catch (IOException e) { } } }
Problem: Encode the file by adding 5 to every byte in the file. Write a program that prompts the useer to enter an input file name and an output file name and saves the encrypted version of the input file to the output file. I don't get any encrypted file. Why?
package encrypt;
import java.io.*;
import java.util.*;
public class Encrypt {
public static void main(String[] args){
// Input from user
Scanner input = new Scanner(System.in);
System.out.print("Enter a file to encrupt:");
// Input file from user
File inputfile = new File(input.nextLine());
System.out.print("Enter the output file:");
// Output file from user
File outputfile = new File(input.nextLine());
try {
// Create BufferedInputStream from bis
BufferedInputStream bis = new BufferedInputStream (new FileInputStream(inputfile));
// Create BufferedOutputStream from bos
BufferedOutputStream bos = new BufferedOutputStream (new FileOutputStream(outputfile));
// Declare the variable num
int num;
// Checks content in the variable num is not equal to -1
while ((num=bis.read())!=-1)
{
// Call the function Write()
bos.write(num+5);
}
} catch (IOException e) {
}
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

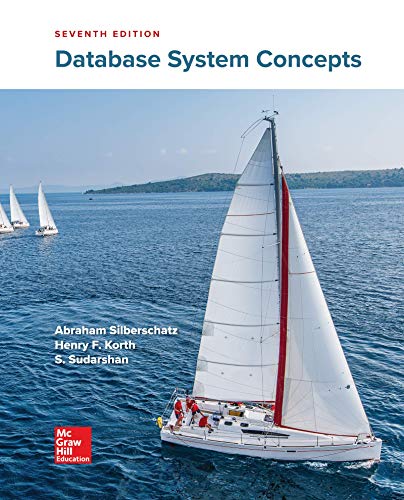
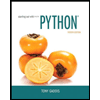
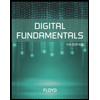
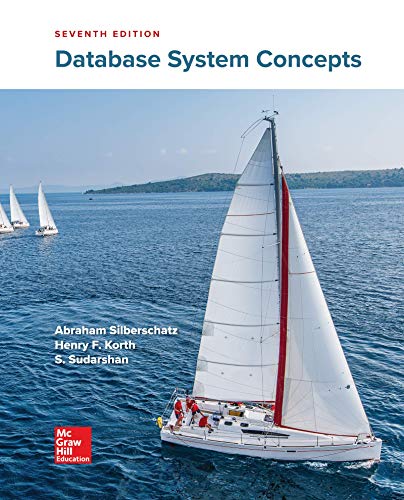
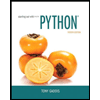
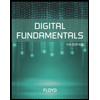
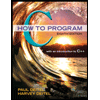
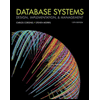
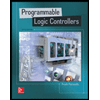