Build a program that... Ask user for day of month (1 - 31) get value and save (add to month and year to this value) Ask user for month (1 - 12) get value and save Ask user for year (1980 - 2107) 2^7 = 128; 1980 + 128 = 2108 get value; subtract 1980(base), and save
**This is a Assembly Language x86 Processors.
- The file needs to be in .asm file
- The code should not be too advance.
- Please submit the screenshot of the program and to text copy.
Build a program that...
- Ask user for day of month (1 - 31)
- get value and save (add to month and year to this value)
- Ask user for month (1 - 12)
- get value and save
- Ask user for year (1980 - 2107) 2^7 = 128; 1980 + 128 = 2108
- get value; subtract 1980(base), and save
This is the part of the code.
; Day: 1-31 (5 bits)
; Month: 1-12 (4 bits)
; Year: 25-31 (7 bits)
; mov ax, day (day of year)
; shift left ax, 5b bits 0000000DDDD00000
; add ax, month 0000000DDDDMMMM
; shift ax by 7 bits (left) DDDDMMMMM0000000
add year to ax DDDDMMMMMYYYYYYY
;
; date
mov eax, edx
and ax, 011111b ;
call WriteDec
; month
mov eax, edx
shr ax, 5
and ax, 01111b
call WriteDec
; year
mov eax, edx
sar eax, 9
add eax, 1980 calle WriteDec
; printout value as bit string (call WriteBin)
;
; Now Verify (UNPACK AND PRINT)
; pull out Day and print
; pull out month and print
; pull out Year and print
; | DH | DL
; DDDDMMMM MYYYYYYY
;
; Bit index # Num of Bits
; Year: 6-0 7
; Month: 11-7 4
; Day 15-12 5
This is my program, its a working program, and it only prints the first output:
INCLUDE Irvine32.inc
INCLUDE Macros.inc
.data
year BYTE 0
month BYTE 0
day BYTE 0
.code
main PROC
mwrite <"enter the day (of month) as 1-31: " >
call ReadDec
mov day, al
mwrite <"enter the month (1-12): " >
call ReadDec
mov month, al
mwrite <"enter year: " >
call readDec
sub ax, 1980
mov year, al
movzx ax, day ;ax: 0000 0000 0000 0000
shl ax, 4 ;ax: 0000 00d ddd0 0000
add al, month ; 0000 0000 dddm mmmm
shl ax, 7 ; dddd dmmm m000 0000
add al, year ; dddd mmmm myyy yyyy
mov ebx, 2
call WriteBinb
exit
main ENDP
END main
first Output:
This is the user input:
Enter the day (of month) as 1 - 31: 28
Enter the month (1 - 12): 4
Enter year: 1981
1110 0010 0000 0001
The program output: (This is the final output I want and with the first output needs to show)
day: 11100 16+8+4 = 28
month: 0100 4 = 4
year: 0000001 1 = 1981

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

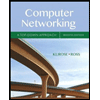
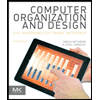
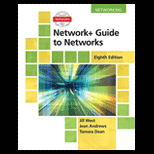
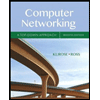
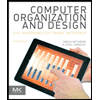
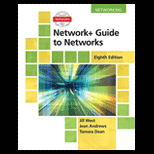
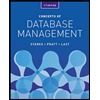
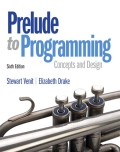
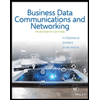